Untangling Spring: WebAppInitializer vs. ApplicationContextInitializer
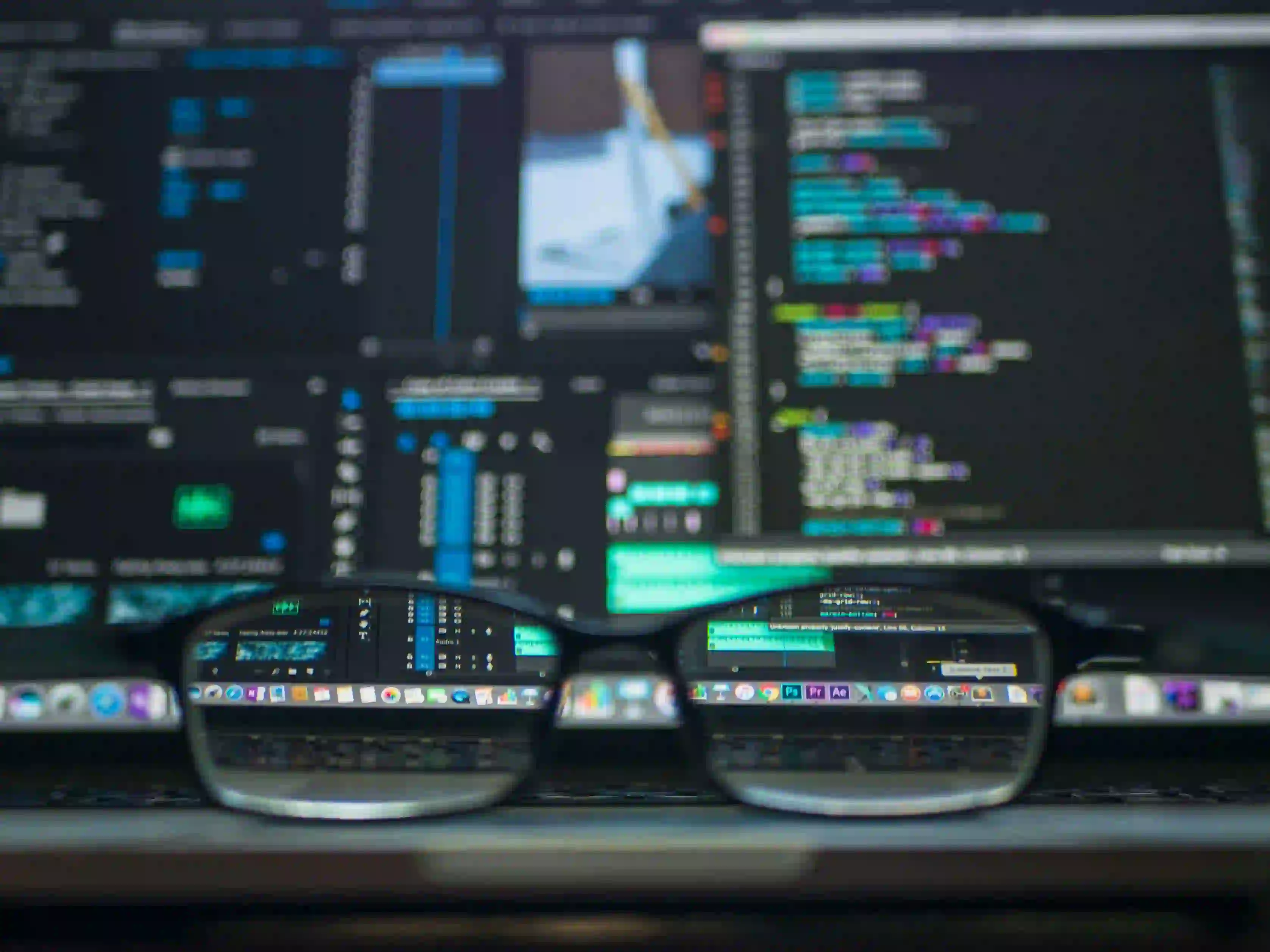
Demystifying Spring: A Comprehensive Guide to WebAppInitializer and ApplicationContextInitializer
When it comes to building robust and scalable Java web applications, Spring Framework is a go-to choice for developers. However, users often encounter confusion in distinguishing between two crucial components: WebAppInitializer
and ApplicationContextInitializer
. Let's delve into an in-depth analysis of these elements, unravel their significance, and understand how they facilitate the seamless functioning of Spring applications.
Understanding WebAppInitializer
In the realm of Spring and Java-based web applications, WebAppInitializer
plays a pivotal role in initializing the application's Servlet context programmatically. This feature was introduced in Servlet 3.0 as an enhancement to the previous web.xml deployment descriptor, allowing developers to configure the Servlet context without the necessity of a web.xml file.
The Why Behind WebAppInitializer
The primary advantage of WebAppInitializer
is its ability to provide a programmatic approach to the Servlet container's configuration, offering greater flexibility and enhanced control over the initialization process. By leveraging Java-based configuration classes, developers can easily customize the Servlet context setup, manage filters, listeners, and servlets, and thereby streamline the application's initialization process.
Let's dive into a concise yet powerful code snippet that exemplifies the implementation of a WebAppInitializer
.
import org.springframework.web.servlet.support.AbstractAnnotationConfigDispatcherServletInitializer;
public class MyWebAppInitializer extends AbstractAnnotationConfigDispatcherServletInitializer {
@Override
protected Class<?>[] getRootConfigClasses() {
return new Class[]{AppConfig.class};
}
@Override
protected Class<?>[] getServletConfigClasses() {
return null;
}
@Override
protected String[] getServletMappings() {
return new String[]{"/"};
}
}
In this code snippet, the class MyWebAppInitializer
extends AbstractAnnotationConfigDispatcherServletInitializer
, instigating a concise yet robust setup for the Spring-based application's Servlet context. The getRootConfigClasses
method references the AppConfig
class, designating it as the root context configuration class. This illustrates the elegance of WebAppInitializer
, providing a straightforward means of configuring the application's Servlet context.
Deciphering ApplicationContextInitializer
On the other hand, ApplicationContextInitializer
operates within the domain of Spring's ApplicationContext, allowing developers to hook into the application context's lifecycle and customize its behavior as it initializes. This feature proves immensely beneficial when the need arises to implement specific logic or perform custom operations during the application context's initialization phase.
The Essence of ApplicationContextInitializer
The crux lies in the capability of ApplicationContextInitializer
to enhance the application context initialization process by injecting custom logic, which may range from environment-specific configurations to dynamic profile activation, thus bolstering the application's extensibility and adaptability.
Let's glean insight from a succinct code excerpt that illuminates the utilization of ApplicationContextInitializer
.
import org.springframework.context.ApplicationContextInitializer;
import org.springframework.context.ConfigurableApplicationContext;
public class MyApplicationContextInitializer implements ApplicationContextInitializer<ConfigurableApplicationContext> {
@Override
public void initialize(ConfigurableApplicationContext applicationContext) {
// Custom initialization logic can be performed here
}
}
In this snippet, the class MyApplicationContextInitializer
implements ApplicationContextInitializer
and overrides the initialize
method, signifying the inclusion of custom initialization logic within the application context setup. This exemplifies the efficacy of ApplicationContextInitializer
in tailoring the initialization process to accommodate specific requirements.
Embracing Harmony: Coalescing WebAppInitializer and ApplicationContextInitializer
Now, the question arises: how do these two entities harmonize within a Spring-based web application?
The answer lies in their collective potential to fortify the application's initialization and configuration phases. Whereas WebAppInitializer
excels in orchestrating the Servlet context's setup, ApplicationContextInitializer
complements this by empowering developers to wield greater influence over the application context's initialization.
By integrating these components cohesively, developers can capitalize on the amalgamated strength of both WebAppInitializer
and ApplicationContextInitializer
, thereby fortifying the underpinnings of the Spring application and augmenting its resilience and extensibility.
The Verdict
In the realm of Spring Framework, understanding and harnessing the capabilities of WebAppInitializer
and ApplicationContextInitializer
is pivotal to crafting robust, adaptable, and well-structured web applications. WebAppInitializer
empowers developers to programmatically configure the Servlet context, while ApplicationContextInitializer
augments this by enabling custom logic injection into the application context's initialization process.
Ultimately, a judicious amalgamation of these two entities culminates in a harmonious synergy, enriching the application's initialization and setup phases and underpinning it with a sturdy foundation.
In conclusion, demystifying WebAppInitializer
and ApplicationContextInitializer
equips developers with the acumen to leverage these components efficaciously, thereby sculpting resilient and agile Spring-based web applications that resonate with proficiency and finesse.
References:
Start incorporating these components into your Spring applications and revel in the amplified control and customization they deliver!