Boosting App Performance with Generational Caching & Envers
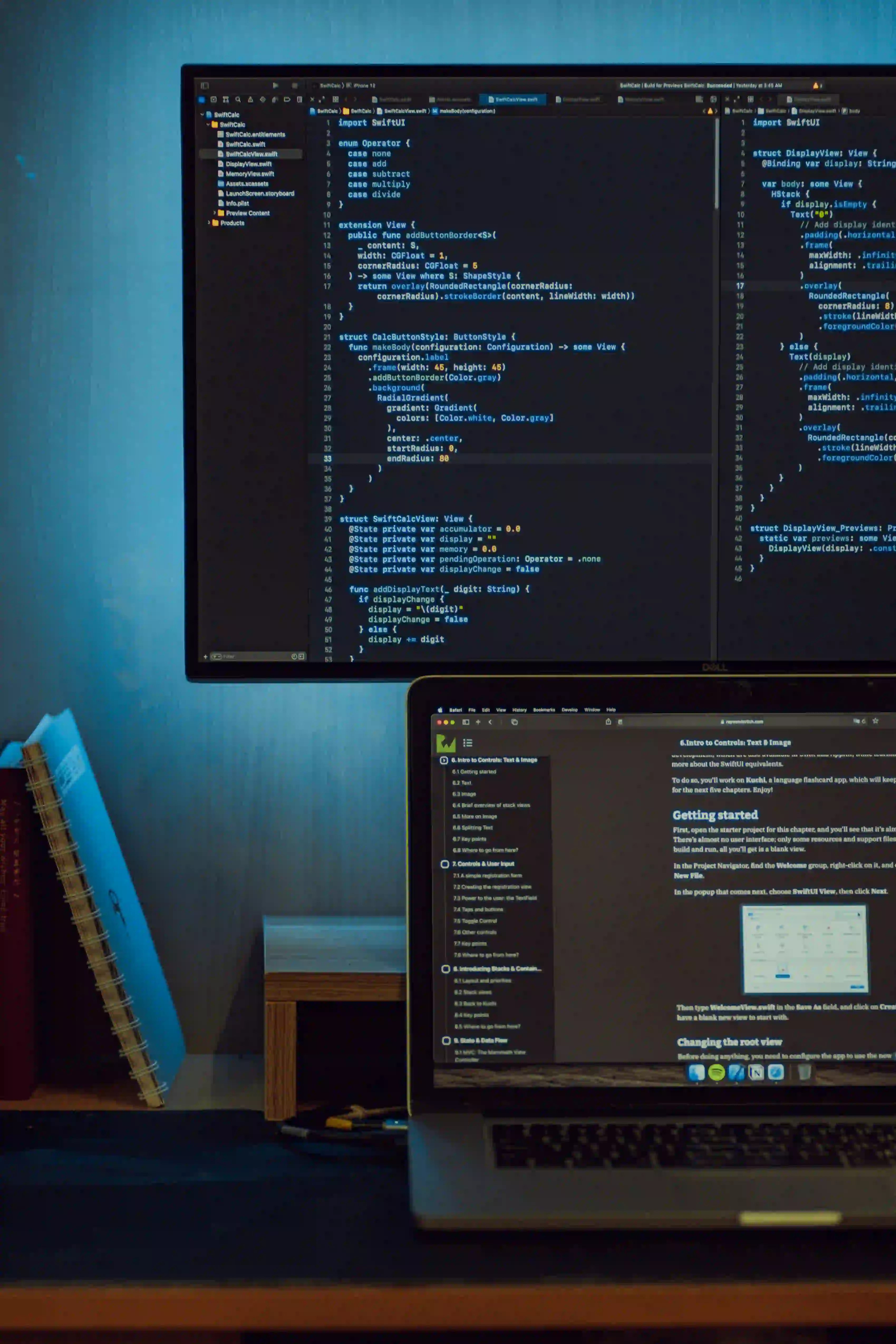
Boosting App Performance with Generational Caching & Envers
In our quest to enhance app performance, we often delve into various strategies and tools. Two powerful techniques for improving application performance are generational caching and Envers. These techniques not only optimize app performance but also add flexibility and robustness to the application.
Generational Caching
Generational caching is a caching strategy that leverages the concept of generations to efficiently manage and retrieve cached data. By categorizing cache entries into different generations, applications can optimize memory usage and access speed.
Key Benefits of Generational Caching
-
Reduced Memory Footprint: By segregating cached data based on generations, older and less frequently accessed data can be evicted from the cache, reducing memory usage.
-
Improved Access Speed: By organizing cached data into generations, applications can prioritize access to frequently used data, thereby improving retrieval speeds.
-
Enhanced Cache Management: Generational caching simplifies cache management by providing a structured approach to handle different categories of data.
Implementing Generational Caching in Java
Let's explore a simple example of how to implement generational caching in a Java application.
import java.util.HashMap;
import java.util.Map;
public class GenerationalCache {
private Map<String, String> youngGeneration = new HashMap<>();
private Map<String, String> oldGeneration = new HashMap<>();
public void addToYoungGeneration(String key, String value) {
youngGeneration.put(key, value);
}
public String getFromYoungGeneration(String key) {
return youngGeneration.get(key);
}
// Additional methods for managing oldGeneration cache
public static void main(String[] args) {
GenerationalCache cache = new GenerationalCache();
cache.addToYoungGeneration("1", "Data 1");
String data = cache.getFromYoungGeneration("1");
System.out.println(data);
}
}
In the above example, we create a GenerationalCache
class with separate maps for young and old generations. The addToYoungGeneration
and getFromYoungGeneration
methods demonstrate the management of the young generation cache. The application can further extend this implementation to manage the old generation cache while maintaining the generational approach.
Envers
Envers is a powerful auditing and versioning tool for Hibernate-based applications. It enables automatic versioning of entities, allowing for historical data retrieval and tracking changes to entities over time.
Key Benefits of Envers
-
Auditing & Compliance: Envers facilitates auditing and compliance requirements by capturing and storing historical changes to entities, providing a comprehensive view of data evolution.
-
Querying Historical Data: Envers simplifies the querying of historical data, allowing applications to retrieve and compare previous versions of entities effortlessly.
-
Data Integrity & Accountability: By keeping track of entity modifications, Envers promotes data integrity and accountability within applications.
Implementing Envers in Java with Hibernate
Let's consider a basic example to demonstrate the implementation of Envers in a Java application using Hibernate.
@Entity
@Audited
public class Product {
@Id
@GeneratedValue
private Long id;
@Column
private String name;
// Getters and setters
}
In this example, the Product
entity is annotated with @Audited
to enable versioning with Envers. Any modifications to instances of the Product
entity will be automatically versioned by Envers, allowing for historical data retrieval and tracking changes.
Combining Generational Caching and Envers for Enhanced Performance
When used in conjunction, generational caching and Envers offer a potent combination for enhancing application performance and data management.
Efficient Data Retrieval
By applying generational caching, frequently accessed data is readily available, improving data retrieval performance. Meanwhile, Envers ensures that historical data versions are efficiently stored and retrievable, enabling applications to access and compare historical data with ease.
Optimal Memory Management
Generational caching optimizes memory usage by evicting older and less frequently accessed data, while Envers manages historical data versions efficiently. This dual approach ensures that memory resources are utilized optimally, enhancing overall application performance.
Comprehensive Data Management
The combination of generational caching and Envers provides a comprehensive solution for data management. While generational caching focuses on optimizing real-time data access, Envers caters to historical data tracking and compliance, resulting in a balanced and robust data management strategy.
The Last Word
As performance optimization continues to be a critical aspect of application development, leveraging techniques such as generational caching and Envers can significantly enhance an application's performance, memory management, and data tracking capabilities. By implementing these techniques effectively, developers can ensure that their applications are not only high-performing but also robust and adaptable to evolving data requirements.
In conclusion, incorporating generational caching and Envers into Java applications can elevate performance, foster efficient memory utilization, and empower comprehensive data management, thereby contributing to a seamless and resilient user experience.
To explore more about generational caching and Envers, delve into the official documentation for Ehcache, a widely used caching framework in Java, and the official documentation for Hibernate Envers, which provides extensive insights into utilizing Envers for auditing and versioning.