Solving Nested Transactions with ThreadLocal in Java POJOs
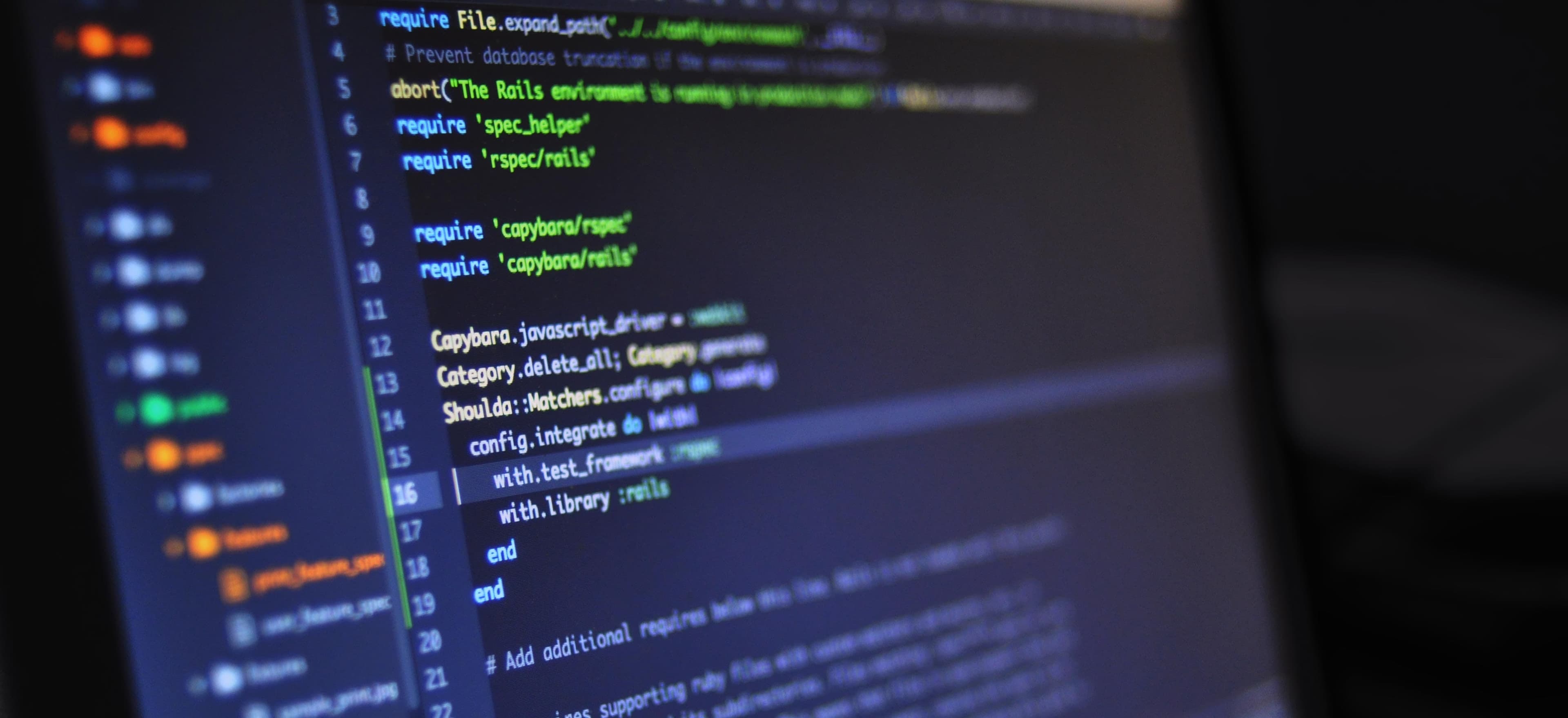
- Published on
Solving Nested Transactions with ThreadLocal in Java POJOs
When working with Java applications, especially those that interact with databases, it's common to encounter the need for nested transactions. Nested transactions occur when a transaction is started within the scope of another transaction. While the JDBC API does not directly support nested transactions, we can work around this limitation by using the ThreadLocal
class in Java to manage transaction state.
In this article, we'll explore how to address the issue of nested transactions using the ThreadLocal
class in Java plain old Java objects (POJOs). We'll start by discussing the concept of nested transactions and why they are useful. Then, we'll delve into a practical example and demonstrate how to use ThreadLocal
to manage nested transactions effectively.
Understanding Nested Transactions
Nested transactions provide a way to logically separate different parts of a transaction to make the code more modular and maintainable. For example, consider a scenario where a service method doTransaction
is responsible for performing a complex operation that involves multiple steps, each of which needs to be treated as an atomic unit of work. In such cases, breaking down the overall operation into nested transactions can make the code easier to understand and maintain.
The Challenge with Nested Transactions in Java
In Java, the JDBC API does not natively support nested transactions. When we attempt to start a new transaction within the scope of an existing transaction, the inner transaction will often inherit the properties of the outer transaction. This behavior can lead to unexpected results and make it challenging to rollback or commit nested transactions independently.
To manage nested transactions effectively, we need a way to isolate the transaction state for each level of nesting. This is where the ThreadLocal
class comes into play.
Leveraging ThreadLocal to Manage Transaction State
The ThreadLocal
class in Java provides a way to store data that is accessible only within the context of a single thread. By using ThreadLocal
, we can create a separate transaction state for each level of nesting, ensuring that nested transactions remain isolated from one another.
Let's consider an example where we have a TransactionManager
class responsible for managing transactions using ThreadLocal
. The TransactionManager
will provide methods to begin, commit, and rollback transactions as well as to retrieve the current transaction for the current thread.
Here's an example of the TransactionManager
class:
public class TransactionManager {
private static ThreadLocal<Transaction> transaction = new ThreadLocal<>();
public static void beginTransaction() {
Transaction tx = new Transaction();
// Start the transaction and set it in the thread-local variable
transaction.set(tx);
}
public static void commitTransaction() {
Transaction tx = transaction.get();
// Commit the transaction
transaction.remove(); // Remove the transaction from the thread-local variable
}
public static void rollbackTransaction() {
Transaction tx = transaction.get();
// Rollback the transaction
transaction.remove(); // Remove the transaction from the thread-local variable
}
public static Transaction getCurrentTransaction() {
return transaction.get();
}
}
In this example, we use a ThreadLocal
variable to store the Transaction
object for the current thread. When a new transaction is started, it is stored in the ThreadLocal
variable, ensuring that each thread has its own isolated transaction state. When the transaction is committed or rolled back, we remove it from the ThreadLocal
variable to clean up the state.
Implementing Nested Transactions Using ThreadLocal
Let's demonstrate how to use the TransactionManager
class to implement nested transactions in a hypothetical service class called EmployeeService
.
public class EmployeeService {
public void performComplexOperation() {
// Start the outermost transaction
TransactionManager.beginTransaction();
try {
// Perform some database operation
// ...
// Start a nested transaction
TransactionManager.beginTransaction();
try {
// Perform another database operation
// ...
// Commit the nested transaction
TransactionManager.commitTransaction();
} catch (Exception e) {
// Handle exceptions and rollback the nested transaction
TransactionManager.rollbackTransaction();
}
// Continue with the outer transaction
// ...
// Commit the outer transaction
TransactionManager.commitTransaction();
} catch (Exception e) {
// Handle exceptions and rollback the outer transaction
TransactionManager.rollbackTransaction();
}
}
}
In this example, the performComplexOperation
method in the EmployeeService
class starts the outermost transaction using the TransactionManager.beginTransaction
method. It then proceeds to start a nested transaction within a try-catch block. If an exception occurs during the nested transaction, it rolls back the nested transaction. Otherwise, it continues with the outer transaction and commits it at the end.
By using ThreadLocal
to manage transaction state, we can ensure that nested transactions are isolated and can be rolled back or committed independently of one another, providing a way to handle complex transactional scenarios effectively.
The Last Word
In this article, we discussed the concept of nested transactions and the challenges associated with implementing them in Java. We explored how the ThreadLocal
class can be leveraged to manage transaction state at the thread level, enabling us to address the limitations of nested transactions in Java.
By implementing a TransactionManager
class that utilizes ThreadLocal
, we demonstrated how to handle nested transactions effectively, ensuring that each level of nesting can be managed independently. When dealing with complex transactional scenarios in Java applications, the approach described in this article can provide a practical solution for managing nested transactions.
Incorporating ThreadLocal
to address nested transactions showcases the adaptability and flexibility of Java when faced with challenges that are not natively supported by the language or its APIs. When handling transactions in Java POJOs, considering the use of ThreadLocal
for managing transaction state can greatly enhance the robustness and maintainability of the codebase.
In conclusion, understanding how to effectively manage nested transactions using ThreadLocal
empowers Java developers to build resilient and scalable applications that can handle complex transactional logic with ease.