Reviving Java: The Raw String Literals Dilemma Unraveled
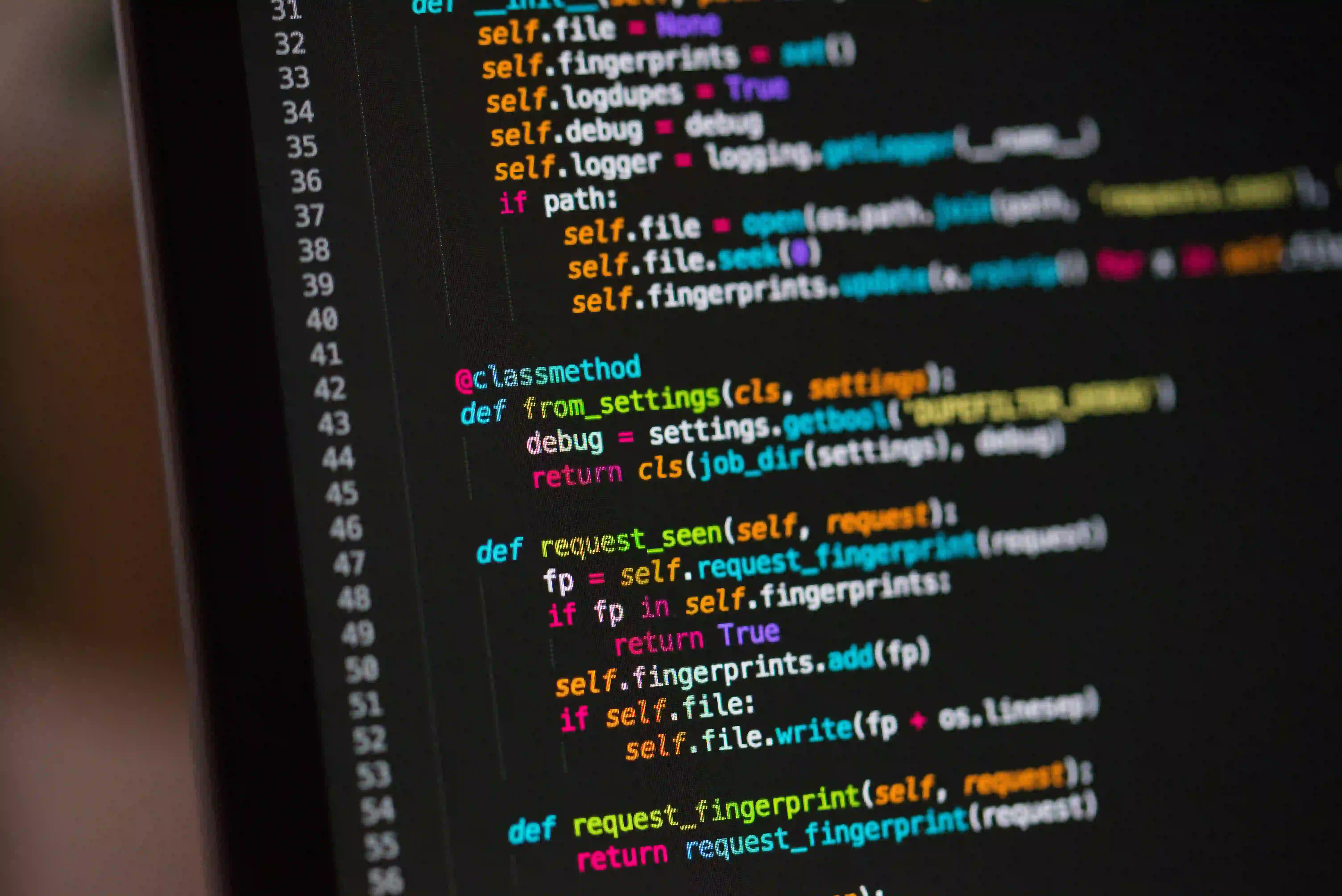
Reviving Java: The Raw String Literals Dilemma Unraveled
As a Java developer, staying abreast of the latest language features and enhancements is crucial to writing efficient, elegant, and maintainable code. In this post, we'll delve into the intricacies of raw string literals in Java, exploring their significance, the challenges they aim to solve, and how they can be harnessed to elevate the quality of your code.
Understanding the Need for Raw String Literals
Java has long been criticized for its verbose and cumbersome syntax when it comes to handling multi-line strings and strings containing special characters. Prior to the introduction of raw string literals, developers had to resort to cumbersome workarounds such as concatenating multiple string literals or using escape characters to represent special characters within a string.
Consider a scenario where you need to define an HTML snippet within a Java string. Prior to raw string literals, the code would be cluttered with escape characters and line breaks, making it arduous to comprehend and maintain:
String htmlSnippet = "<div class=\"container\">\n\t<h1>Hello, World!</h1>\n</div>";
The Birth of Raw String Literals in Java
With the release of Java 13, the much-awaited feature of raw string literals was introduced, providing a concise and expressive syntax for defining multi-line strings and strings containing special characters. Raw string literals are delimited by backticks (`) and allow for the inclusion of line breaks and special characters without the need for escape sequences.
Let's see how the aforementioned HTML snippet can be elegantly represented using raw string literals:
String htmlSnippet = `
<div class="container">
<h1>Hello, World!</h1>
</div>
`;
The adoption of raw string literals in Java serves to enhance code readability, maintainability, and reduces the cognitive load on developers when working with complex string literals.
Unraveling the Syntax of Raw String Literals
Raw string literals in Java aim to strike a balance between expressiveness and clarity. By embracing a minimalistic approach, Java ensures that the syntax remains intuitive and aligns with developers' expectations.
The backtick (`) symbol serves as the delimiter for raw string literals, marking the beginning and end of the string. The inclusion of backticks prevents the need for escaping backslashes and other special characters commonly found in strings.
In cases where a backtick needs to be included within the raw string literal itself, the backtick can be repeated twice to escape it, as demonstrated in the following snippet:
String stringWithBacktick = `` `This is a string with a backtick: ` ``;
Embracing the Flexibility of Raw String Literals
Raw string literals in Java excel not only in simplifying the representation of multi-line strings but also in accommodating the inclusion of backticks within the string without causing ambiguity.
Consider a scenario where you need to define a SQL query containing backticks within the string. Prior to the advent of raw string literals, this would have necessitated the careful placement of escape characters to distinguish between the backticks used for delimiting identifiers and those intended as part of the string literal.
Let's illustrate how raw string literals alleviate the burden of dealing with backticks within the string:
String sqlQuery = `
SELECT * FROM `users` WHERE age > 18
`;
Notice how the raw string literal allows the seamless inclusion of backticks within the string, eliminating the need for convoluted escape sequences and enhancing the overall legibility of the code.
Overcoming the Pitfalls and Limitations
While raw string literals bring significant improvements to the handling of multi-line strings and strings containing special characters, it's important to note that they come with their own set of limitations and trade-offs.
One notable limitation is the inability to include a backtick immediately preceding or succeeding the opening/closing delimiter, as it would lead to ambiguity in determining the boundary of the raw string literal. This necessitates the use of concatenation or traditional string literals in scenarios where such a requirement arises.
Additionally, raw string literals cannot span across multiple lines if they are to be included within annotations, as the presence of line breaks within annotations is disallowed by the Java language specification.
Practical Applications of Raw String Literals
The introduction of raw string literals in Java opens up a myriad of opportunities for streamlining the representation of complex strings in various domains, ranging from defining SQL queries and HTML templates to embedding JSON payloads within Java code.
Consider the task of defining a JSON payload within a Java application. Raw string literals provide an elegant solution, as demonstrated in the following snippet:
String jsonPayload = `
{
"name": "John Doe",
"age": 30,
"email": "john.doe@example.com"
}
`;
By leveraging raw string literals, the verbosity and clutter associated with traditional string representations of JSON payloads are alleviated, leading to more concise and readable code.
Embracing Forward Compatibility
It's crucial to acknowledge that the incorporation of raw string literals in Java signifies a significant step towards aligning the language with modern programming paradigms and bolstering its position in the ever-evolving landscape of software development.
As newer versions of Java continue to evolve, it's imperative for developers to embrace and leverage the features introduced, including raw string literals, to ensure that their code remains aligned with best practices and harnesses the latest language capabilities.
My Closing Thoughts on the Matter
In conclusion, the introduction of raw string literals in Java marks a pivotal milestone in simplifying the representation of multi-line strings and strings containing special characters. By alleviating the need for cumbersome escape sequences and convoluted workarounds, raw string literals elevate the readability and maintainability of Java code, fostering a more expressive and elegant coding experience.
As Java developers, it's paramount to not only embrace the adoption of raw string literals but to also explore their practical applications across diverse domains, from defining SQL queries and HTML templates to encapsulating JSON payloads within Java code. By doing so, we stand to harness the full potential of raw string literals, ushering in a new era of clarity and conciseness in Java string manipulation.
For further exploration of raw string literals and the latest advancements in Java, refer to the official Java documentation and the Java Enhancement Proposals (JEPs). Happy coding!