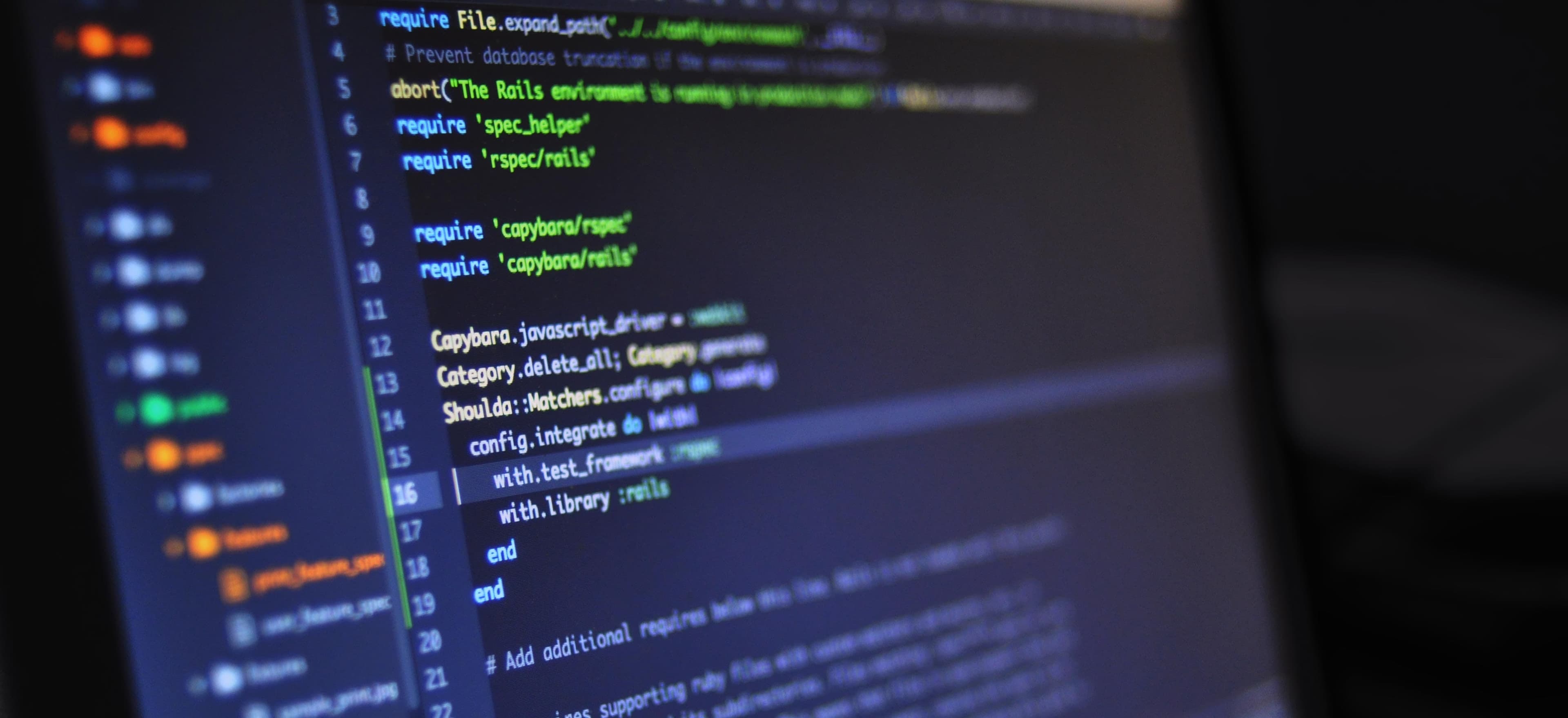
- Published on
Reviving Android ActionBar: Solving Dropdown Woes
The Android ActionBar has been a cornerstone of the Android user interface (UI) framework since its introduction, providing a convenient space for navigation and action items. Over time, the ActionBar has evolved in response to changing design trends and user expectations. In this article, we will explore the significance of the ActionBar, share best practices for its implementation, and offer solutions for common issues related to dropdown menus. Whether you are a novice developer or an experienced Android enthusiast, mastering the customization of ActionBar dropdowns can elevate the user experience of your applications.
Understanding the ActionBar
History and Evolution
The ActionBar made its debut in Android 3.0 (Honeycomb) as a replacement for the older title bar and was designed to accommodate a more expansive and functional UI. Over the years, it has undergone significant changes, such as the introduction of the Toolbar, which made it more flexible and customizable. These improvements have empowered developers to create more visually appealing and user-friendly applications.
Why It's Still Relevant
In the modern Android ecosystem, the ActionBar remains relevant due to its ability to enhance user experience and provide intuitive navigation. By integrating a cohesive and efficient navigation system, applications can guide users seamlessly through different sections and features. Moreover, its customization potential allows developers to maintain consistent branding and aesthetics throughout their applications.
Common Problems with Dropdown Menus in ActionBar
Overview of Issues
Dropdown menus in the ActionBar pose unique challenges for developers. These include ensuring a consistent and attractive visual style, managing user interactions, and maintaining compatibility across various Android versions and device sizes.
Case Study: Common Pitfalls
Consider a scenario where a developer attempts to implement a dropdown menu within an application's ActionBar. However, the dropdown items are unresponsive to user interactions, leading to a frustrating user experience. This case study highlights the importance of addressing common pitfalls associated with dropdown menus to ensure a seamless interaction.
Solving Dropdown Woes in ActionBar
Step-by-Step Guide to Elegant Dropdowns
To address these challenges, let's explore a step-by-step guide to creating and customizing dropdown menus within the ActionBar:
Step 1: Initializing the Dropdown
To initialize a dropdown menu in the ActionBar, you'll start by defining the items to be displayed in the menu. This involves creating a menu resource file and inflating it in the ActionBar.
@Override
public boolean onCreateOptionsMenu(Menu menu) {
getMenuInflater().inflate(R.menu.menu_main, menu);
return true;
}
Step 2: Customizing the Appearance
To customize the appearance of the dropdown menu, you can modify the visual attributes such as text color, background color, and item spacing in the menu resource file.
<menu xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:app="http://schemas.android.com/apk/res-auto">
<item
android:id="@+id/item1"
android:title="Item 1"
app:showAsAction="never" />
<item
android:id="@+id/item2"
android:title="Item 2"
app:showAsAction="never" />
<!-- Add more items as needed -->
</menu>
Step 3: Handling Item Selection Events
To respond to user interactions with the dropdown menu items, you'll implement a listener to capture the selected item and perform the corresponding action.
@Override
public boolean onOptionsItemSelected(MenuItem item) {
switch (item.getItemId()) {
case R.id.item1:
// Handle item 1 selection
return true;
case R.id.item2:
// Handle item 2 selection
return true;
default:
return super.onOptionsItemSelected(item);
}
}
Best Practices for Implementation
In addition to the step-by-step guide, here are some best practices for implementing dropdown menus in the ActionBar:
- Accessibility: Ensure that the dropdown menu is accessible to all users, including those with visual impairments, by providing descriptive text and using appropriate touch target sizes.
- Responsiveness: Test the dropdown menu across different screen sizes and orientations to guarantee a consistent and responsive experience for all users.
- Design Language: Adhere to the overall design language of the application to maintain a coherent and polished UI.
Advanced Tips and Tricks
Customizing Appearance
For advanced customization of the appearance of ActionBar dropdown menus, you can leverage the following techniques:
Using Custom Themes
By applying custom themes to the dropdown menu, you can achieve a consistent and branded look that aligns with the overall aesthetic of the application.
<style name="AppTheme" parent="Theme.AppCompat.Light.DarkActionBar">
<item name="actionBarTheme">@style/CustomActionBarTheme</item>
</style>
<style name="CustomActionBarTheme" parent="ThemeOverlay.AppCompat.ActionBar">
<item name="android:textColorPrimary">@color/customTextColor</item>
<item name="android:background">@drawable/customBackground</item>
<!-- Add more customizations as needed -->
</style>
Changing Item Highlight Colors
By modifying the item highlight colors, you can draw attention to selected items within the dropdown menu.
<selector xmlns:android="http://schemas.android.com/apk/res/android">
<item android:state_pressed="true" android:drawable="@color/pressedColor" />
<item android:state_focused="true" android:drawable="@color/focusedColor" />
<item android:drawable="@color/defaultColor" />
</selector>
Using Custom Fonts
Integrating custom fonts into the dropdown menu items can enhance the visual appeal and reinforce the branding of the application.
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Custom font"
android:textColor="@color/customTextColor"
android:fontFamily="@font/customFont" />
Streamlining User Experience
To optimize the user experience of ActionBar dropdown menus, consider the following strategies:
- Icons: Incorporate icons alongside menu items to visually communicate their functions and improve navigation cues.
- Search Functionality: Integrate search functionality within the dropdown menu to enable users to access content swiftly.
- UI Components Integration: Explore integration with other UI components, such as RecyclerView or CardView, to create a seamlessly integrated interface.
Real-world Applications
Showcasing Examples
Two notable examples of successful implementation of customized ActionBar dropdown menus can be observed in the applications WhatsApp and Airbnb. Both applications have seamlessly integrated dropdown menus within their ActionBar, enhancing the user experience and strengthening their brand identity.
Lessons Learned
By examining these examples, we can learn the importance of meticulously customizing the ActionBar dropdown menus to align with the overall aesthetics and usability of the application. Attention to detail and thoughtful customization contribute significantly to a compelling user interface.
The Bottom Line
In conclusion, the Android ActionBar remains a vital component of modern Android applications, offering a platform for seamless navigation and interaction. By mastering the customization of ActionBar dropdown menus, developers can elevate their applications to stand out in the competitive app marketplace. Embrace the creative possibilities enabled by ActionBar customization and embark on a journey to create intuitive and visually stunning user interfaces.
Call to Action
We encourage you to share your experiences and tips regarding ActionBar customization in the comments section below. Explore further resources and tutorials to continue expanding your knowledge and skills in Android development.
Additional Resources
For further exploration of the Android ActionBar and its customization, we recommend the following resources:
- Official Android Developer Documentation on ActionBar
- CodePath Android Cliffnotes: ActionBar
- Stack Overflow: Android ActionBar Questions
In this article, we've delved into the pertinent aspects of the Android ActionBar and tackled the intricacies of customizing dropdown menus within it. With the provided step-by-step guide, best practices, advanced tips, and real-world examples, developers can equip themselves with the necessary knowledge to create visually appealing and functional ActionBar dropdown menus. Whether you're an aspiring Android developer or a seasoned professional, mastering this skill will undoubtedly enrich the user experience of your applications.
Checkout our other articles