Mastering Field Validation in Your Spring Boot App
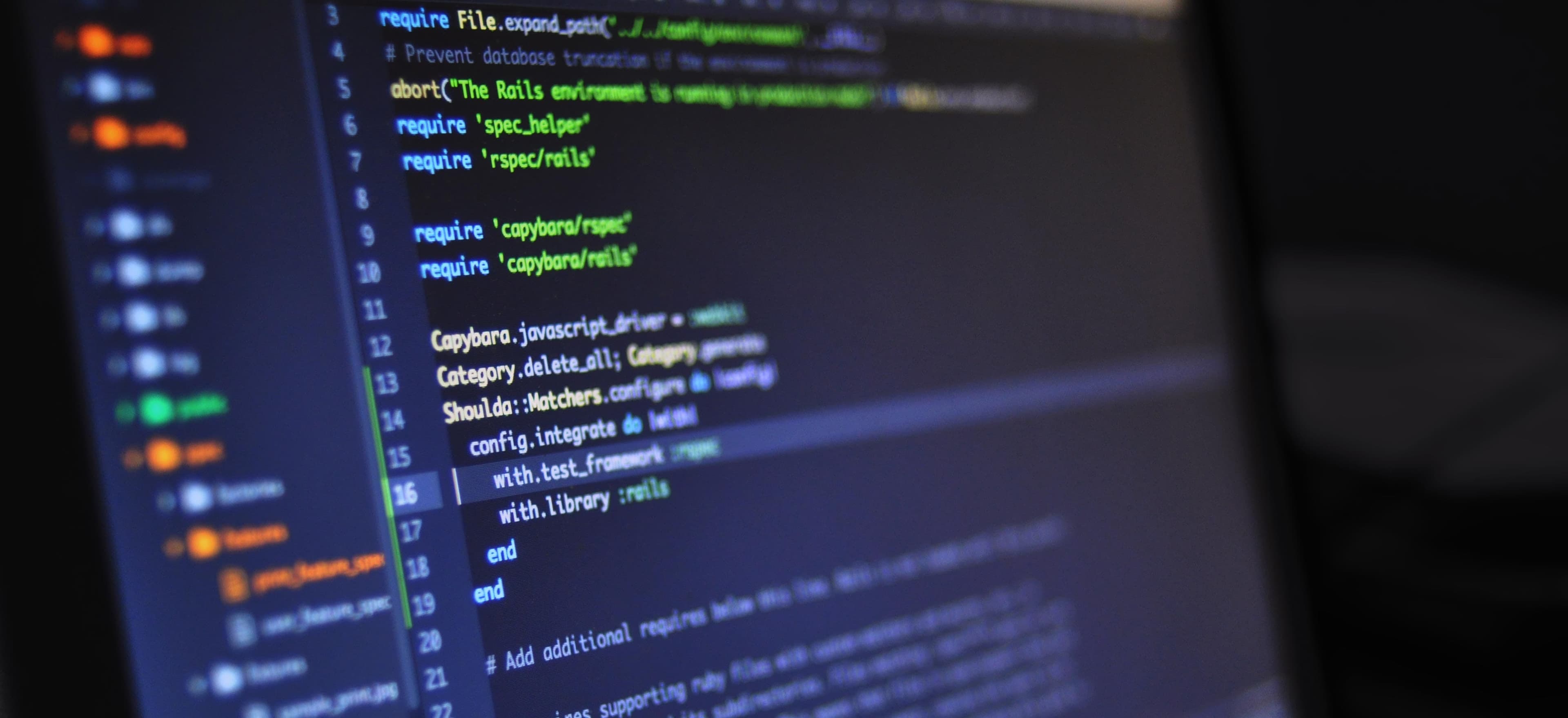
- Published on
Mastering Field Validation in Your Spring Boot App
Field validation is a crucial aspect of any web application, ensuring that the data entered by users meets the required criteria. In a Spring Boot application, you can implement field validation using various techniques provided by the Spring framework. In this blog post, we'll explore different approaches to field validation in a Spring Boot application and learn how to effectively apply them to create robust and secure web applications.
Why Field Validation is Important
Field validation is essential for maintaining data integrity, ensuring that the data stored in the application's database is accurate and consistent. It also enhances the user experience by providing immediate feedback on the correctness of the data they input, thereby preventing unnecessary form submissions and reducing errors.
In a Spring Boot application, field validation can be applied at different levels, including the frontend for a better user experience and the backend to enforce business rules and security requirements.
Using Annotations for Field Validation
One of the most common approaches for field validation in Spring Boot is using annotations provided by the javax.validation
package. These annotations can be applied to the fields of your data transfer objects (DTOs) or model classes to define validation constraints.
Let's consider a simple DTO representing a user registration form:
public class UserRegistrationDto {
@NotBlank(message = "Username is required")
private String username;
@Email(message = "Invalid email address")
private String email;
@Size(min = 6, message = "Password must be at least 6 characters")
private String password;
// Getters and setters
}
In the above example, we've utilized annotations such as @NotBlank
, @Email
, and @Size
to specify validation constraints for the username
, email
, and password
fields, respectively.
When a request containing this DTO is processed by a Spring MVC controller, the validation constraints are automatically enforced, and any validation errors are reported back to the client.
Handling Validation Errors
To handle validation errors in a Spring MVC controller, you can use the @Valid
annotation to trigger the validation process for a given DTO. Additionally, you can utilize the BindingResult
object to inspect and process the validation results.
@RestController
@RequestMapping("/users")
public class UserController {
@PostMapping("/register")
public ResponseEntity<String> registerUser(@Valid @RequestBody UserRegistrationDto userDto, BindingResult result) {
if (result.hasErrors()) {
// Handle validation errors
return ResponseEntity.badRequest().body("Invalid user registration data");
}
// Process user registration
return ResponseEntity.ok("User registered successfully");
}
}
In the above example, the @Valid
annotation triggers the validation process for the userDto
, and any validation errors are captured in the result
object. If validation errors are present, an appropriate response can be sent back to the client.
Customizing Validation Error Responses
Spring Boot provides the flexibility to customize validation error responses using the MethodArgumentNotValidException
and ResponseEntityExceptionHandler
.
@ControllerAdvice
public class GlobalExceptionHandler extends ResponseEntityExceptionHandler {
@Override
protected ResponseEntity<Object> handleMethodArgumentNotValid(MethodArgumentNotValidException ex, HttpHeaders headers, HttpStatus status, WebRequest request) {
Map<String, Object> body = new LinkedHashMap<>();
body.put("timestamp", LocalDateTime.now());
body.put("status", status.value());
// Get all validation errors
List<String> errors = ex.getBindingResult().getFieldErrors().stream()
.map(DefaultMessageSourceResolvable::getDefaultMessage)
.collect(Collectors.toList());
body.put("errors", errors);
return new ResponseEntity<>(body, headers, status);
}
}
In the above example, we've created a global exception handler to customize the response for MethodArgumentNotValidException
. This allows us to construct a custom error response including the timestamp, status, and a list of validation errors.
Programmatic Validation using Validator
In addition to annotation-based validation, Spring Boot also supports programmatic validation using the Validator
interface. This approach is beneficial when custom validation logic needs to be applied or when validation constraints are dynamic.
Let's consider an example where we need to apply conditional validation based on the value of a field:
public class PurchaseOrderDto {
private boolean isImmediateDelivery;
private LocalDate deliveryDate;
// Getters and setters
}
To validate the deliveryDate
based on the value of isImmediateDelivery
, we can create a custom validator:
public class PurchaseOrderValidator implements Validator {
@Override
public boolean supports(Class<?> clazz) {
return PurchaseOrderDto.class.isAssignableFrom(clazz);
}
@Override
public void validate(Object target, Errors errors) {
PurchaseOrderDto purchaseOrder = (PurchaseOrderDto) target;
if (purchaseOrder.isImmediateDelivery() && purchaseOrder.getDeliveryDate() != null) {
errors.rejectValue("deliveryDate", "Immediate delivery must not have a specified delivery date");
} else if (!purchaseOrder.isImmediateDelivery() && purchaseOrder.getDeliveryDate() == null) {
errors.rejectValue("deliveryDate", "Delivery date is required");
}
}
}
The custom validator PurchaseOrderValidator
performs conditional validation based on the isImmediateDelivery
field, rejecting the deliveryDate
with appropriate error messages when necessary.
Integrating Programmatic Validation
To integrate the PurchaseOrderValidator
with a Spring MVC controller, we can use the @InitBinder
annotation:
@RestController
@RequestMapping("/orders")
public class OrderController {
@Autowired
private PurchaseOrderValidator purchaseOrderValidator;
@InitBinder
protected void initBinder(WebDataBinder binder) {
binder.addValidators(purchaseOrderValidator);
}
@PostMapping("/create")
public ResponseEntity<String> createOrder(@Valid @RequestBody PurchaseOrderDto orderDto, BindingResult result) {
if (result.hasErrors()) {
// Handle validation errors
return ResponseEntity.badRequest().body("Invalid order data");
}
// Process order creation
return ResponseEntity.ok("Order created successfully");
}
}
In the above example, we've integrated the PurchaseOrderValidator
using the @InitBinder
annotation to provide programmatic validation for the orderDto
.
Lessons Learned
Field validation is a fundamental aspect of building reliable and secure web applications. In a Spring Boot application, you have various options for implementing field validation, from annotation-based validation to programmatic validation using the Validator
interface.
By applying field validation effectively, you can ensure data integrity, improve the user experience, and enforce business rules and security requirements in your Spring Boot application.
In this blog post, we've explored different approaches to field validation and provided examples demonstrating the implementation and customization of validation processes in a Spring Boot application. I hope this provides you with a solid understanding of field validation best practices in Spring Boot, enabling you to build robust and secure web applications.
Start mastering field validation in your Spring Boot app today for a more reliable and secure user experience!