10 Essential Skills Every Java Developer Needs Today
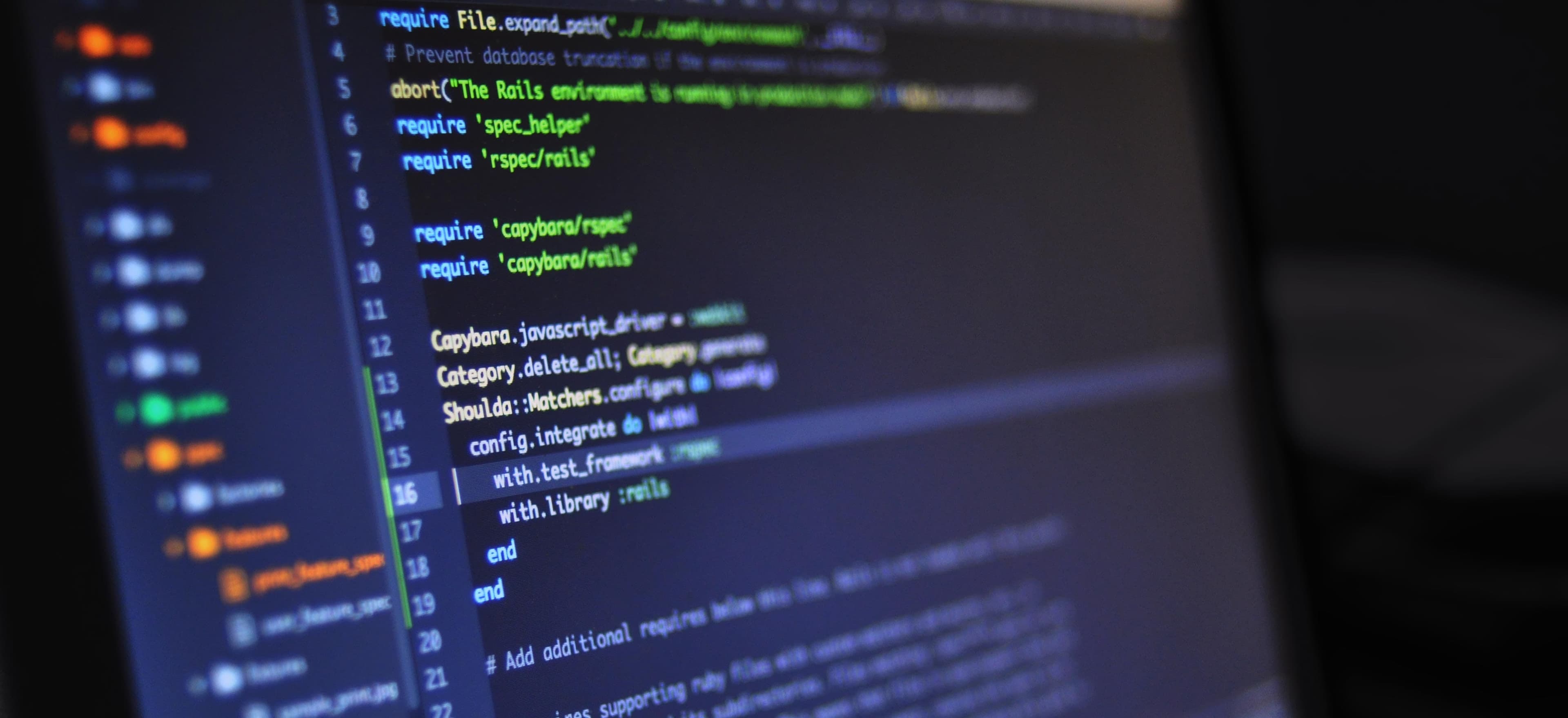
- Published on
10 Essential Skills Every Java Developer Needs Today
As a Java developer, it's crucial to stay up-to-date with the latest technologies, trends, and best practices. Whether you're a seasoned pro or new to Java development, honing these essential skills will not only enhance your expertise but also make you highly sought after in the competitive tech industry.
In this article, we'll explore the 10 essential skills every Java developer needs to excel in today's fast-paced and dynamic software development landscape.
1. Proficiency in Java Core
Mastering the fundamentals of Java is non-negotiable. From understanding the basic syntax and data types to advanced topics such as multithreading, generics, and exception handling, a strong command of Java's core concepts forms the backbone of your development journey.
Code Example:
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Understanding the 'why' behind Java's syntax and principles is as important as knowing how to use them effectively. Utilize resources like Oracle's Java Tutorials to deepen your understanding.
2. Object-Oriented Programming (OOP) Principles
Java is an object-oriented language, and a profound grasp of OOP principles like inheritance, polymorphism, encapsulation, and abstraction is paramount. These principles enable you to write modular, maintainable, and scalable code.
Code Example:
public class Animal {
String name;
public void makeSound() {
System.out.println("Some generic sound");
}
}
public class Dog extends Animal {
public void makeSound() {
System.out.println("Woof!");
}
}
Understanding OOP in Java can be a game-changer in designing elegant and efficient solutions. Revising the basics through platforms like Codecademy can solidify your understanding.
3. Proficiency in Java Frameworks
Mastery of popular Java frameworks like Spring, Hibernate, and Struts is essential for building enterprise-level applications. Understanding their intricacies, such as Spring's dependency injection and Hibernate's object-relational mapping, can significantly boost your productivity.
Code Example:
// Spring Dependency Injection
public class Car {
private Engine engine;
public Car(Engine engine) {
this.engine = engine;
}
}
In-depth knowledge of these frameworks allows you to harness their full potential and create robust, scalable applications. Exploring tutorials on platforms like Baeldung can provide comprehensive insights.
4. Database Management
Java developers often work with databases, so a strong understanding of database management systems (DBMS) and SQL is crucial. Familiarize yourself with database design, querying, and optimization to build efficient and scalable data solutions.
Code Example:
// JDBC Connection
Connection connection = DriverManager.getConnection("jdbc:mysql://localhost:3306/mydatabase", "username", "password");
Statement statement = connection.createStatement();
ResultSet resultSet = statement.executeQuery("SELECT * FROM users");
Solidifying your knowledge of database concepts and technologies like MySQL or PostgreSQL can elevate your ability to build data-centric applications. Platforms like Khan Academy offer insightful tutorials.
5. Proficiency in Testing
Being adept at unit testing with JUnit or TestNG is indispensable in ensuring the quality and reliability of your code. Understanding testing methodologies, mock objects, and test-driven development (TDD) empowers you to deliver high-quality, bug-free code.
Code Example:
// JUnit Test
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(3, 5);
assertEquals(8, result);
}
}
Embracing test-driven development and learning advanced testing techniques can significantly enhance the quality of your Java applications. Platforms like JUnit offer comprehensive documentation.
6. Understanding of Design Patterns
Familiarity with design patterns like Singleton, Factory, and Observer equips you with proven solutions to common development challenges. Applying these patterns enhances the flexibility, reusability, and maintainability of your codebase.
Code Example:
// Singleton Design Pattern
public class Singleton {
private static final Singleton INSTANCE = new Singleton();
private Singleton() {}
public static Singleton getInstance() {
return INSTANCE;
}
}
Mastering design patterns enables you to architect robust and scalable solutions, reducing redundancy and increasing code maintainability. Resources like Refactoring Guru provide comprehensive insights into various design patterns.
7. Knowledge of Build Automation Tools
Proficiency in build automation tools like Maven or Gradle streamlines the build process, dependency management, and project structuring. Understanding these tools empowers you to efficiently manage complex projects and their dependencies.
Code Example:
<!-- Maven Project Structure -->
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.example</groupId>
<artifactId>my-project</artifactId>
<version>1.0.0</version>
</project>
Utilizing build automation tools simplifies project management, enhances collaboration, and ensures the reproducibility of builds. Platforms like Maven offer comprehensive guides for getting started.
8. Proficiency in Version Control Systems
Mastery of version control systems, particularly Git, is indispensable in modern software development. Understanding branching strategies, pull requests, and merging workflows facilitates seamless collaboration and codebase management.
Code Example:
# Git Branching
git checkout -b feature/new-feature
git add .
git commit -m "Implement new feature"
git push origin feature/new-feature
A strong command of version control systems enables efficient collaboration, tracking of changes, and safeguarding against code loss. Platforms like Atlassian's Git tutorial provide comprehensive resources for mastering Git.
9. Knowledge of Microservices Architecture
In the era of distributed systems, understanding microservices architecture and technologies like Spring Boot and Docker is paramount. Mastery of containerization, service orchestration, and communication protocols empowers you to design scalable and resilient systems.
Code Example:
// Spring Boot Microservice
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users/{id}")
public User getUser(@PathVariable Long id) {
return userService.getUserById(id);
}
}
Embracing microservices architecture allows you to build modular, scalable, and independent components, facilitating agility and resilience in your applications. Platforms like Docker Documentation offer comprehensive resources for learning containerization.
10. Continuous Learning and Problem-Solving Skills
In the dynamic landscape of software development, continuous learning and effective problem-solving are indispensable. Staying updated with the latest technologies, trends, and best practices empowers you to adapt to evolving requirements and challenges.
Code Example:
// Effective Problem-Solving
public int findMax(int[] arr) {
int max = arr[0];
for (int i = 1; i < arr.length; i++) {
if (arr[i] > max) {
max = arr[i];
}
}
return max;
}
Cultivating a mindset of continuous learning and honing your problem-solving skills equips you to tackle complex challenges and innovate in the ever-evolving landscape of Java development. Platforms like HackerRank offer diverse challenges to sharpen your problem-solving skills.
Final Considerations
Navigating the rapidly evolving realm of Java development necessitates a holistic skill set that encompasses core Java principles, advanced frameworks, database management, testing, design patterns, and modern architectural paradigms. By mastering these essential skills and staying attuned to emerging trends, you'll position yourself as a highly proficient and sought-after Java developer in today's competitive tech industry.
Checkout our other articles