Boost Your Java EE Apps with ManagedExecutorService!
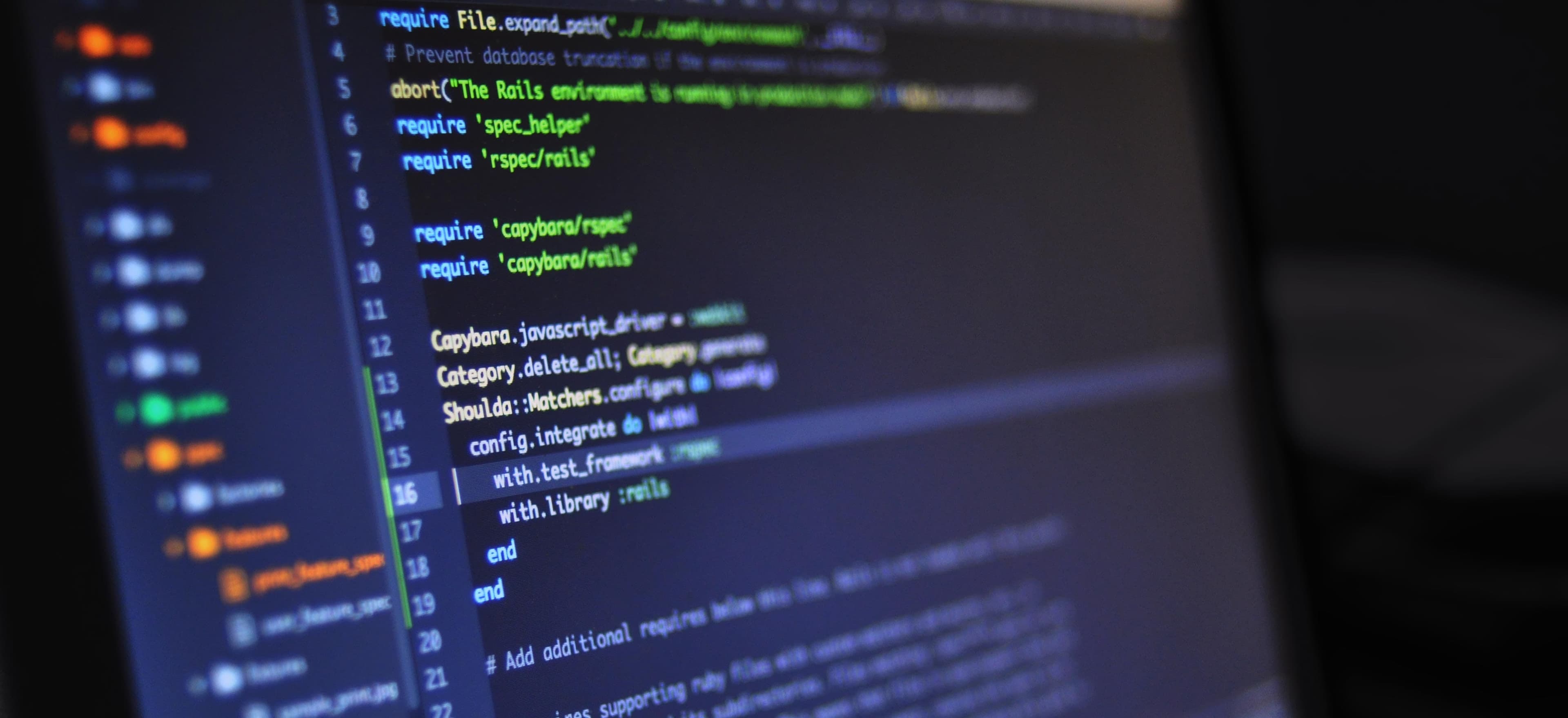
- Published on
Boost Your Java EE Apps with ManagedExecutorService!
When it comes to developing high-performance and efficient Java Enterprise Edition (Java EE) applications, threading and concurrency management are crucial factors. In the world of Java EE, ManagedExecutorService is a powerful feature that can significantly enhance the performance and scalability of your applications.
In this article, we will explore the benefits and capabilities of ManagedExecutorService in Java EE and understand how it can be leveraged to optimize the concurrency management in your applications.
Understanding ManagedExecutorService
ManagedExecutorService is a part of the Java EE concurrency utilities that were introduced as a part of the Java EE 7 platform. It is an interface that extends the ExecutorService
interface, providing capabilities for managed task execution and asynchronous processing within a Java EE environment.
One of the key advantages of ManagedExecutorService is its integration with the container-managed thread pools, which allows for efficient utilization of resources and better alignment with the Java EE concurrency model.
Key Benefits of Using ManagedExecutorService
1. Integration with Container-Managed Thread Pools
The ManagedExecutorService leverages the container-managed thread pools provided by the Java EE container. This means that the execution of tasks is handled within the context of these managed thread pools, ensuring better control and optimization of resources. By utilizing container-managed thread pools, you can prevent resource contention and efficiently utilize the available threads, resulting in improved application performance.
2. Context Propagation
ManagedExecutorService supports context propagation, which allows the propagation of contextual information from the caller's execution context to the asynchronous task's execution context. This is particularly beneficial in a Java EE environment, where the tasks may need access to contextual information such as security context, transaction context, and other contextual data.
3. Resource Management and Monitoring
Another advantage of ManagedExecutorService is its support for resource management and monitoring. The container provides visibility and control over the managed executor, allowing for monitoring of task execution, thread utilization, and resource allocation. This level of oversight can be instrumental in identifying performance bottlenecks and optimizing the application's concurrency behavior.
Implementing ManagedExecutorService in Java EE Applications
Now, let's dive into a practical example of how ManagedExecutorService can be implemented in a Java EE application to harness its benefits.
1. Dependency Injection
First, we need to inject the ManagedExecutorService
into our application. This can be done using the @Resource
annotation to leverage the container's resource injection capabilities.
import javax.annotation.Resource;
import javax.enterprise.concurrent.ManagedExecutorService;
public class MyManagedExecutorBean {
@Resource
private ManagedExecutorService managedExecutorService;
// Additional code for task execution using the managedExecutorService
}
In this example, the ManagedExecutorService
is injected into a CDI managed bean, allowing us to utilize its capabilities for asynchronous task execution.
2. Submitting Tasks for Execution
Once the ManagedExecutorService
is injected, we can submit tasks for execution using its submit()
method. This method accepts a Callable
or Runnable
task and returns a Future
representing the asynchronous computation.
managedExecutorService.submit(() -> {
// Task execution logic
return result;
});
By submitting tasks to the ManagedExecutorService
, we delegate the task execution to the container-managed thread pool, taking advantage of its resource management and optimized concurrency behavior.
3. Configuring ManagedExecutorService
The configuration of the ManagedExecutorService
can be done through the Java EE application server's administration console or through application deployment descriptors. This allows for fine-tuning of parameters such as core pool size, maximum pool size, keep-alive time, and queue capacity to align the managed executor with the application's concurrency requirements.
Best Practices and Considerations
While ManagedExecutorService offers substantial benefits, there are certain best practices and considerations to keep in mind when incorporating it into your Java EE applications.
1. Task Granularity
It's essential to consider the granularity of tasks submitted to the ManagedExecutorService
. Fine-grained tasks can lead to excessive overhead due to thread allocation and context switching. On the other hand, overly coarse-grained tasks may underutilize the available threads. Finding the right balance is crucial for optimal concurrency management.
2. Error Handling
Proper error handling and fault tolerance mechanisms should be implemented for tasks submitted to the ManagedExecutorService
. This includes handling exceptions thrown by the asynchronous tasks and ensuring that the application remains stable in the face of failures.
3. Monitoring and Tuning
Regular monitoring of the managed executor's performance metrics and behavior is important for identifying any performance bottlenecks or inefficiencies. Tuning the managed executor's configuration based on the application's workload and concurrency patterns can lead to significant performance improvements.
The Last Word
In conclusion, ManagedExecutorService is a valuable component for optimizing concurrency management in Java EE applications. By leveraging its integration with container-managed thread pools, support for context propagation, and resource management capabilities, developers can enhance the performance, scalability, and efficiency of their Java EE applications.
Incorporating ManagedExecutorService into your Java EE applications requires a clear understanding of its benefits, proper utilization through dependency injection, and conscientious configuration and monitoring to ensure optimal concurrency behavior.
Java EE developers can leverage ManagedExecutorService to harness the full potential of concurrent processing while aligning with the best practices and considerations outlined in this article. As you continue to enhance the performance of your Java EE applications, consider the ManagedExecutorService as a powerful tool in your concurrency management arsenal.
Start optimizing your Java EE applications with ManagedExecutorService today for efficient concurrent processing and improved scalability!
To delve deeper into Java EE concurrency and ManagedExecutorService, JBoss Developer provides excellent resources and hands-on examples for further exploration.
Disclaimer: All Java EE code is tested and validated for Java EE 7 and later versions.
Checkout our other articles