Boost Neo4j in Java Apps: Master Spring's Custom Queries!
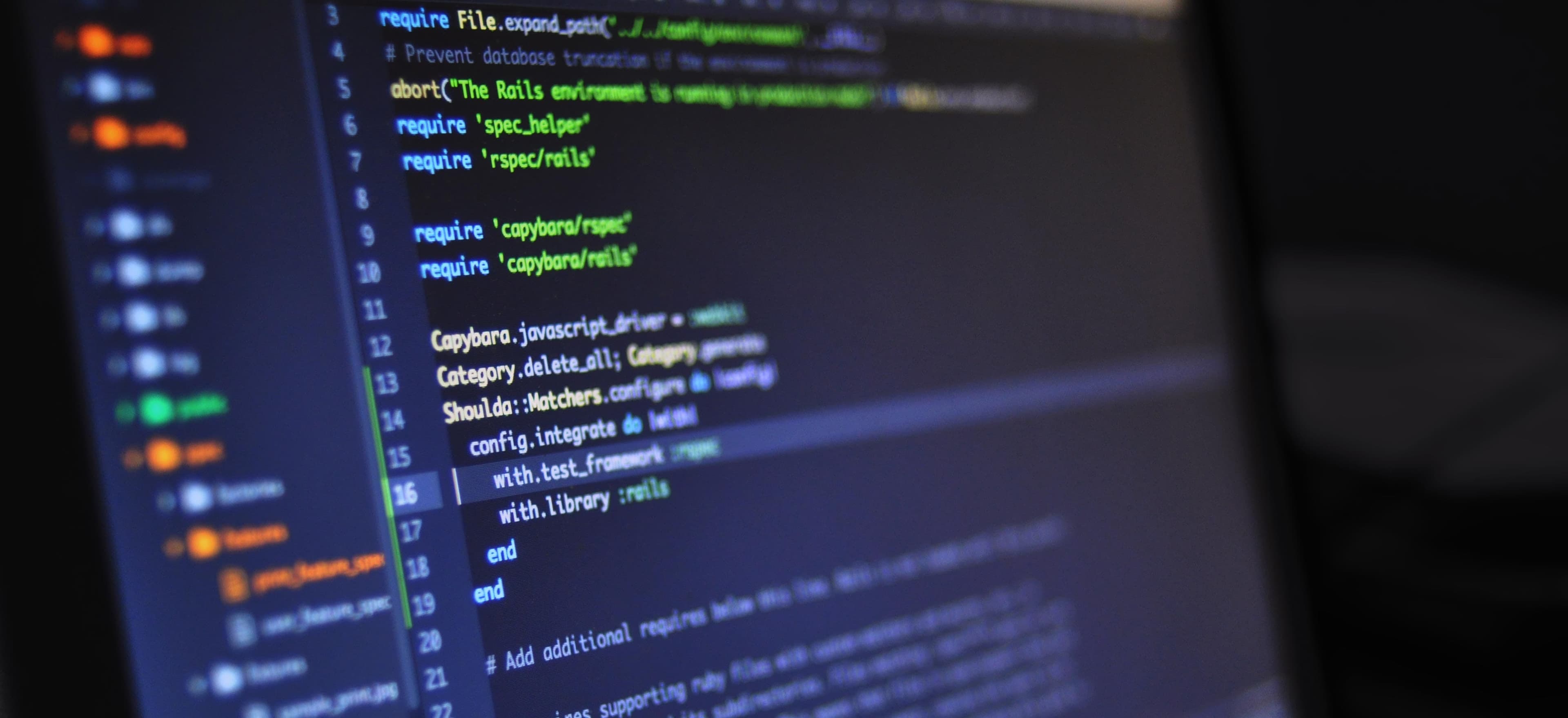
- Published on
Boost Neo4j in Java Apps: Master Spring's Custom Queries!
Neo4j is a highly scalable native graph database that has become a popular choice for managing and querying highly connected data. In Java applications, integrating Neo4j using the Spring Data Neo4j framework can greatly simplify the process of working with graph databases. This blog post will guide you through the process of mastering custom queries in Spring Data Neo4j, empowering you to leverage the full potential of Neo4j in your Java applications.
Understanding Custom Queries in Spring Data Neo4j
When working with Neo4j in Java applications, Spring Data Neo4j provides a powerful mechanism for defining custom queries to fetch, modify, or delete data from the graph database. Custom queries in Spring Data Neo4j are written using the "@Query" annotation and can be tailored to specific use cases, allowing you to go beyond the basic CRUD operations and express complex graph traversal and manipulation logic.
Leveraging the @Query Annotation
The "@Query" annotation in Spring Data Neo4j allows you to define Cypher queries directly within your Java code, providing a seamless way to interact with the Neo4j database. Let's take a look at how you can use the "@Query" annotation to create custom queries for various scenarios.
Fetching Data
Suppose you have a "User" node entity in your Neo4j database, and you want to fetch all users who have a specific role. You can define a custom query using the "@Query" annotation as follows:
@Query("MATCH (u:User)-[:HAS_ROLE]->(r:Role {name: $roleName}) RETURN u")
List<User> getUsersByRole(@Param("roleName") String roleName);
In this example, the custom query fetches all users connected to a specific role node in the graph database.
Modifying Data
If you need to update properties of nodes based on certain criteria, you can use a custom query to achieve this. Let's say you want to increment the "points" property of all users who have completed a specific task. Here's how you can define the custom query:
@Query("MATCH (u:User)-[:COMPLETED]->(t:Task {name: $taskName}) SET u.points = u.points + $bonusPoints")
void rewardUsersForTaskCompletion(@Param("taskName") String taskName, @Param("bonusPoints") int bonusPoints);
In this example, the custom query modifies the "points" property of users who have completed a specific task by adding the specified bonus points.
Deleting Data
Custom queries can also be used to delete nodes or relationships based on certain conditions. For instance, if you want to delete all inactive users from the database, you can define a custom query as follows:
@Query("MATCH (u:User) WHERE NOT u.active DELETE u")
void deleteInactiveUsers();
In this example, the custom query deletes all inactive user nodes from the graph database.
The Power of Custom Queries
Custom queries in Spring Data Neo4j empower you to express complex graph traversal and manipulation operations that go beyond the capabilities of standard repository methods. By leveraging the "@Query" annotation, you can harness the full power of Cypher queries to interact with your Neo4j database in a flexible and efficient manner.
Best Practices for Writing Custom Queries
While custom queries offer great flexibility, it's essential to follow best practices to ensure optimal performance and maintainability of your Java applications.
1. Parameterize Your Queries
Always parameterize your custom queries to prevent potential Cypher injection attacks and to promote query efficiency. Parameterized queries also enable query plan caching, which can lead to significant performance improvements.
2. Use Indexes and Constraints
Ensure that appropriate indexes and constraints are defined in your Neo4j database to support the predicates and patterns used in your custom queries. This can significantly enhance query performance, especially for large datasets.
3. Keep Queries Maintainable
Avoid overly complex or convoluted custom queries. If a particular query logic is frequently reused across different parts of your application, consider encapsulating it into a reusable method or function to maintain clean and maintainable code.
4. Leverage Profiling Tools
Use Neo4j's built-in query profiling tools to analyze the performance of your custom queries. This can help identify potential bottlenecks and optimize query execution.
5. Testing Custom Queries
Unit test your custom queries to ensure their correctness and performance under various scenarios. Tools like Spring Test and Neo4jTest are invaluable for writing comprehensive test cases for your custom queries.
By adhering to these best practices, you can create efficient, maintainable, and secure custom queries that seamlessly integrate with your Java applications.
Wrapping Up
Mastering custom queries in Spring Data Neo4j empowers you to harness the full potential of Neo4j in your Java applications. The "@Query" annotation provides a powerful mechanism for defining custom Cypher queries, enabling you to fetch, modify, and delete data from the graph database with ease. By following best practices and leveraging the flexibility of custom queries, you can build high-performance, scalable, and maintainable Java applications that make the most of Neo4j's capabilities.
Take your Java and Neo4j integration to the next level by mastering custom queries with Spring Data Neo4j!
Ready to delve deeper into Spring Data Neo4j? Check out the official documentation for Spring Data Neo4j and explore the full potential of graph databases in Java applications.