Unlocking Secrets: Mastering Java Identity API Challenges
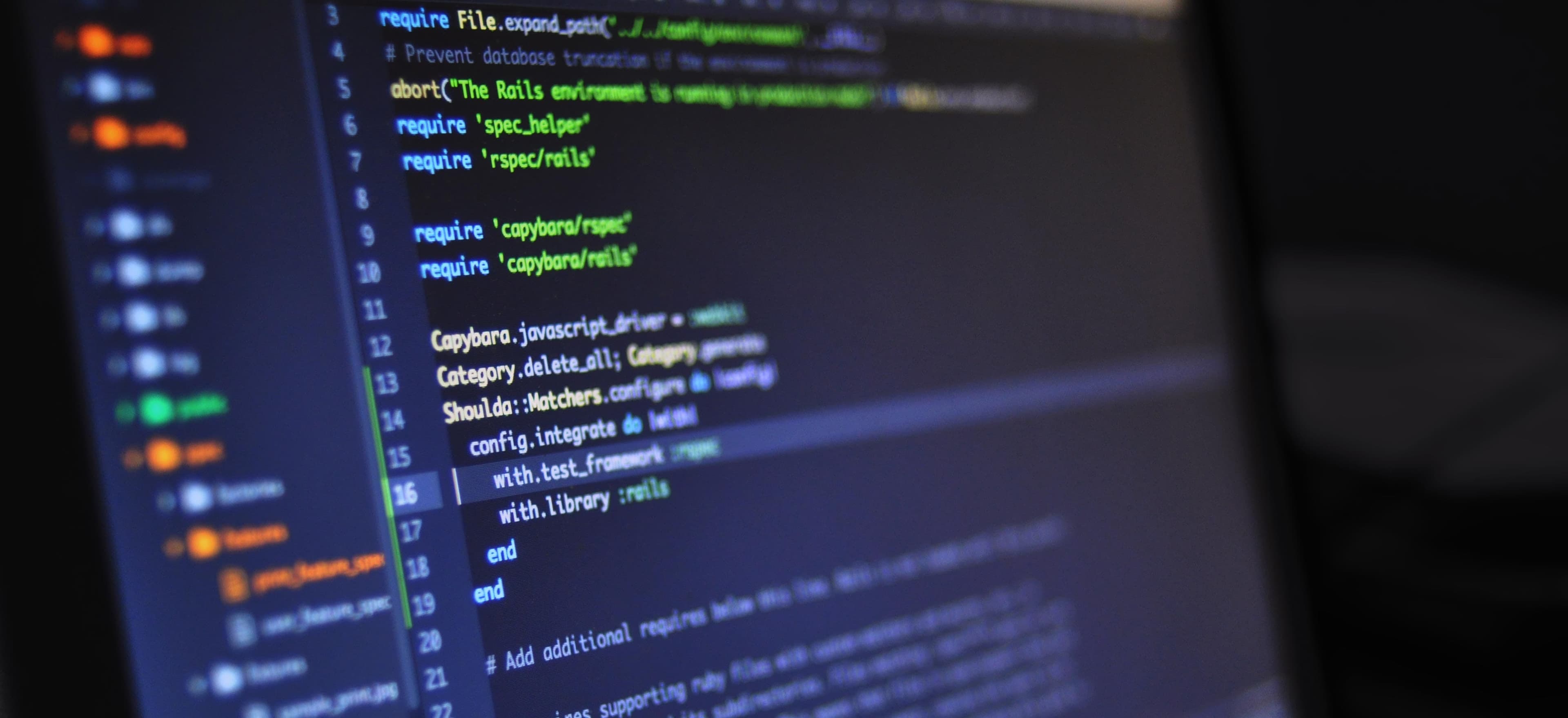
- Published on
Unlocking Secrets: Mastering Java Identity API Challenges
Java has long stood as a bastion of reliability and versatility in the programming world. Its ability to evolve while maintaining backward compatibility ensures it remains relevant in today's rapidly advancing tech landscape. One intriguing feature in Java's arsenal is the Identity API, a crucial aspect for developers dealing with security, authentication, and authorization processes. This post delves deep into the nuances of mastering Java Identity API challenges, navigating its complexities, and leveraging its full potential to secure applications against the backdrop of ever-evolving cyber threats.
Understanding Java Identity API
Before we dive into the intricacies of the Java Identity API, let's establish a foundational understanding of what it encompasses. At its core, the Identity API in Java provides a framework for representing and manipulating identity-related information: users, groups, and roles. This framework serves as a cornerstone for developing secure Java applications, ensuring that access control and user management are handled efficiently and effectively.
Why Is It Important?
In a digital era where security breaches are commonplace, having robust identity management and authentication mechanisms is non-negotiable. The Java Identity API aids developers in creating systems that can precisely determine who the user is (authentication) and what resources the user can access (authorization), making it an indispensable part of securing Java applications.
Challenges in Mastering Java Identity API
While the benefits of utilizing the Java Identity API are clear, mastering it comes with its set of challenges:
- Complex Configuration: Setting up the Identity API can be daunting due to its complex configurations.
- Security Concerns: With great power comes great responsibility. Incorrect implementation can lead to vulnerabilities.
- Performance Overheads: Ensuring that the security layer does not significantly impact application performance is crucial.
How to Overcome These Challenges
Overcoming the challenges associated with the Java Identity API requires a combination of understanding best practices, leveraging existing solutions, and continuously learning. Here are some strategies to tackle these hurdles efficiently:
Simplify Configuration with Spring Security
One way to simplify the configuration and implementation of the Java Identity API is by using Spring Security. Spring Security provides abstractions over the Java Security API, simplifying security configurations while offering robust authentication and authorization features.
For example, configuring in-memory authentication with Spring Security can be as simple as:
@EnableWebSecurity
public class WebSecurityConfig extends WebSecurityConfigurerAdapter {
@Override
protected void configure(AuthenticationManagerBuilder auth) throws Exception {
auth.inMemoryAuthentication()
.withUser("user").password(passwordEncoder().encode("password")).roles("USER")
.and()
.withUser("admin").password(passwordEncoder().encode("admin")).roles("USER", "ADMIN");
}
@Bean
public PasswordEncoder passwordEncoder() {
return new BCryptPasswordEncoder();
}
}
In this example, we've set up in-memory authentication with two users (user
and admin
) using the BCryptPasswordEncoder
for password encoding, showcasing the simplicity of Spring Security configurations.
Addressing Security Concerns
To address security concerns effectively, adhere to best practices such as:
- Principle of Least Privilege: Assign users the minimum permissions required to perform their duties.
- Regular Security Audits: Regularly audit your security configurations and codebase for vulnerabilities.
- Use of Secure Coding Practices: Follow secure coding guidelines, such as those outlined by OWASP (Open Web Application Security Project).
Optimizing Performance
To mitigate performance overheads, consider the following:
- Caching: Implement caching strategies for frequently accessed identity data.
- Lazy Loading: Employ lazy loading techniques for identity data to reduce the initial load time.
- Asynchronous Processing: Use asynchronous processing for heavy identity management tasks to enhance application responsiveness.
Leveraging Java Identity API in Your Projects
Incorporating the Java Identity API into your projects allows you to build secure and efficient Java applications capable of handling complex identity management requirements. Here are steps to get started:
- Assess Your Requirements: Understand the specific identity management needs of your application.
- Choose the Right Tools: Based on your requirements, decide whether to use the Java Identity API directly, leverage frameworks like Spring Security, or both.
- Implement Best Practices: Apply the best practices mentioned above throughout your development process.
- Test Thoroughly: Ensure your implementation is secure and efficient through rigorous testing.
A Final Look
Mastering the Java Identity API is a journey that demands a deep understanding of its complexities, a commitment to security, and a focus on performance. By tackling the challenges head-on, leveraging frameworks like Spring Security, and adhering to best practices, developers can unlock the full potential of the Java Identity API, crafting secure and robust Java applications. As we navigate the ever-evolving landscape of digital security, staying informed and adaptable is key. For more insights into leveraging the Java ecosystem for building secure applications, consider exploring resources such as the official Java documentation.
With persistence and continuous learning, unlocking the secrets of the Java Identity API not only enhances your skillset but also elevates the security and efficiency of your Java applications. Dive into the world of Java Identity API and embrace the challenges as stepping stones to mastering Java security.
Checkout our other articles