Avoiding MVC Pitfalls in Game Design: Expert Tips
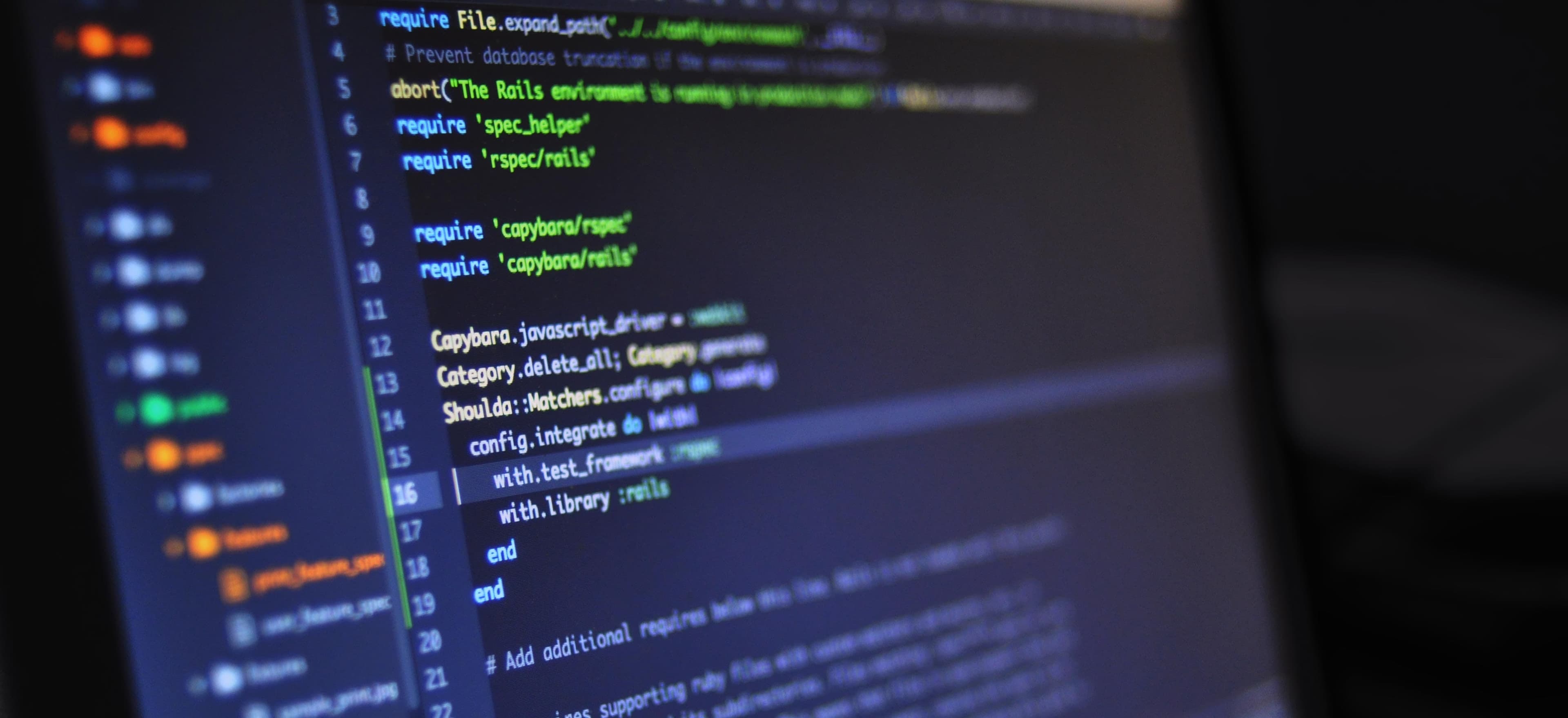
- Published on
Avoiding MVC (Model-View-Controller) Pitfalls in Java Game Design: Expert Tips
In the dynamic realm of game development, adopting a robust architectural pattern is crucial for fostering a well-organized and scalable project structure. The Model-View-Controller (MVC) pattern has emerged as a prominent paradigm in game design, particularly for developers leveraging the robustness of Java. However, navigating the MVC architecture while avoiding common pitfalls can be challenging, especially for burgeoning developers aiming to elevate their game designs to professional standards.
This blog post delves into the intricacies of utilizing the MVC pattern in Java game design, highlighting how to sidestep common mistakes and optimize your development process for better performance, maintainability, and scalability. Whether you're a novice venturing into game development or an experienced Java programmer keen on refining your MVC application, this guide offers expert insights to enhance your project's architecture.
Understanding MVC in Java Game Design
Before we dive into the pitfalls, let's briefly recap the MVC architecture. MVC separates the application into three interconnected components:
- Model: Manages the data and business logic.
- View: Handles the display and user interface.
- Controller: Acts as an intermediary that manages the communication between the model and the view.
For game development, this separation helps organize game components, making code more reusable and easier to manage. However, misapplying MVC can lead to performance bottlenecks, convoluted code, and scalability issues.
Pitfall #1: Overusing Observers
In an attempt to keep the model and view synchronized, developers often employ observers (listeners) excessively. While observers are essential in the MVC pattern, overuse can lead to performance degradation, especially in games where the state changes frequently.
Better Approach:
Optimize the use of observers by batching updates or using event aggregation. For instance, instead of updating the view on every minor model change, aggregate those changes and update the view at once.
public void updateViewBatch(List<ModelChange> changes) {
// Aggregate changes and apply to view
for (ModelChange change : changes) {
// Handle change
}
// Refresh view after all changes are applied
view.refresh();
}
Pitfall #2: Blurring Lines Between MVC Components
A common mistake is not maintaining a clear separation of concerns, leading to intertwined model, view, and controller code. This not only complicates the codebase but also hampers reusability and testing.
Better Approach:
Strictly adhere to the MVC principles. Ensure that each component only interacts with its direct responsibility. Use interfaces and dependency injection to further decouple components.
public interface GameModel {
// Model methods
}
public class GameModelImpl implements GameModel {
// Implementation
}
public class GameController {
private GameModel model;
private GameView view;
public GameController(GameModel model, GameView view) {
this.model = model;
this.view = view;
// Initialize interaction between model and view
}
}
Pitfall #3: Neglecting the Game Loop
MVC is about interaction, but game design also relies heavily on the game loop (updating game state and rendering). Integrating the game loop within MVC without jeopardizing the pattern's integrity can be tricky.
Better Approach:
Implement the game loop within the controller while keeping game logic (model) and rendering (view) separate. The controller should manage the timing and sequence of updates and renders.
public void startGameLoop() {
final long NANOSECONDS_PER_UPDATE = 1000000000 / 60; // 60 FPS
long lastUpdateTime = System.nanoTime();
while (gameRunning) {
long now = System.nanoTime();
if (now - lastUpdateTime >= NANOSECONDS_PER_UPDATE) {
model.updateGameState();
view.render();
lastUpdateTime = now;
}
}
}
Additional Expert Tips
- Performance Tuning: Investigate Java performance tuning options, such as Just-In-Time (JIT) compilation and garbage collection optimizations, to mitigate any MVC-related overhead. The official Java Performance Documentation is a good place to start.
- Profiling Tools: Leverage Java profiling tools to identify bottlenecks in your MVC game architecture. Tools like VisualVM can provide invaluable insights into memory usage and method performance.
- Continuous Refactoring: As your game evolves, so should your implementation of MVC. Continuously refactor your code to adapt to new requirements while maintaining a clear separation of concerns.
To Wrap Things Up
The MVC architecture holds great promise for Java game development, offering a structured approach to organizing code and facilitating scalability. However, its effective implementation demands attentiveness to common pitfalls such as overusing observers, blurring component responsibilities, and mismanaging the game loop. By following the strategies outlined in this post, game developers can leverage MVC to its full potential, resulting in cleaner, more maintainable, and scalable game projects.
Mastering MVC in game design is an ongoing journey. As technologies and methodologies evolve, so will the best practices for MVC in Java game development. Stay engaged with the community and continuously seek out new insights and strategies to refine your skill set.
Remember, the ultimate goal of adopting MVC or any architectural pattern is to enhance the maintainability, scalability, and performance of your game. With diligent application and continuous learning, the MVC pattern will become an invaluable tool in your Java game development arsenal.
For more insights into Java game development and architectural patterns, stay tuned to this blog. Happy coding!
Checkout our other articles