Mastery of Microservices: Decoding Design Principles
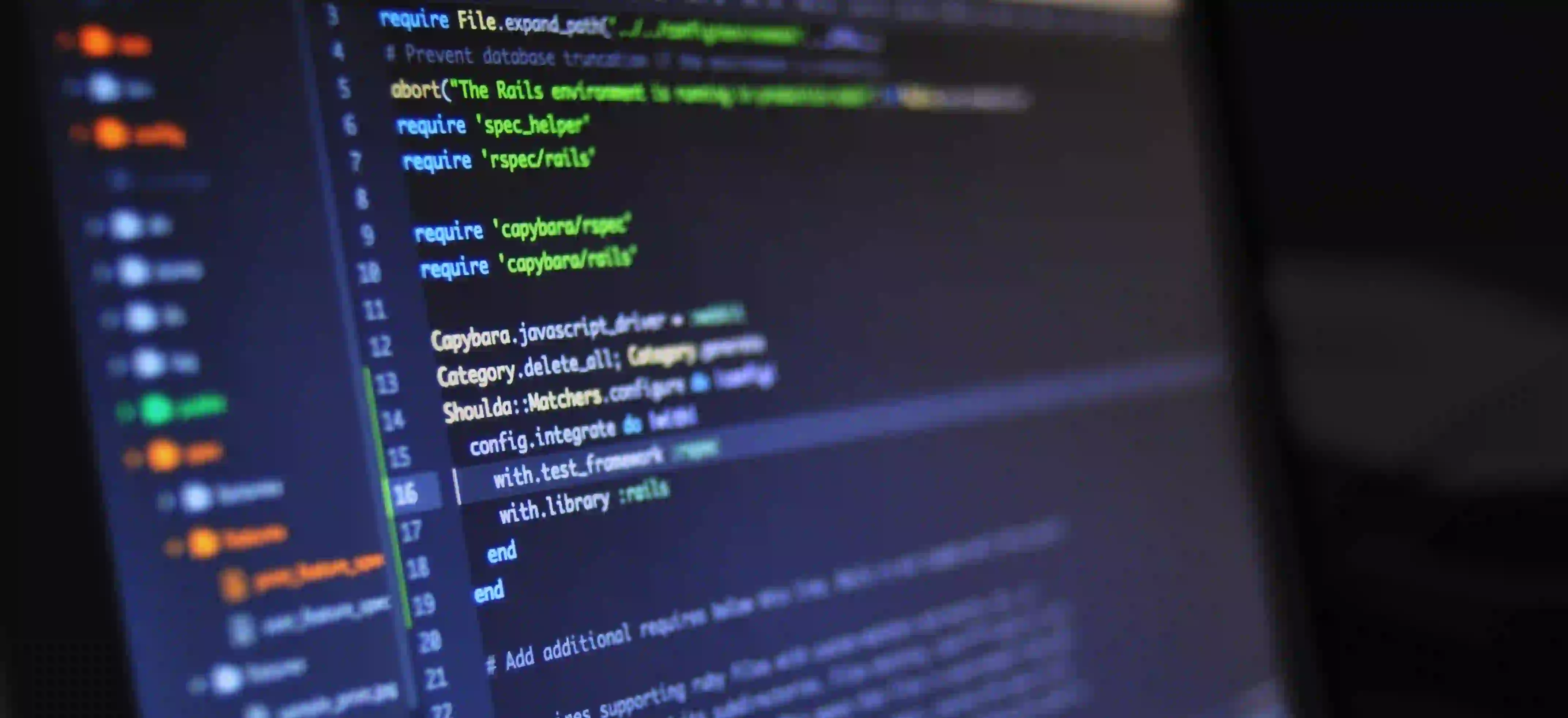
Mastery of Microservices: Decoding Design Principles
Microservices architecture has gained significant traction in recent years due to its ability to enable continuous delivery and deployment of large, complex applications. However, designing and implementing a microservices architecture comes with its own set of challenges. In this blog post, we will delve into the essential design principles that are crucial for mastering microservices architecture.
Understanding Microservices Architecture
Microservices architecture is a design approach in which a single application is composed of small, independent services that are built around specific business capabilities. Each microservice runs its own process and communicates with other services via well-defined APIs. This architecture enables teams to work independently, deploy more frequently, and scale different services based on varying demands.
Design Principles for Mastery
1. Decompose by Business Capability
When designing a microservices architecture, it is essential to decompose the application into smaller services based on business capabilities rather than technical layers. This approach ensures that each service is aligned with a specific business function, making the application more modular and easier to understand.
public class OrderService {
// Methods for handling orders
}
public class PaymentService {
// Methods for processing payments
}
2. Embrace Decentralization
Microservices should be designed to operate independently, with each service owning its data and being responsible for its own persistence. This decentralized approach allows for greater flexibility and scalability, as well as reducing the dependencies between services.
3. Resilience through Isolation
Since microservices interact with each other over the network, failures are inevitable. Designing for resilience involves isolating failure and preventing it from cascading across the system. This can be achieved through techniques such as circuit breakers, bulkheads, and timeouts.
4. Autonomous Deployment
Each microservice should be independently deployable without requiring changes to other services. This enables teams to release new features and fixes more rapidly, leading to faster innovation and a more responsive application.
5. Stateless Services
Microservices should strive to be stateless, meaning that each request contains all the information necessary to fulfill it. This design principle simplifies scaling, as requests can be distributed to any instance of a service without concerns about session affinity.
public class ShoppingCartService {
public void addItemToCart(String userId, String itemId) {
// Process the request without relying on previous state
}
}
6. Event-Driven Collaboration
Utilizing an event-driven architecture facilitates loose coupling between services, allowing them to communicate asynchronously. Events can be used to trigger actions in other services, leading to greater flexibility and scalability.
Best Practices for Implementation
In addition to understanding the design principles, adopting best practices for implementing microservices is crucial for successful outcomes. Here are some best practices to consider:
1. Containerization with Docker
Utilize containerization with Docker to package each microservice along with its dependencies, enabling consistent deployment across different environments. This practice ensures that the service runs consistently regardless of the deployment environment.
2. API Gateway for External Access
Implement an API gateway to provide a single entry point for external clients to access the microservices. This gateway can handle authentication, monitoring, and routing of requests to the appropriate services, simplifying the external interface.
3. Continuous Integration and Deployment (CI/CD)
Establish a robust CI/CD pipeline to automate the build, testing, and deployment of microservices. This practice reduces the time and effort required to deliver changes to production, increasing the overall agility of the development process.
4. Monitoring and Observability
Implement comprehensive monitoring and observability tools to gain insights into the performance and behavior of microservices. This includes logging, tracing, and metrics collection to facilitate troubleshooting and performance optimization.
5. Service Mesh for Communication
Consider implementing a service mesh, such as Istio or Linkerd, to manage the communication between microservices. This approach provides features like traffic control, security, and resilience, easing the complexities of microservice communication.
Final Thoughts
Mastering microservices architecture requires a deep understanding of the foundational design principles and best practices for implementation. By embracing the principles of decomposition, decentralization, resilience, autonomy, statelessness, and event-driven collaboration, and leveraging best practices such as containerization, API gateways, CI/CD, monitoring, and service mesh, organizations can effectively design and implement scalable, resilient, and agile microservices architectures.
In conclusion, as microservices continue to be a dominant architectural style for building cloud-native applications, mastering the design principles and best practices is essential for architecting and deploying successful microservices-based solutions.
To delve deeper into the world of microservices, check out The 12 Factor App, a methodology for building modern, scalable, and maintainable software-as-a-service applications.
Remember, the mastery of microservices lies not only in understanding the theory but also in the skillful application of these principles in real-world scenarios.