Boosting Your Code's Shield: TDD for Stronger Security
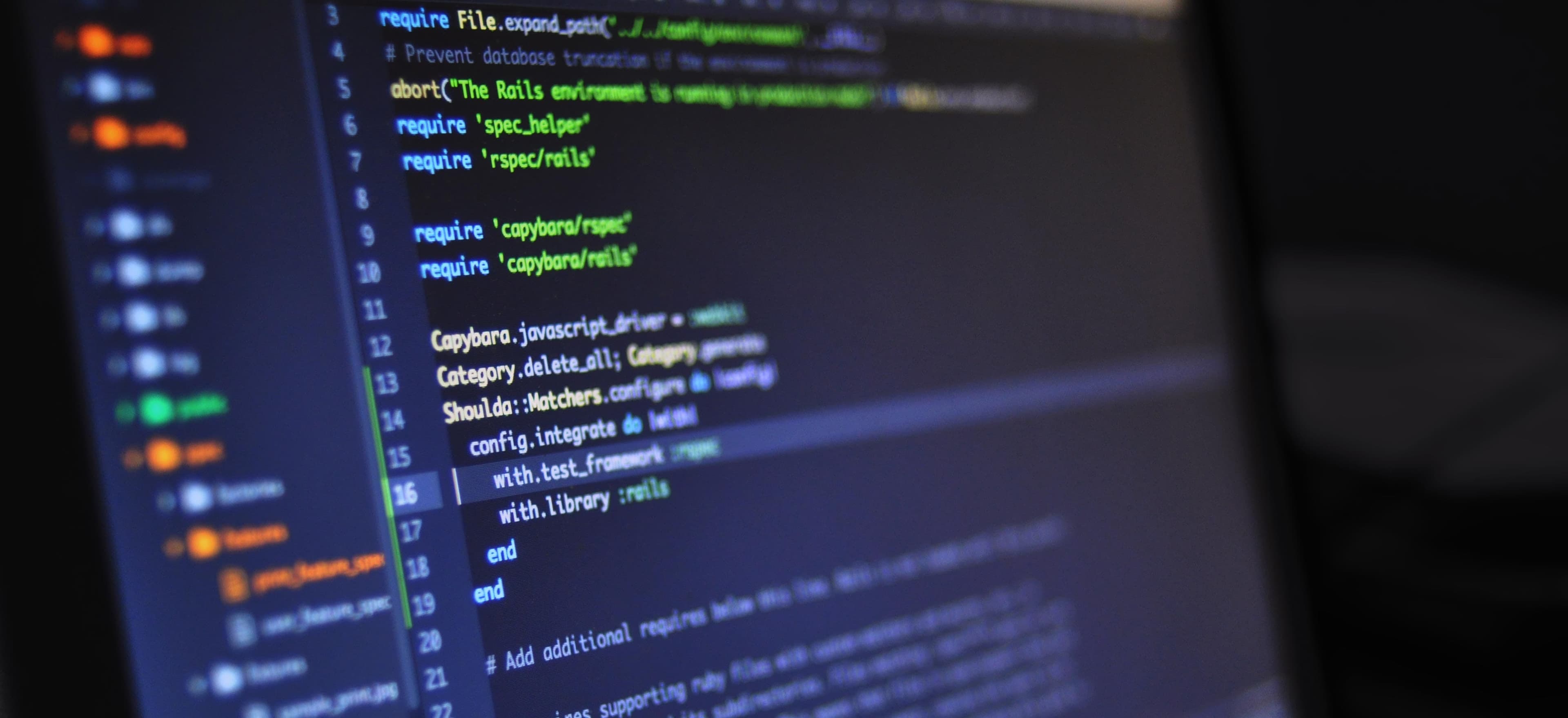
- Published on
Boosting Your Code's Shield: TDD for Stronger Security
In the world of software development, security is paramount. As cyber threats continue to evolve, it's crucial for developers to adopt best practices that ensure the resilience of their code against malicious attacks. One powerful approach to fortifying your software against security vulnerabilities is Test-Driven Development (TDD). By integrating security considerations into the heart of the development process, TDD not only helps in writing robust, bug-free code but also contributes to creating a more secure software architecture.
Understanding Test-Driven Development (TDD)
Test-Driven Development is a development process where tests are written before the actual code implementation. The process generally follows three steps:
- Red: Write a test that checks for the existence of a specific feature
- Green: Write the minimum amount of code to make the test pass
- Refactor: Improve the code without changing its functionality
This iterative approach encourages developers to focus on the requirements and the expected behavior of the code before writing it. As a result, TDD encourages thorough scrutiny of the input validation, error-handling, and other significant aspects that are vital for strengthening the security of a system.
Implementing TDD for Security
When following the TDD approach, security-specific tests can be included along with the functional tests for the system. This proactive inclusion ensures that potential security vulnerabilities are taken into consideration at the very inception of the development process.
Let's take an example where we are developing a simple user authentication module and apply TDD principles with a security focus.
public class UserAuthenticationTest {
@Test
public void testValidCredentials() {
// Test for valid username and password
// Expected outcome: Successful authentication
}
@Test
public void testInvalidCredentials() {
// Test for invalid username and password
// Expected outcome: Authentication failure
}
@Test
public void testSQLInjectionAttack() {
// Test for SQL injection attempt in the authentication process
// Expected outcome: Authentication failure
}
}
In this example, we've incorporated security-focused tests alongside functional tests. The testSQLInjectionAttack
method simulates an attempt to execute a SQL injection attack during the authentication process. By proactively testing for such scenarios, we can identify and mitigate potential security vulnerabilities at an early stage, ensuring a more robust and secure authentication module.
Benefits of TDD for Security
- Proactive Security Measures: By integrating security tests into the development process, potential vulnerabilities can be identified and addressed much earlier in the lifecycle of the code.
- Improved Code Quality: TDD inherently promotes writing modular, loosely-coupled, and easily maintainable code, which contributes to overall system security.
- Effective Refactoring: The refactor step in TDD allows for security improvements to be seamlessly integrated without compromising the functionality of the code.
- Reduced Cost of Security Breaches: Catching security issues early in the development phase can significantly reduce the cost and impact of potential security breaches in the future.
Best Practices for Secure TDD
While TDD can bolster the security of your code, certain best practices can further enhance its effectiveness in ensuring a robust and secure software development process:
1. Thorough Input Validation
Ensure that tests cover a wide range of input validation scenarios, including boundary cases, invalid inputs, and potential attack vectors such as SQL injection, cross-site scripting (XSS), and command injection.
2. Mocking Security Dependencies
Use mocking frameworks to simulate interactions with security-sensitive components such as encryption libraries, authentication services, and authorization systems. By doing so, you can thoroughly test the behavior of the code in isolation while ensuring that external security dependencies are also functioning as expected.
3. Security Code Reviews
Incorporate peer code reviews that specifically focus on the security aspects of the code. This can help in identifying potential security loopholes that may have been overlooked during the TDD process.
4. Utilize Secure Coding Guidelines
Adhere to secure coding guidelines and industry best practices throughout the TDD process. Following recognized standards such as OWASP (Open Web Application Security Project) guidelines can significantly enhance the overall security posture of your code.
5. Keep Abreast of Security Threats
Regularly update security tests to include scenarios based on the latest security threats and vulnerabilities. By staying informed about emerging security issues, you can ensure that your TDD approach aligns with the evolving threat landscape.
Bringing It All Together
Incorporating security considerations into the TDD workflow can significantly bolster the resilience of your code against potential security risks. By proactively testing for security vulnerabilities and adhering to best practices, developers can build software that not only meets functional requirements but also stands strong against malicious attacks. TDD for security is not just a development practice; it's a proactive stance against the ever-evolving landscape of cybersecurity threats.
By adhering to TDD with a security-first mindset, developers can fortify their code's shield, ensuring that it remains impervious to the ever-persistent attempts of malicious entities to exploit vulnerabilities for their gain. Embracing TDD for security is a proactive step towards building a safer digital ecosystem for businesses and end-users alike.
So, are you ready to embrace TDD for stronger security in your software development endeavors?
Feel free to share your thoughts and experiences in the comments below!