Mastering Spring Boot: Navigating Context Hierarchy
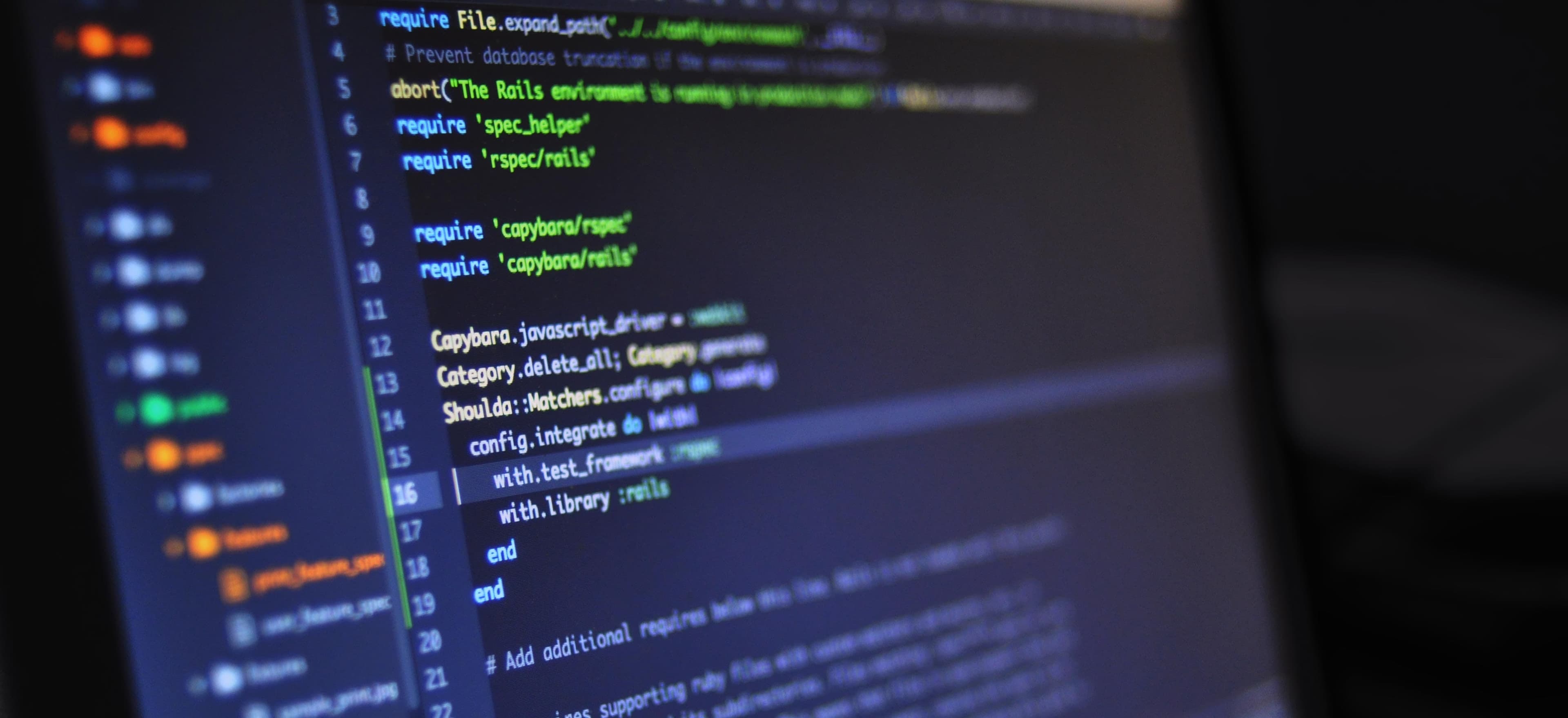
- Published on
Mastering Spring Boot: Navigating Context Hierarchy
When working with complex enterprise applications, understanding the context hierarchy in Spring Boot is essential for managing dependencies and resources effectively. In this post, we will explore the concept of context hierarchy in Spring Boot and how it can be leveraged to streamline application development.
Understanding Context Hierarchy
In Spring Boot, the concept of context hierarchy revolves around the creation and management of multiple application contexts. Each context represents a different layer or module within the application, with a parent-child relationship established between them. This allows for the inheritance of beans and resources from the parent context to the child context, facilitating a modular and organized approach to application development.
Benefits of Context Hierarchy
The use of context hierarchy in Spring Boot offers several benefits:
- Modularity: By breaking down the application into multiple contexts, developers can maintain a modular structure, making it easier to manage and scale the application.
- Inheritance: Child contexts can inherit beans and resources from the parent context, reducing duplicate configurations and promoting code reusability.
- Isolation: Each context operates independently, allowing for isolation of configuration and resources, leading to better maintainability and testability.
- Granular Control: Developers have fine-grained control over the lifecycle of beans and resources within each context, enabling specific configurations for different layers of the application.
Creating a Context Hierarchy
Let's dive into an example to understand how context hierarchy is implemented in Spring Boot. Consider a scenario where we have a web application with separate contexts for the web layer and the service layer.
Web Layer Context
@Configuration
public class WebConfig {
// Web layer configurations and beans
}
Service Layer Context
@Configuration
public class ServiceConfig {
// Service layer configurations and beans
}
In this example, we have two separate configuration classes for the web layer and the service layer. To establish a context hierarchy, we can define the web layer context as the parent context of the service layer context.
Application Context
@SpringBootApplication
public class ApplicationContext extends SpringBootServletInitializer {
@Override
protected SpringApplicationBuilder configure(SpringApplicationBuilder application) {
return application.sources(ApplicationContext.class);
}
public static void main(String[] args) {
SpringApplication.run(ApplicationContext.class, args);
}
}
In the main application context, we can configure the web layer context as the parent context for the service layer context using the @Import
annotation.
Setting up Context Hierarchy
@SpringBootApplication
@Import({WebConfig.class, ServiceConfig.class})
public class ApplicationContext extends SpringBootServletInitializer {
// Application context configuration
}
By setting up the context hierarchy in this manner, the beans and resources defined in the web layer context will be available to the service layer context, establishing a clear parent-child relationship between the two contexts.
Accessing Beans Across Contexts
One of the key advantages of context hierarchy is the ability to access beans defined in the parent context from the child context. This promotes code reusability and streamlined dependency management across different layers of the application.
Accessing Parent Beans
@Service
public class UserService {
@Autowired
private UserRepository userRepository; // Accessing bean from parent context
// Service layer methods
}
In this example, the UserService
in the service layer context can directly access the UserRepository
bean defined in the web layer context, leveraging the context hierarchy to reuse resources and promote a modular architecture.
Final Considerations
Understanding and implementing context hierarchy in Spring Boot is a valuable skill for developers working on complex applications. By leveraging context hierarchy, developers can achieve modularity, code reusability, and fine-grained control over resources, leading to efficient and scalable application development.
Incorporating context hierarchy in Spring Boot projects can contribute to a more maintainable and organized codebase, fostering a systematic approach to managing dependencies and resources across different layers of the application.
In summary, mastering context hierarchy in Spring Boot is a pivotal aspect of building robust and flexible enterprise applications, and developers should strive to leverage this feature to its full potential to achieve optimal code architecture and resource management.
To dive deeper into Spring Boot and its features, check out the official Spring Boot documentation and explore the extensive resources available to enhance your proficiency in Spring Boot development.
Start harnessing the power of context hierarchy in Spring Boot to elevate your application development skills and streamline the management of dependencies across various layers of your projects. Happy coding!