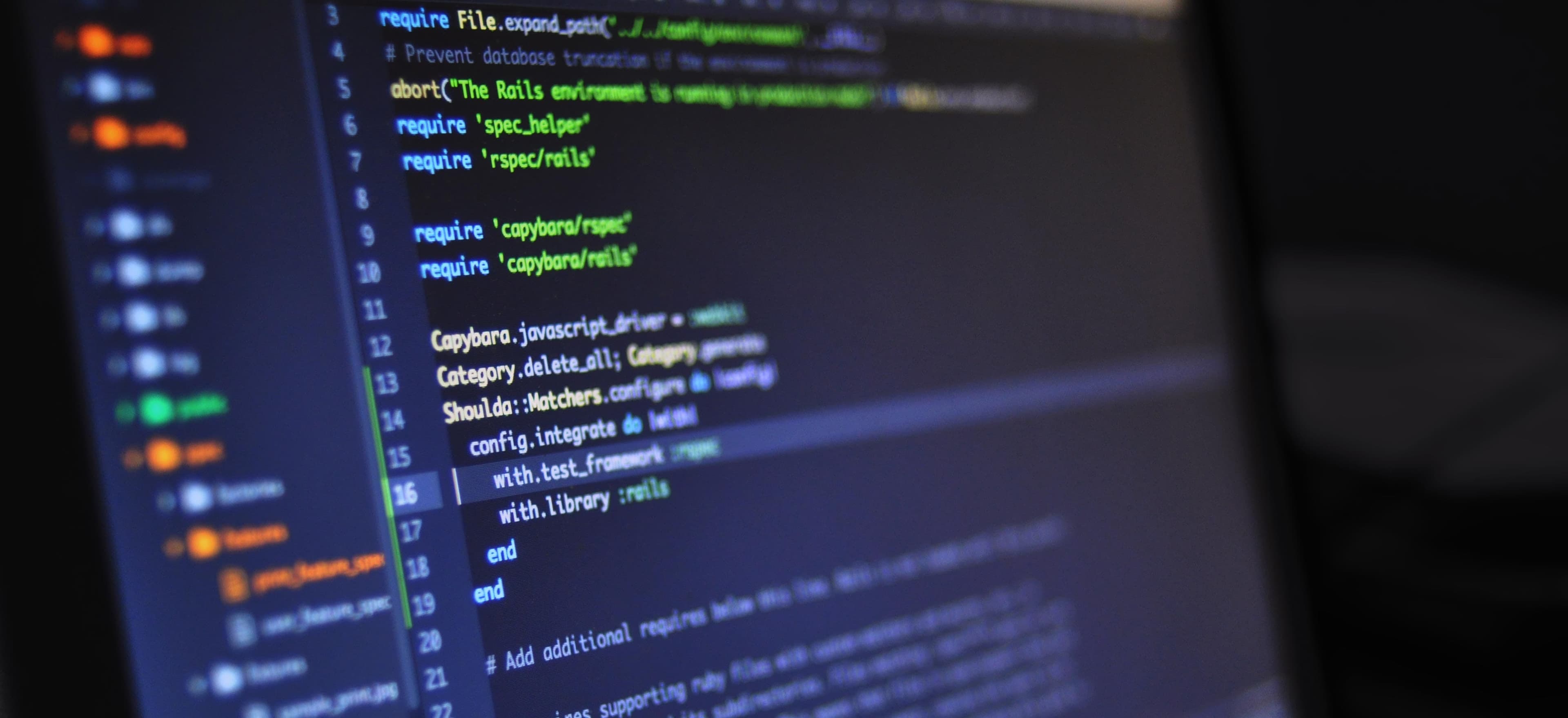
- Published on
Enhance Your Application with Custom Functions in Spring Repositories
When working with Spring repositories in a Java application, you may encounter scenarios where the built-in CRUD (Create, Read, Update, Delete) operations are not sufficient to fulfill your requirements. In such cases, adding custom functions to your Spring repositories can be an effective way to extend the repository capabilities and tailor them to your specific needs.
In this article, we'll explore the process of adding custom functions to Spring repositories and demonstrate how this can enhance the functionality and flexibility of your application.
Why Add Custom Functions to Spring Repositories?
Spring Data provides powerful repository support that allows you to perform common database operations without having to write boilerplate code. However, there are situations where you need to implement custom database queries or operations that are beyond the scope of the standard CRUD operations.
By adding custom functions to your Spring repositories, you can:
- Implement complex database queries that are not supported by the built-in repository methods.
- Encapsulate commonly used query logic within the repository interface, promoting reusability and maintainability.
- Leverage the benefits of Spring Data while catering to specific business requirements.
Now, let's delve into the process of adding custom functions to Spring repositories.
Defining a Custom Function in a Spring Repository
To add a custom function to a Spring repository, you can simply declare a method in the repository interface and annotate it with the @Query
annotation to specify the custom query.
Here's an example of how to define a custom function in a Spring repository interface:
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
import java.util.List;
public interface ProductRepository extends CrudRepository<Product, Long> {
@Query("SELECT p FROM Product p WHERE p.price > ?1")
List<Product> findProductsAbovePrice(double minPrice);
}
In this example, the ProductRepository
interface declares a custom function findProductsAbovePrice
using the @Query
annotation. The custom query retrieves all products with a price greater than the specified minimum price.
Understanding the @Query Annotation
The @Query
annotation in Spring Data repositories allows you to specify custom JPQL (Java Persistence Query Language) or native SQL queries to be executed when the method is called.
By using the @Query
annotation, you have the flexibility to define complex queries involving joins, aggregations, and other advanced SQL features directly within the repository interface, avoiding the need to scatter query logic throughout your codebase.
Additionally, the @Query
annotation supports named parameters, allowing you to reference method parameters in the query by name, enhancing the readability and maintainability of the custom function.
Using Named Parameters in Custom Functions
Named parameters in custom repository functions can greatly improve the clarity and maintainability of your code. Let's modify the previous example to utilize named parameters:
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
import org.springframework.data.repository.query.Param;
import java.util.List;
public interface ProductRepository extends CrudRepository<Product, Long> {
@Query("SELECT p FROM Product p WHERE p.price > :minPrice")
List<Product> findProductsAbovePrice(@Param("minPrice") double minPrice);
}
In this modified example, the :minPrice
named parameter is used in the JPQL query, and the @Param
annotation is added to the method parameter to map it to the named parameter in the query.
Using named parameters not only improves the readability of the query but also makes it easier to understand the relationship between the method parameters and the query parameters.
Custom Repository Functions with Native Queries
While JPQL is a powerful query language, there are scenarios where you may need to execute native SQL queries in your custom repository functions. Spring Data provides support for native queries through the nativeQuery
attribute of the @Query
annotation.
Let's consider an example of a custom function that executes a native SQL query:
import org.springframework.data.jpa.repository.Query;
import org.springframework.data.repository.CrudRepository;
import java.util.List;
public interface ProductRepository extends CrudRepository<Product, Long> {
@Query(value = "SELECT * FROM products WHERE price > ?1", nativeQuery = true)
List<Product> findProductsAbovePrice(double minPrice);
}
In this example, the nativeQuery = true
attribute indicates that the query is a native SQL query. By setting nativeQuery
to true
, you can execute native SQL queries within your custom repository functions, providing you with the flexibility to leverage native database features when necessary.
Testing Custom Repository Functions
Once you have defined custom functions in your Spring repositories, it's essential to write tests to verify their behavior and ensure they function as expected.
You can use the powerful testing capabilities provided by Spring Boot and JUnit to write comprehensive tests for your custom repository functions. By creating test cases that cover different scenarios and edge cases, you can validate the correctness of your custom repository functions and identify any potential issues early in the development process.
Bringing It All Together
Adding custom functions to Spring repositories empowers you to extend the capabilities of your data access layer, allowing you to implement complex queries and operations tailored to your application's specific requirements. By leveraging the features of Spring Data and JPQL or native SQL queries, you can encapsulate database logic within the repository interface, promoting reusability and maintainability.
When designing custom repository functions:
- Use the
@Query
annotation to specify custom JPQL or native SQL queries. - Utilize named parameters to enhance query readability and maintainability.
- Write comprehensive tests to validate the behavior of your custom repository functions.
By following these best practices, you can maximize the flexibility and power of your Spring repositories, leading to a more robust and efficient application architecture.
Implementing custom functions in Spring repositories not only unlocks advanced query capabilities but also streamlines your development process. If you’re ready to elevate your application's functionality and performance, it's time to explore the world of custom repository functions in Spring. Start enhancing your application today!
Checkout our other articles