Solving Many-to-Many Relationships in REST: A Guide
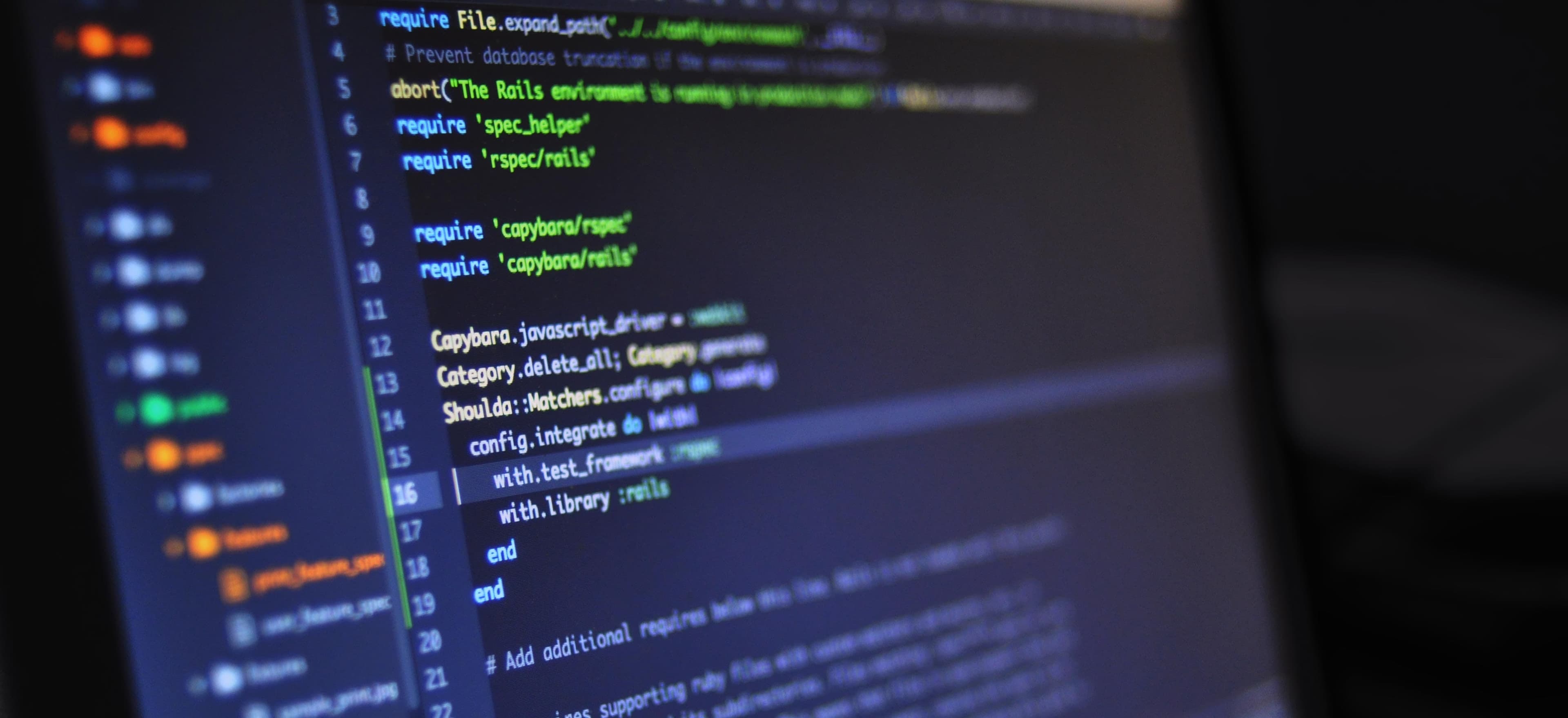
- Published on
Solving Many-to-Many Relationships in REST: A Guide
When designing RESTful APIs, representing many-to-many relationships between resources can be tricky. In this post, we will explore different approaches to handling many-to-many relationships in REST, focusing on Java-based solutions.
The Challenge of Many-to-Many Relationships
In a typical database, many-to-many relationships are represented using a junction table that contains foreign keys from both related entities. But in RESTful APIs, the challenge is how to represent and manage these relationships using the principles of REST and HTTP.
Approach 1: Using Subresources
One common approach is to use subresources to represent many-to-many relationships. In this approach, each subresource represents a specific relationship between two entities. For example, consider a scenario where User
can have multiple Roles
. Instead of directly exposing a many-to-many relationship between User
and Role
, we can create a subresource called UserRole
to represent the relationship.
Example Code
@Path("/users/{userId}/roles")
public class UserRoleResource {
@POST
public Response assignRoleToUser(@PathParam("userId") long userId, Role role) {
// Assign the role to the user
return Response.status(Response.Status.CREATED).build();
}
@DELETE
public Response removeRoleFromUser(@PathParam("userId") long userId, @QueryParam("roleId") long roleId) {
// Remove the role from the user
return Response.status(Response.Status.NO_CONTENT).build();
}
}
Why This Approach?
Using subresources provides a clear and explicit way to manage many-to-many relationships. It allows us to define specific operations for manipulating the relationship and keeps the API endpoints clean and focused on individual resources.
Approach 2: Using Request Parameters
Another approach is to use request parameters to represent many-to-many relationships. Instead of creating subresources, we can use query parameters to add or remove related entities.
Example Code
@Path("/users/{userId}/roles")
public class UserResource {
@POST
public Response assignRoleToUser(@PathParam("userId") long userId, @QueryParam("roleId") long roleId) {
// Assign the role to the user
return Response.status(Response.Status.CREATED).build();
}
@DELETE
public Response removeRoleFromUser(@PathParam("userId") long userId, @QueryParam("roleId") long roleId) {
// Remove the role from the user
return Response.status(Response.Status.NO_CONTENT).build();
}
}
Why This Approach?
Using request parameters simplifies the API endpoints and makes the operations more straightforward. It can be easier to understand and use, especially for simple many-to-many relationships.
Key Takeaways
Managing many-to-many relationships in RESTful APIs requires careful consideration of the best approach for representing and manipulating these relationships. Subresources and request parameters are two common strategies, each with its own advantages and trade-offs.
By understanding these approaches, you can make informed decisions when designing your APIs and ensure that your many-to-many relationships are handled efficiently and intuitively.
To delve deeper into RESTful API design and Java development, consider exploring resources like Java's JAX-RS for creating RESTful web services in Java and this comprehensive guide by Baeldung for RESTful API development with Spring.