Mastering Ceylon Reduce: Solving Signature Confusion
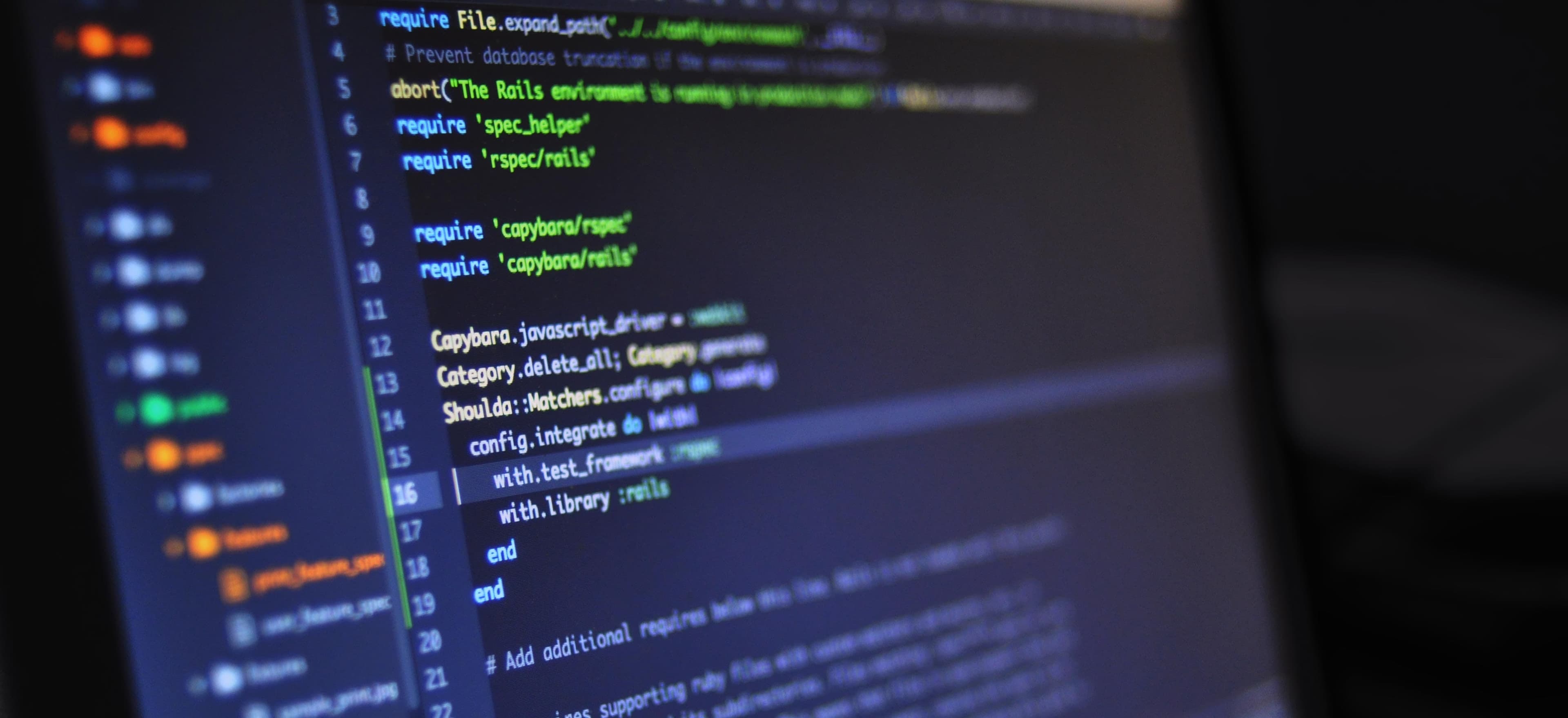
- Published on
Mastering Ceylon Reduce: Solving Signature Confusion
In this blog post, we will explore the reduce function in Ceylon and dive into solving signature confusion that can arise while using reduce. The reduce
function is a powerful tool for processing collections in Ceylon, but it can also be tricky to master, especially when it comes to handling its signature. We will discuss in detail how to effectively use reduce and provide solutions to signature-related issues that developers often encounter.
Before we delve into the details, let's understand what reduce is and why it is a valuable feature in Ceylon.
Understanding Reduce
reduce
is a higher-order function that allows you to iteratively process the elements of a collection to produce a single result. It applies a binary function to the elements of the collection, accumulating a single result. The signature of the reduce
function in Ceylon is as follows:
Result reduce<Element>(Result initialValue, Result*(Element, Result) accumulatingFunction)
The reduce
function takes two parameters:
initialValue
: The initial value for the accumulation.accumulatingFunction
: A binary function that takes an element of the collection and the current accumulated value, and produces a new accumulated value.
You can use reduce
to perform various operations such as summing the elements of a collection, finding the maximum or minimum element, or even constructing a new data structure based on the elements in the collection.
The Signature Confusion
One common problem developers face when using reduce
is signature confusion. Since the accumulating function in reduce
has a specific signature, it may not be immediately clear how to use it for different types of operations. This can lead to confusion and errors in the code.
Let's consider an example to understand this issue better. Say we have a list of integers and we want to find the sum of all the elements using reduce
. The accumulating function for this operation should take an integer element and the current sum, and return the updated sum. The signature of this accumulating function would be (Integer, Integer) -> Integer
.
Here's a simple example of using reduce
to find the sum of a list of integers:
Integer sum = numbers.reduce(0)((element, accumulator) => element + accumulator);
In the above code, 0
is the initial value for the sum, and the accumulating function (element, accumulator) => element + accumulator
calculates the new sum by adding each element to the accumulator.
Solving Signature Confusion
To solve signature confusion while using reduce
, you can make use of Ceylon's type inference and lambda expressions to simplify the code and make it more readable. By utilizing type inference, you can let the compiler infer the types of the parameters and the return type, making the code more concise.
Here's the same sum example, but with type inference and lambda expressions:
Integer sum = numbers.reduce(0)((element, accumulator) => element + accumulator);
With type inference, you don't need to explicitly specify the types of the parameters and the return type in the lambda expression. This simplifies the code and reduces the chance of making errors related to the signature of the accumulating function.
Generic Operations with Reduce
One of the key advantages of using reduce
is its ability to perform generic operations on collections. You can use reduce
to perform a wide range of operations, from simple arithmetic operations to more complex transformations of the elements in the collection.
Let's consider another example where we want to find the maximum element in a list of integers using reduce
. The accumulating function for this operation should take an integer element and the current maximum value, and return the new maximum value. The signature of this accumulating function would be (Integer, Integer) -> Integer
.
Here's how you can use reduce
to find the maximum element in a list of integers:
Integer max = numbers.reduce(numbers.first)((element, max) => if (element > max) then element else max);
In the above code, numbers.first
is used as the initial value for the maximum, and the accumulating function ((element, max) => if (element > max) then element else max)
compares each element with the current maximum and updates it accordingly.
Handling Empty Collections
When using reduce
, you need to handle the case where the collection is empty. Since reduce
requires an initial value, an empty collection would result in the initial value being returned as the result, which may not always be the desired behavior.
To handle empty collections while using reduce
, you can make use of the disjunction
operation to check if the collection is empty, and return a default value in that case.
Here's an example of how to handle empty collections while finding the maximum element:
Integer max = numbers.empty then 0 else numbers.reduce(numbers.first)((element, max) => if (element > max) then element else max);
In the above code, we use the disjunction
operation numbers.empty then 0
to return 0
as the default value if the collection is empty.
Summary
In this blog post, we explored the reduce
function in Ceylon and discussed how to effectively use it for processing collections. We delved into the issue of signature confusion that developers often encounter when using reduce
and provided solutions to handle it. We also demonstrated how to perform generic operations with reduce
and how to handle empty collections effectively.
Mastering reduce
is crucial for efficient collection processing in Ceylon, and understanding its signature and best practices is key to writing clean and effective code.
To learn more about reduce, check out the official documentation on Ceylon reduce.
Checkout our other articles