Top Tool Woes: Streamlining Java Testing Integration
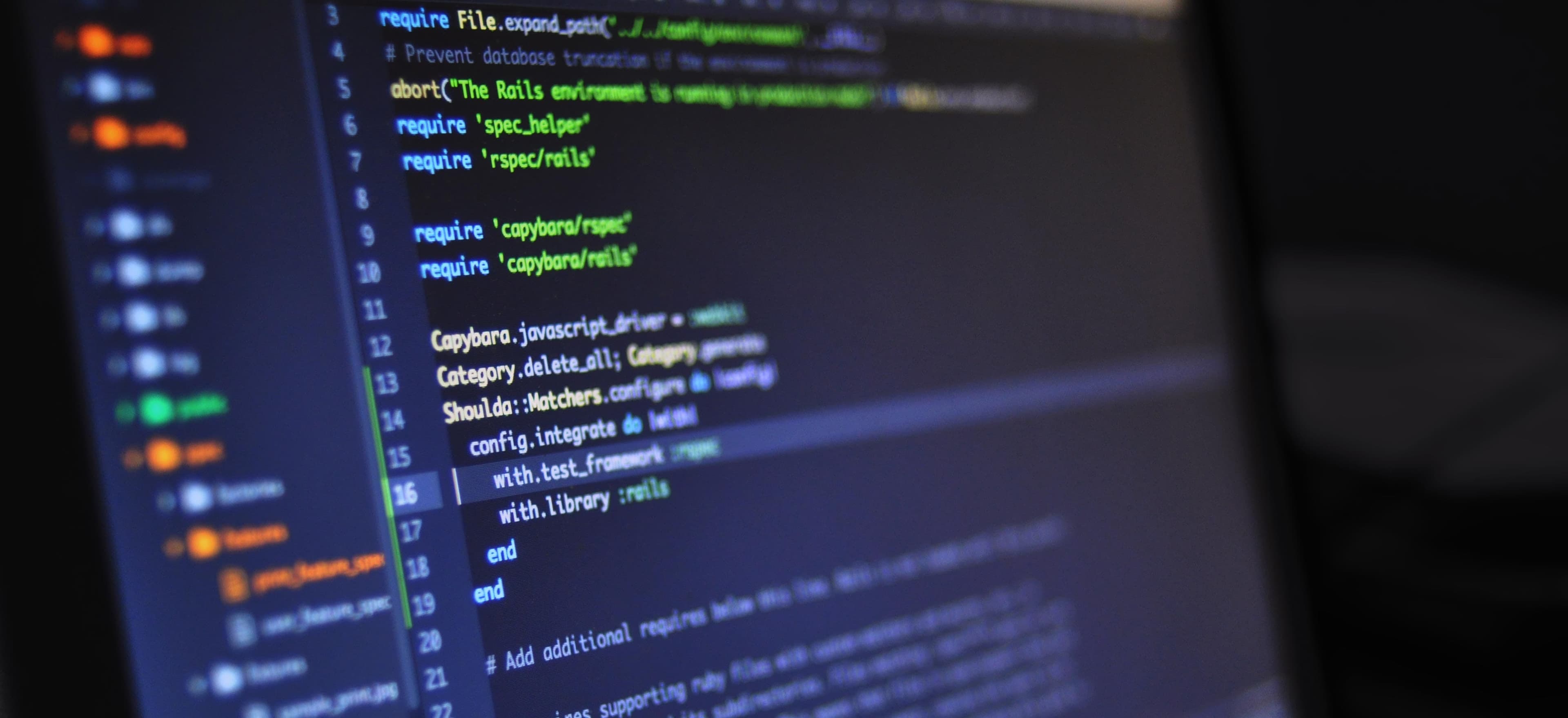
- Published on
Top Tool Woes: Streamlining Java Testing Integration
When it comes to Java development, testing is an indispensable part of the process. Quality testing ensures the reliability and efficiency of your Java applications. However, integrating testing into your Java development workflow can be a challenging task. This is where the right tools and practices come into play to streamline Java testing integration.
In this blog post, we will delve into the common challenges developers face when integrating testing into their Java projects, and explore some effective strategies and tools for streamlining the process.
The Testing Conundrum
Developers often encounter a range of issues when it comes to the integration of testing in their Java projects:
-
Complex Configuration: Setting up testing frameworks and tools can be complex and time-consuming.
-
Maintenance Overhead: Managing and maintaining test suites, especially in large codebases, can become cumbersome.
-
Integration with CI/CD: Seamless integration of testing with continuous integration and continuous deployment pipelines is crucial but often poses challenges.
Streamlining the Testing Integration Process
Embracing JUnit for Unit Testing
JUnit stands out as a robust framework for writing and running repeatable tests. Its annotation-driven approach simplifies the task of writing unit tests in Java. Let's take a look at a simple JUnit test case for a hypothetical Calculator
class:
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 4);
assertEquals(7, result);
}
}
In this example, the @Test
annotation marks the method as a test case. The assertEquals
method validates the expected result against the actual result. This simplifies the process of writing and executing unit tests.
Leveraging Mockito for Mocking Dependencies
In modern Java development, leveraging external dependencies is inevitable. However, testing classes with external dependencies can be challenging. This is where Mockito comes to the rescue by providing capabilities for mocking objects.
Consider a scenario where the Calculator
class depends on a MathService
:
public class Calculator {
private MathService mathService;
public Calculator(MathService mathService) {
this.mathService = mathService;
}
public int performComplexCalculation(int a, int b) {
int resultFromMathService = mathService.performOperation(a, b);
// Perform complex calculation based on resultFromMathService
return result;
}
}
Using Mockito, we can easily mock the MathService
for testing the Calculator
class without the need for a real implementation:
import static org.mockito.Mockito.*;
public class CalculatorTest {
@Test
public void testPerformComplexCalculation() {
MathService mathService = mock(MathService.class);
when(mathService.performOperation(3, 4)).thenReturn(7);
Calculator calculator = new Calculator(mathService);
int result = calculator.performComplexCalculation(3, 4);
assertEquals(14, result);
}
}
Mockito's expressive syntax allows us to simulate the behavior of the MathService
and focus solely on testing the Calculator
class in isolation.
Harnessing JUnit Jupiter for Parameterized Testing
Parameterized testing is valuable for testing with multiple inputs. JUnit Jupiter provides built-in support for parameterized tests, allowing us to run the same test with different arguments.
Let's consider a parameterized test for a FactorialCalculator
:
import org.junit.jupiter.params.ParameterizedTest;
import org.junit.jupiter.params.provider.ValueSource;
import static org.junit.jupiter.api.Assertions.assertEquals;
public class FactorialCalculatorTest {
@ParameterizedTest
@ValueSource(ints = { 0, 1, 2, 3, 4, 5 })
public void testCalculateFactorial(int number) {
FactorialCalculator calculator = new FactorialCalculator();
int expectedResult = calculateFactorial(number);
assertEquals(expectedResult, calculator.calculateFactorial(number));
}
}
In this example, the @ParameterizedTest
annotation enables parameterized testing with a range of input values, reducing the need for writing multiple test cases.
Simplifying Test Configuration with Spring Boot
Spring Boot excels in simplifying the configuration of Java applications, including testing. It provides a comprehensive set of tools for testing, such as auto-configured testing annotations and utilities.
Consider a sample integration test for a Spring Boot application:
@SpringBootTest
class UserServiceIntegrationTest {
@Autowired
private UserService userService;
@Test
void testUserCreation() {
User user = new User("John Doe");
userService.createUser(user);
assertNotNull(user.getId());
}
}
In this example, the @SpringBootTest
annotation initializes the Spring application context, allowing the UserService
to be autowired for seamless integration testing. Spring Boot's testing support simplifies the setup and execution of integration tests for Spring-based applications.
Integrating Testing with CI/CD Pipelines
A robust testing strategy is incomplete without seamless integration into your CI/CD pipelines. Utilizing tools like Jenkins and Travis CI can automate the execution of tests as part of the deployment process. This not only ensures the stability of your codebase but also accelerates the feedback loop for developers.
By configuring your CI/CD pipelines to trigger tests upon each code commit or pull request, you can catch potential issues early in the development lifecycle, promoting a more robust and reliable codebase.
Lessons Learned
Effectively integrating testing into your Java development workflow is crucial for delivering high-quality, reliable software. By leveraging tools like JUnit, Mockito, JUnit Jupiter, and Spring Boot, along with seamless CI/CD pipeline integration, you can streamline the testing process and ensure the resilience of your Java applications.
With the right tools and practices in place, the once daunting task of testing integration becomes a seamless and integral part of the Java development process.
Remember, a well-tested codebase is the cornerstone of robust and scalable applications!
In conclusion, remember that testing is not only about finding bugs, but also about designing better software. It’s about enforcing good architectural practices. Happy testing!
Now, go forth with confidence, armed with the tools and knowledge to conquer the challenges of testing integration in Java development. Good luck!