Step-by-Step: Easily Replace A Build Module using Veripacks
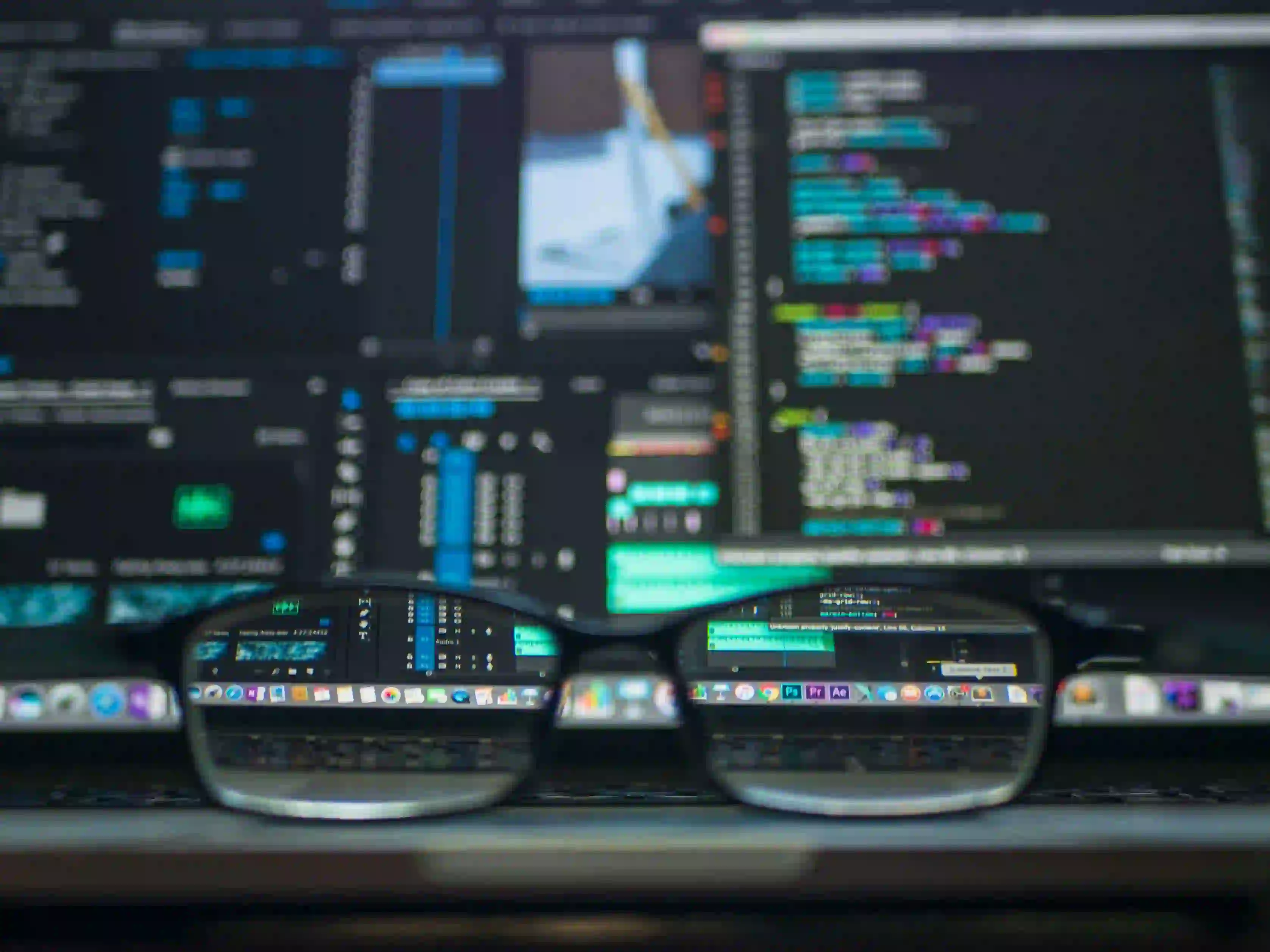
Step-by-Step: Easily Replace A Build Module using Veripacks
In Java development, managing dependencies can often be a complex and challenging task. One popular approach to simplifying dependency management is through the use of Veripacks. Veripacks provides a way to replace a build module with a different implementation, making the application more modular and flexible.
In this step-by-step guide, we will walk through the process of replacing a build module using Veripacks. By following these steps, you can enhance the modularity of your Java application and make it easier to maintain and extend.
Let's get started!
Step 1: Create a Java Project
First, create a new Java project, or use an existing one if you have a project where you want to apply Veripacks. For the purpose of this tutorial, we will assume you have a Maven-based project. You can use the following Maven archetype to create a new project:
mvn archetype:generate -DgroupId=com.example -DartifactId=my-java-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
Step 2: Add Veripacks Dependency
Once you have your Java project set up, you need to add the Veripacks dependency to your project's pom.xml
file. Include the following dependency:
<dependency>
<groupId>io.veripacks</groupId>
<artifactId>veripacks</artifactId>
<version>1.3</version>
</dependency>
This will allow you to use the Veripacks library to manage your module dependencies.
Step 3: Create Modules
In order to replace a build module, you first need to have multiple modules in your project. Create at least two modules, each with their own set of classes and dependencies. For example, you can have ModuleA
and ModuleB
, each with their respective classes.
Step 4: Define Module Dependency Rules
Next, you need to define the module dependency rules using Veripacks' DSL (Domain Specific Language). This DSL allows you to specify the dependencies between modules and define the rules for module replacement. Here's an example of how you can define the module dependency rules in a veripack-config.dsl
file:
modules {
module("ModuleA") {
dependents += module("ModuleB")
}
}
In this example, we specify that ModuleA
depends on ModuleB
. This configuration can be expanded to include more complex dependency rules as needed.
Step 5: Replace Module
Now, let's say we want to replace ModuleB
with a different implementation, ModuleBNew
. To do this, you need to create the new module and update the module dependency rules in the veripack-config.dsl
file.
modules {
module("ModuleA") {
dependents += module("ModuleBNew")
}
}
By making this change in the veripack-config.dsl
file, you are effectively replacing the original ModuleB
with ModuleBNew
.
Step 6: Run Veripacks Processor
Finally, you need to run the Veripacks processor to apply the module replacement and validate the module dependencies. Add the Veripacks processor to your project's build configuration in the pom.xml
file:
<build>
<plugins>
<plugin>
<groupId>io.veripacks</groupId>
<artifactId>veripacks-maven-plugin</artifactId>
<version>1.3</version>
<executions>
<execution>
<goals>
<goal>verify</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
Once you have added the Veripacks processor to your build configuration, running the mvn verify
command will trigger the Veripacks processor to validate the module dependencies and apply the module replacements as defined in the veripack-config.dsl
file.
My Closing Thoughts on the Matter
In this step-by-step guide, we have explored how to easily replace a build module using Veripacks in a Java project. By following these steps, you can effectively manage module dependencies and enhance the modularity of your application. Veripacks provides a powerful way to define and enforce module dependency rules, making it easier to maintain and extend your Java projects.
To learn more about Veripacks and its capabilities, you can visit the official Veripacks documentation. Additionally, you can explore more advanced features and use cases to further enhance your Java development experience with Veripacks.
Now that you have a grasp of how to use Veripacks, try implementing it in your own projects and see the benefits of improved modularity and flexibility firsthand. Happy coding!