Overcoming the Queue Quandary: Fixing SQS Poison Messages
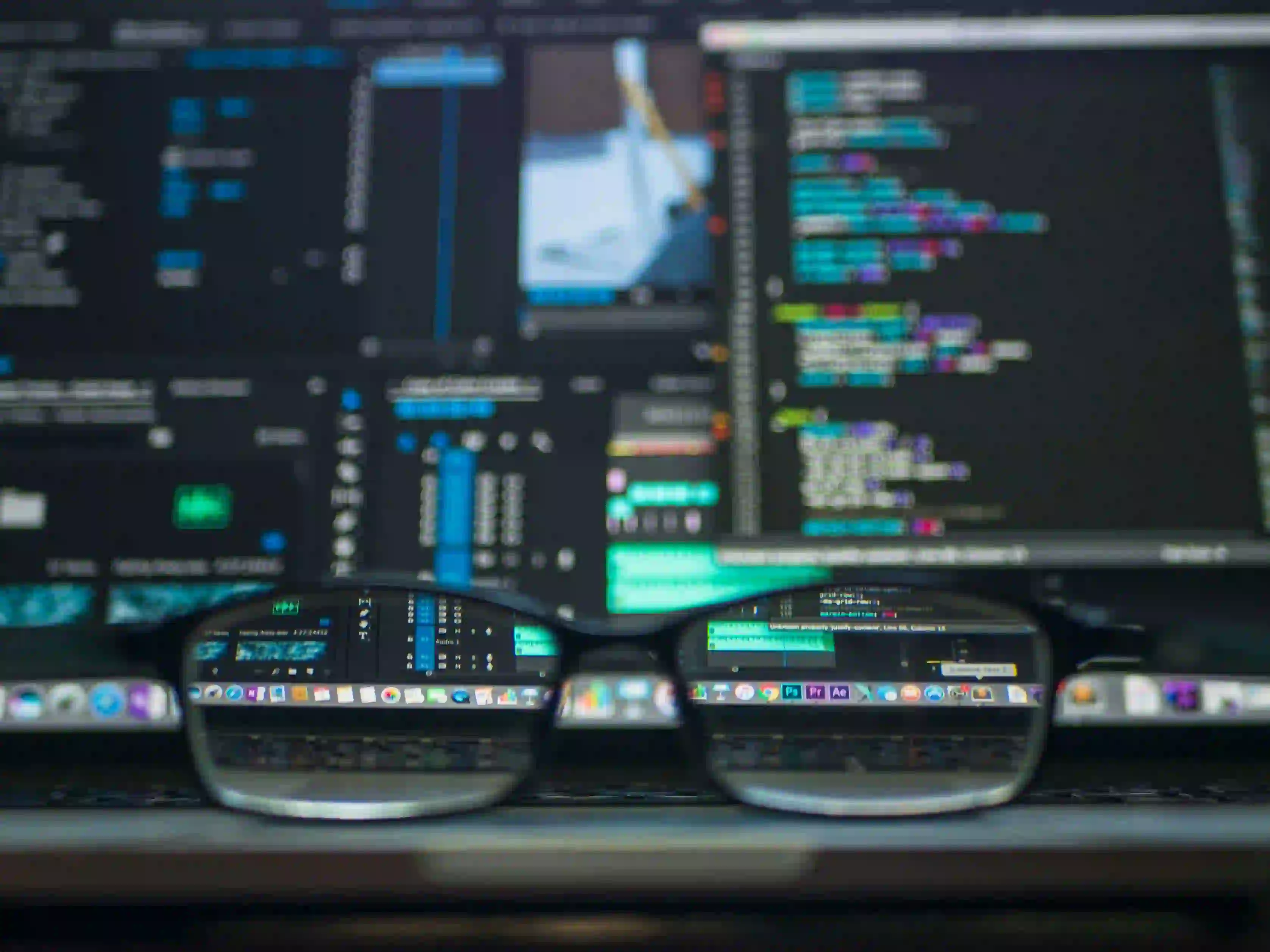
Overcoming the Queue Quandary: Fixing SQS Poison Messages
Amazon Simple Queue Service (SQS) is a reliable, scalable, fully managed message queuing service that enables decoupling and asynchronous communication between distributed software components. However, one common issue that can disrupt the smooth operation of SQS is the occurrence of poison messages.
Understanding Poison Messages
Poison messages are problematic messages that, upon processing, cause failures or exceptions in the consumer application. These messages can get stuck in the queue, leading to a processing bottleneck, and potentially causing cascading failures in downstream systems.
Identifying the Problem
When encountering poison messages, it's crucial to determine their cause. It could be due to malformed content, incorrect message handling, or even external factors such as sudden spikes in load or unexpected changes in message format.
Implementing a Solution
To address the challenge of poison messages in Amazon SQS, a robust error-handling strategy combined with a systematic approach can be employed to rectify the issue. Here, we will explore strategies to mitigate poison messages through code-level adjustments and infrastructure enhancements.
Dead-Letter Queues
Amazon SQS provides Dead-Letter Queues (DLQs) as a feature to handle problematic messages. When a message fails to be processed after a certain number of retries, it can be redirected to a DLQ for further analysis and troubleshooting. By setting up a DLQ, poison messages can be systematically isolated and managed, allowing the main queue to remain unimpacted.
Example of setting up a Dead-Letter Queue in Java using the AWS SDK:
CreateQueueRequest createQueueRequest = new CreateQueueRequest("MainQueue")
.addAttributesEntry("RedrivePolicy",
"{\"maxReceiveCount\":\"5\", \"deadLetterTargetArn\":\"arn:aws:sqs:us-east-1:123456789012:DeadLetterQueue\"}");
CreateQueueResult createQueueResult = sqs.createQueue(createQueueRequest);
In this example, a DLQ named "DeadLetterQueue" is created and associated with the main queue "MainQueue". If a message is received more than 5 times without being successfully processed, it will be moved to the DLQ for further analysis.
Backoff and Retrying Mechanisms
Implementing exponential backoff and retry logic can help in handling transient issues that lead to poison messages. By gradually increasing the delay between retries, the system can alleviate load on downstream components and potentially recover from transient failures.
Example of implementing backoff and retry logic in Java:
int maxRetries = 3;
for (int retry = 0; retry < maxRetries; retry++) {
try {
// Process message
break; // If successful, exit the loop
} catch (Exception e) {
// Apply exponential backoff
Thread.sleep((int) Math.pow(2, retry) * 1000);
}
}
In this example, the code attempts to process the message with a maximum of 3 retries. If an exception occurs, the system applies an exponential backoff by increasing the wait time between retries.
Monitoring and Alerting
Establishing comprehensive monitoring capabilities and alerting mechanisms is essential for timely detection and mitigation of poison messages. Leveraging Amazon CloudWatch and setting up custom metrics and alarms allows for proactive identification of abnormal patterns and facilitates swift intervention.
By combining these strategies, developers can effectively tackle the menace of poison messages in Amazon SQS, ensuring the seamless flow of messages and the robustness of the overall system.
Final Considerations
Handling poison messages in Amazon SQS demands a multi-faceted approach comprising error handling, retry mechanisms, and systematic monitoring. By leveraging features such as Dead-Letter Queues, implementing backoff and retry logic, and establishing robust monitoring, developers can effectively address the challenges posed by poison messages, ensuring the reliability and resilience of the message queuing system.
Addressing poison messages optimally ensures smooth operation, safeguards downstream systems from disruptions, and upholds the fundamental tenets of message queuing architectures – reliability, scalability, and decoupling. By embracing these best practices, organizations can harness the full potential of Amazon SQS while mitigating the impact of poison messages on their distributed systems.