Solving Java Modules: A Beginner's Biggest Hurdle
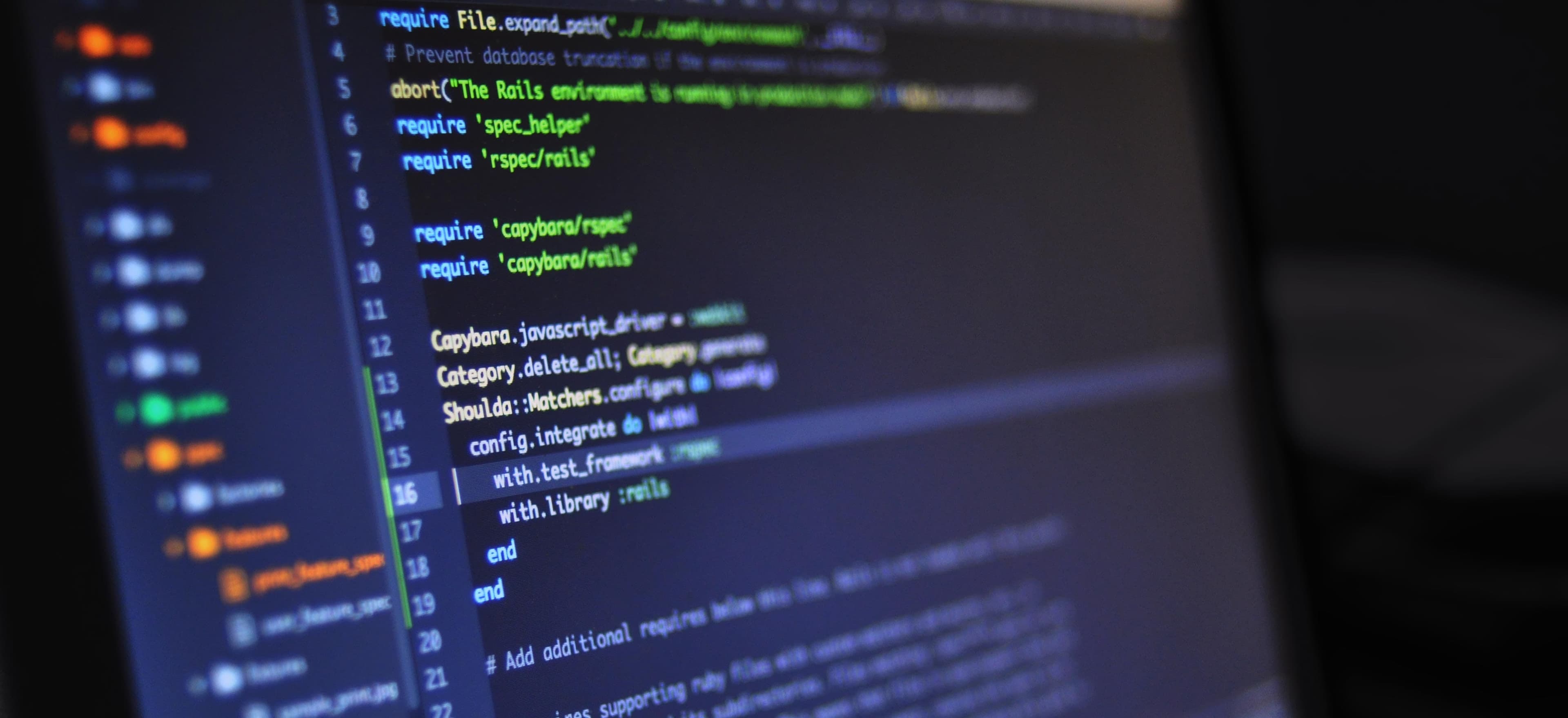
- Published on
Solving Java Modules: A Beginner's Biggest Hurdle
Java has been one of the most popular programming languages for several years. However, the release of Java 9 brought a major change to the way developers work with Java - the introduction of modules. While modules offer benefits such as better encapsulation and improved maintainability, they can also present significant challenges for beginners. In this post, we'll explore the concept of Java modules and provide practical solutions to help beginners overcome the hurdles they may face.
Understanding Java Modules
In traditional Java development, applications consist of packages and JAR files. Java modules introduce a higher level of abstraction to the codebase by creating explicit boundaries and dependencies. This allows developers to organize code more effectively and manage dependencies more rigorously.
The module-info.java
File
The core of Java modules lies in the module-info.java
file, which is used to define a module. This file specifies the module's name, dependencies on other modules, and the packages it exports. Here's an example of a module-info.java
file:
module com.example.myapp {
requires java.sql;
exports com.example.myapp.util;
}
In this example, the module "com.example.myapp" requires the module "java.sql" and exports the package "com.example.myapp.util".
Challenges Faced by Beginners
Lack of Familiarity
For developers accustomed to the traditional Java project structure, adapting to modules can be challenging. Understanding how modules fundamentally change the architecture and how they interact with one another requires a shift in mindset.
Managing Dependencies
While modules provide a better way to manage dependencies, beginners may struggle with defining and resolving module dependencies correctly. This includes understanding transitive dependencies and ensuring all necessary modules are accessible.
Migration from Existing Projects
For developers transitioning existing projects to Java modules, the task can be daunting. It involves restructuring the codebase, resolving module dependencies, and dealing with potential compatibility issues.
Strategies for Overcoming the Hurdles
Learn the Basics
Start by familiarizing yourself with the fundamental concepts of Java modules. Oracle's official Java Platform, Standard Edition Oracle JDK Documentation provides comprehensive resources for understanding modules.
Utilize IDE Support
Modern Integrated Development Environments (IDEs) such as IntelliJ IDEA and Eclipse have robust support for Java modules. Take advantage of features like code completion, module dependency resolution, and project migration tools to simplify the transition to modular development.
Use Modular Libraries
When working with external libraries, look for those that provide modular JAR files. These libraries are designed to work seamlessly with Java modules, making dependency management more straightforward.
Adopt Best Practices
Follow best practices for defining module boundaries, managing dependencies, and organizing code within modules. This includes adhering to the principle of least privilege when exporting packages and keeping module interfaces minimal.
Employ Modularity Patterns
Explore modularity patterns such as the Service Provider Interface (SPI) to decouple modules and improve extensibility. Understanding these patterns can help you harness the full potential of modular architecture.
Practical Example: Creating and Using Java Modules
Let's walk through a practical example to demonstrate the creation and usage of Java modules. We'll create two modules - com.example.calculator
and com.example.app
- where com.example.app
depends on com.example.calculator
.
Step 1: Create the Modules
Create a directory structure for the modules:
calculatorModule
└── src
└── com.example.calculator
├── module-info.java
└── Calculator.java
appModule
└── src
└── com.example.app
├── module-info.java
└── App.java
Step 2: Define the Modules
Define the module-info.java
for the com.example.calculator
module:
module com.example.calculator {
exports com.example.calculator;
}
Define the module-info.java
for the com.example.app
module:
module com.example.app {
requires com.example.calculator;
}
Step 3: Implement the Modules
Implement the Calculator
class in the com.example.calculator
module:
package com.example.calculator;
public class Calculator {
public int add(int a, int b) {
return a + b;
}
}
Implement the App
class in the com.example.app
module:
package com.example.app;
import com.example.calculator.Calculator;
public class App {
public static void main(String[] args) {
Calculator calculator = new Calculator();
System.out.println("Addition result: " + calculator.add(5, 3));
}
}
Step 4: Compile and Run the Modules
Compile the modules using the javac
command and run the App
module using the java
command:
javac -d out --module-source-path calculatorModule/src calculatorModule/src/com.example.calculator/module-info.java calculatorModule/src/com.example.calculator/Calculator.java
javac -d out --module-source-path appModule/src appModule/src/com.example.app/module-info.java appModule/src/com.example.app/App.java
java --module-path out -m com.example.app/com.example.app.App
By following these steps, you've created and utilized Java modules, successfully managing dependencies between them.
In Conclusion, Here is What Matters
While Java modules may present a significant learning curve for beginners, with the right approach and resources, overcoming the initial hurdles is entirely achievable. By understanding the fundamental principles, leveraging IDE support, adopting best practices, and exploring practical examples, developers can seamlessly transition to modular Java development. Embracing modularity not only improves code organization and maintainability but also prepares developers for the future of Java development.
For further exploration, check out the "Modular Programming in Java 9" guide on Baeldung.
Start your modular Java journey today, and unlock the full potential of modular architecture!
Checkout our other articles