Solving the Puzzle: Java 9 Module System Challenges
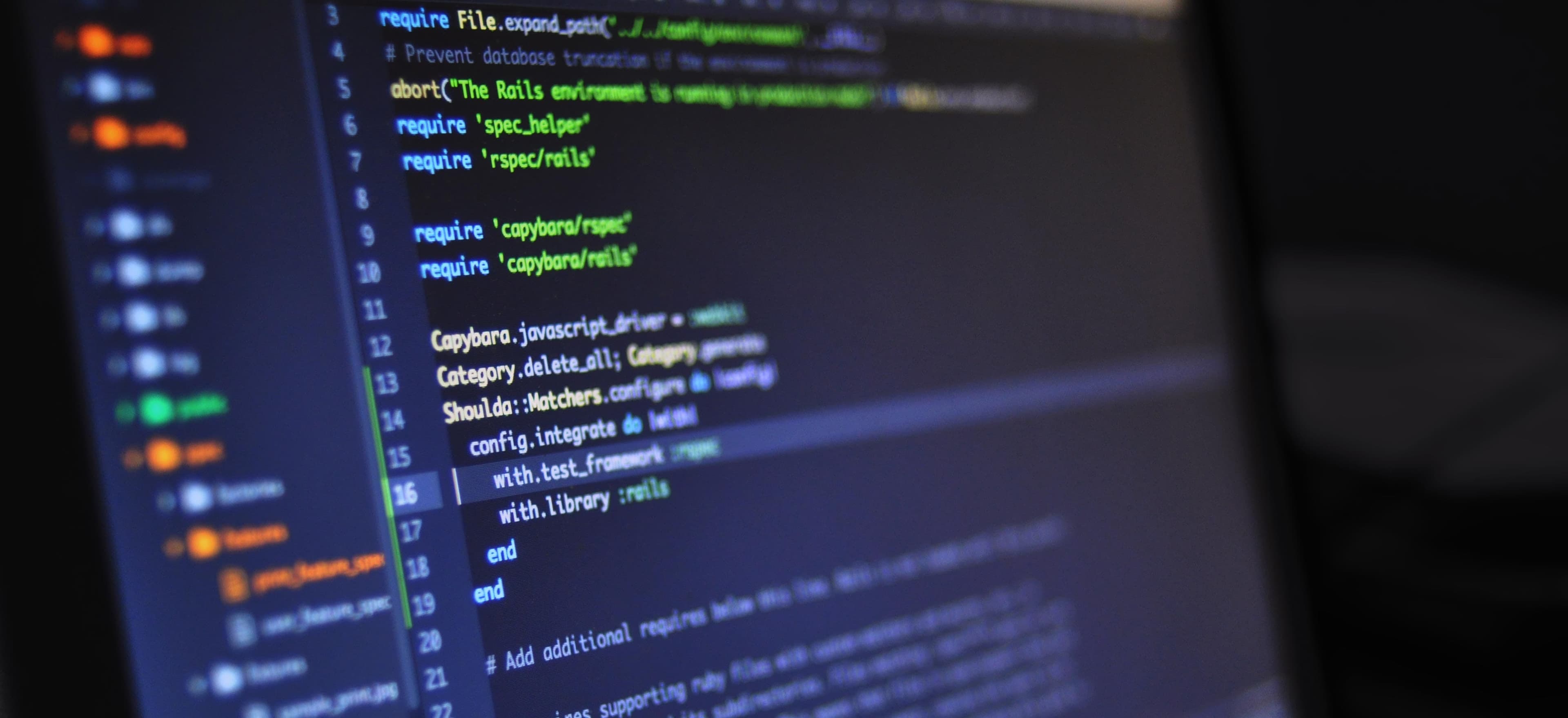
- Published on
Solving the Puzzle: Java 9 Module System Challenges
The release of Java 9 brought a significant change to the way developers structure their applications with the introduction of the Java Platform Module System (JPMS). This new module system aims to solve the long-standing issues of modularity and encapsulation in Java. However, it also presents new challenges for developers to overcome. In this blog post, we will explore some of the key challenges that developers face when working with the Java 9 module system and how to solve them.
Understanding the Java 9 Module System
Before delving into the challenges, it's crucial to have a solid understanding of the Java 9 module system and its core concepts. In Java 9, a module is a collection of related packages and resources with a module descriptor (module-info.java
) that explicitly specifies its dependencies on other modules. This allows developers to define clear boundaries and dependencies between different parts of their applications.
Challenge 1: Modularizing Existing Codebases
One of the significant challenges developers face when migrating to Java 9 is modularizing existing codebases. Many Java applications were not initially designed with modularity in mind, and as a result, they rely on the classpath-based approach to resolve dependencies. When migrating to Java 9, developers need to restructure their applications into modules, which can be a time-consuming and complex task.
To tackle this challenge, developers can start by identifying the core components of their applications and creating modules around these components. They can then gradually modularize the remaining codebase, focusing on resolving inter-module dependencies and ensuring that each module encapsulates its implementation details.
// Example module-info.java
module com.example.core {
exports com.example.core.api;
}
module com.example.feature {
requires com.example.core;
exports com.example.feature.api;
}
In the example above, the com.example.core
module exports its API, which can be accessed by the com.example.feature
module, demonstrating how dependencies are explicitly defined in the module system.
Challenge 2: Managing Module Path and Classpath
With the introduction of the module path in Java 9, developers now have to manage both the module path and the classpath when building and running their applications. This introduces complexity, especially when dealing with libraries and frameworks that have not been modularized.
To address this challenge, developers can use tools like Maven or Gradle, which provide support for the Java 9 module system. These build tools allow developers to declare module descriptors, manage module dependencies, and automate the migration process. Additionally, they can explore the use of tools like jdeps
to analyze dependencies and identify any missing or unused modules.
Challenge 3: Accessing Internal APIs
In traditional Java development, developers have been able to access internal APIs from the sun.misc
package, even though it was not recommended. However, with the module system, access to internal APIs is further restricted, making it challenging for developers who rely on these APIs for certain functionalities.
To overcome this challenge, developers can use reflection to access internal APIs, although this approach should be used cautiously as it bypasses the encapsulation provided by the module system. Alternatively, they can explore alternative APIs provided by the Java SE platform or consider raising a request to make the required functionality available through standard APIs.
Challenge 4: Dealing with Split Packages
Another common challenge that arises when working with the Java 9 module system is dealing with split packages. A split package occurs when the same package is defined in multiple modules, which can lead to runtime errors and classpath conflicts.
To resolve split package issues, developers can refactor their code to eliminate the split packages by reorganizing the affected classes into separate packages or modules. Additionally, they can utilize tools like jdeps
to detect split packages and take appropriate actions to resolve them.
Challenge 5: Testing and Integration with Legacy Code
When migrating to Java 9, developers often encounter difficulties testing and integrating their modularized code with legacy code that has not been migrated to modules. This can result in compatibility issues and hinder the seamless adoption of the module system.
To address this challenge, developers can adopt a phased approach, where they modularize and test individual components of their applications while ensuring backward compatibility with non-modularized code. They can also make use of Java 9's backward-compatibility features to ease the integration process, such as the automatic module naming and the ability to use non-modularized JARs within modularized code.
In Conclusion, Here is What Matters
In conclusion, the Java 9 module system brings significant improvements to modularity and encapsulation in Java applications. However, it also presents several challenges for developers, such as modularizing existing codebases, managing module path and classpath, accessing internal APIs, dealing with split packages, and integrating with legacy code. By understanding these challenges and implementing the suggested solutions, developers can effectively navigate the complexities of the Java 9 module system and build robust, modular applications.
To delve deeper into the Java 9 module system and its intricacies, you can explore the official Java Platform, Standard Edition 9 Documentation and the Java 9 Module System documentation provided by Oracle.
So, what challenges have you faced when working with the Java 9 module system, and how did you solve them? Let's continue the conversation in the comments below!