Shaving Bytes: Optimize Your Groovy Web App Template!
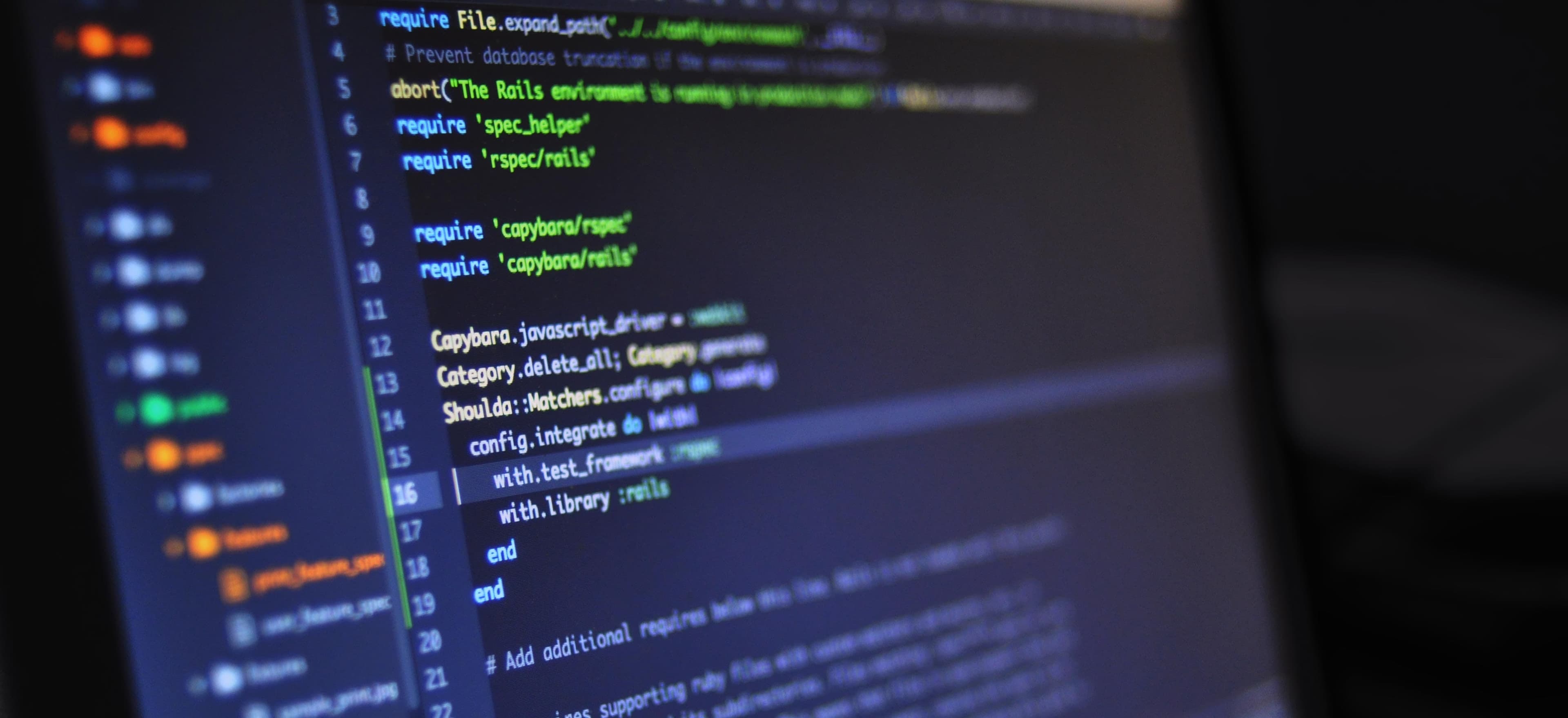
- Published on
Shaving Bytes: Optimize Your Groovy Web App Template
In the world of web development, every byte counts. With the increasing demand for fast-loading web pages and responsive applications, it's crucial to optimize our code for efficiency. This not only improves user experience but also positively impacts search engine rankings. In this blog post, we'll explore ways to optimize a Groovy web app template for improved performance.
Understanding the Importance of Optimization
Before we delve into optimization techniques, let's first understand why it's crucial. Optimizing a web app template has a direct impact on the user experience. Faster page load times lead to lower bounce rates and higher user engagement. Additionally, optimized code is favored by search engines, thus contributing to better SEO rankings.
Analyzing the Initial Template
Before we start optimization, let's take a look at a snippet of our initial Groovy web app template:
// HomeController.groovy
class HomeController {
def index() {
render 'Welcome to the Groovy Web App!'
}
def about() {
render 'This is a Groovy-powered web application.'
}
}
The above code represents a simple HomeController in a Groovy web app. While the code is functional, there are opportunities for optimization, especially as the application scales.
Minimizing Dependencies
One of the key areas for optimization is minimizing dependencies. Unnecessary dependencies can bloat the application and impact performance. Let's consider the addition of a logging library in our Groovy web app:
@Grab('org.slf4j:slf4j-simple:1.7.30')
import org.slf4j.Logger
import org.slf4j.LoggerFactory
class HomeController {
def logger = LoggerFactory.getLogger(this.class)
def index() {
logger.info('Rendering index page')
render 'Welcome to the Groovy Web App!'
}
def about() {
logger.info('Rendering about page')
render 'This is a Groovy-powered web application.'
}
}
In this code, we've introduced the SLF4J logging library. While logging is essential for debugging and monitoring, it's crucial to evaluate the necessity of each dependency. In this case, if logging is not extensively utilized, consider removing the dependency to improve the app's performance.
Utilizing Static Resources
Another effective optimization technique is leveraging static resources. By offloading static content such as CSS, JavaScript, and images to a web server or Content Delivery Network (CDN), we can reduce the load on the application server and improve load times for users.
In a Grails web application, we can utilize the asset-pipeline
plugin to manage and optimize static resources effectively. Here's an example of how we can integrate static resources using the plugin:
// application.groovy
plugins {
id 'asset-pipeline' version '3.0.17'
}
// ApplicationResources.groovy
modules = {
application {
dependsOn 'jquery'
resource url: '/css/app.css'
resource url: '/js/app.js'
}
}
By offloading static resources and utilizing the asset-pipeline plugin, we can significantly improve the performance of our Groovy web application.
Implementing Caching Strategies
Caching is a powerful technique for reducing load times and optimizing web applications. In a Groovy web app, we can implement caching at various levels, including data caching, template caching, and HTTP caching.
// HomeController.groovy
class HomeController {
def grailsApplication
def index() {
def cachedContent = grailsApplication.getCache().get('indexPageContent')
if (cachedContent) {
render cachedContent
} else {
def content = 'Welcome to the Groovy Web App!'
grailsApplication.getCache().put('indexPageContent', content)
render content
}
}
}
In this example, we've implemented simple page content caching using Grails' built-in caching capabilities. By caching frequently accessed content, we can reduce the load on the server and improve response times for users.
Lessons Learned
Optimizing a Groovy web app template is essential for delivering a fast and efficient user experience. By minimizing dependencies, leveraging static resources, and implementing caching strategies, we can significantly improve the performance of our web application. Additionally, tools like the asset-pipeline
plugin in Grails provide powerful features for managing static resources effectively.
In conclusion, optimizing a Groovy web app template not only enhances user experience but also plays a crucial role in achieving better SEO rankings and overall site performance.
By incorporating these optimization techniques, we can ensure that every byte in our Groovy web app counts, resulting in a blazing-fast and responsive application.
Remember, optimizing for performance is an ongoing process, so continuously monitor, test, and refine your web app to ensure it remains finely tuned for optimal performance.
For further reading on Groovy and web application optimization, I recommend checking out the official Grails documentation and exploring best practices for web performance optimization.
Happy optimizing!