Solving Slow Queries: Speed Up with ObjectBox Database!
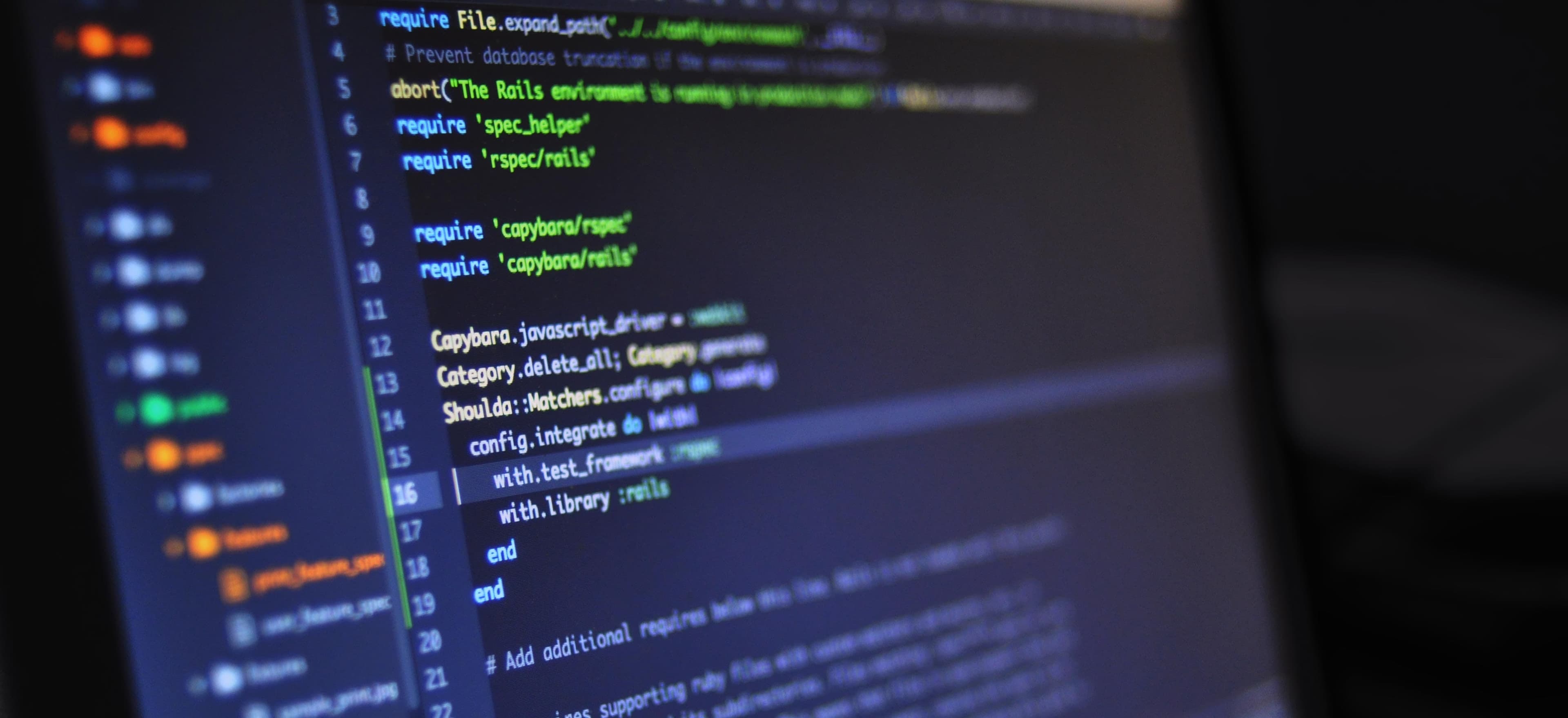
- Published on
How to Solve Slow Queries by Speeding Up with ObjectBox Database
In the world of Java programming, database performance is a critical factor that can greatly impact the overall efficiency of an application. Slow queries can be a common issue when working with traditional relational databases, leading to frustrating delays in retrieving and manipulating data. Fortunately, there are alternative solutions that can address this problem, one of which is the ObjectBox database.
Understanding the Impact of Slow Queries
Slow queries can significantly hinder the performance of an application, leading to decreased user satisfaction and potentially causing scalability issues. The root cause of slow queries often lies in the design and structure of the database, particularly in scenarios where complex relationships and large datasets are involved. In traditional relational databases, queries can become slow as the volume of data grows, impacting the overall responsiveness of the application.
Introducing ObjectBox: A Swift Solution
ObjectBox is a high-performance, object-oriented database designed specifically for mobile and IoT devices, and it can also be utilized in Java applications. By leveraging ObjectBox, developers can significantly improve the speed and efficiency of database operations, particularly when dealing with complex data queries.
Getting Started with ObjectBox
To begin using ObjectBox in a Java project, you first need to integrate the ObjectBox library into your application. Let's take a look at a simple example of how to set up ObjectBox in a Java project.
First, add the ObjectBox Gradle plugin to the project's build.gradle
file:
buildscript {
repositories {
maven {
url "https://objectbox.net/dev/java"
}
}
dependencies {
classpath 'io.objectbox:objectbox-gradle-plugin:3.1.0'
}
}
Then, apply the ObjectBox Gradle plugin and add the ObjectBox dependency to the app's build.gradle
file:
apply plugin: 'io.objectbox'
dependencies {
implementation 'io.objectbox:objectbox-android:3.1.0'
}
By following these steps, you can integrate ObjectBox into your Java application and start leveraging its capabilities to improve database performance.
Optimizing Queries with ObjectBox
One of the key advantages of ObjectBox is its ability to optimize queries for speed and efficiency. Unlike traditional relational databases, ObjectBox utilizes an object-oriented approach to data storage and retrieval, which can lead to significant performance improvements. Let's explore how ObjectBox achieves this optimization.
Entity Object Modeling
In ObjectBox, you define data models using entity classes, each of which represents a specific type of entity in your database. These entity classes encapsulate the structure and behavior of the data they represent, allowing for efficient storage and retrieval.
Here's an example of an entity class in ObjectBox:
@Entity
public class User {
@Id
long id;
String name;
int age;
// Getters and setters
}
By modeling entities in this way, ObjectBox can optimize data storage and retrieval based on the specific structure of the entity, leading to faster query performance.
ObjectBox Query Language (OQL)
ObjectBox provides its own query language, known as ObjectBox Query Language (OQL), which enables developers to construct efficient and precise queries for retrieving data from the database. OQL is designed to be intuitive and expressive, allowing for complex query operations while maintaining high performance.
Let's take a look at an example of an OQL query in ObjectBox:
Box<User> userBox = store.boxFor(User.class);
List<User> users = userBox.query()
.startsWith(User_.name, "J")
.and()
.greater(User_.age, 25)
.build()
.find();
In this example, we're using OQL to construct a query that retrieves users whose names start with "J" and whose age is greater than 25. ObjectBox's query optimization ensures that this operation is executed with minimal overhead, resulting in improved query performance.
Key Takeaways
ObjectBox presents a compelling solution for addressing slow queries and improving database performance in Java applications. By embracing its object-oriented approach to data storage and manipulation, developers can achieve significant speed and efficiency improvements in database operations. If you're facing performance challenges with traditional relational databases, considering ObjectBox as an alternative can lead to a transformative impact on the overall responsiveness and scalability of your application.
By integrating ObjectBox and leveraging its query optimization capabilities, Java developers can effectively tackle the challenges associated with slow queries and propel their applications to new heights of performance and efficiency.
In conclusion, if you want to take your Java application to the next level by optimizing database performance, ObjectBox is certainly a powerful and compelling option worth exploring.
For more information about ObjectBox and its features, you can visit the official website here and the documentation here.
Don't let slow queries hold your application back – embrace the speed and efficiency of ObjectBox today!