Master SSL: Custom TrustManagers for Each URL Connection
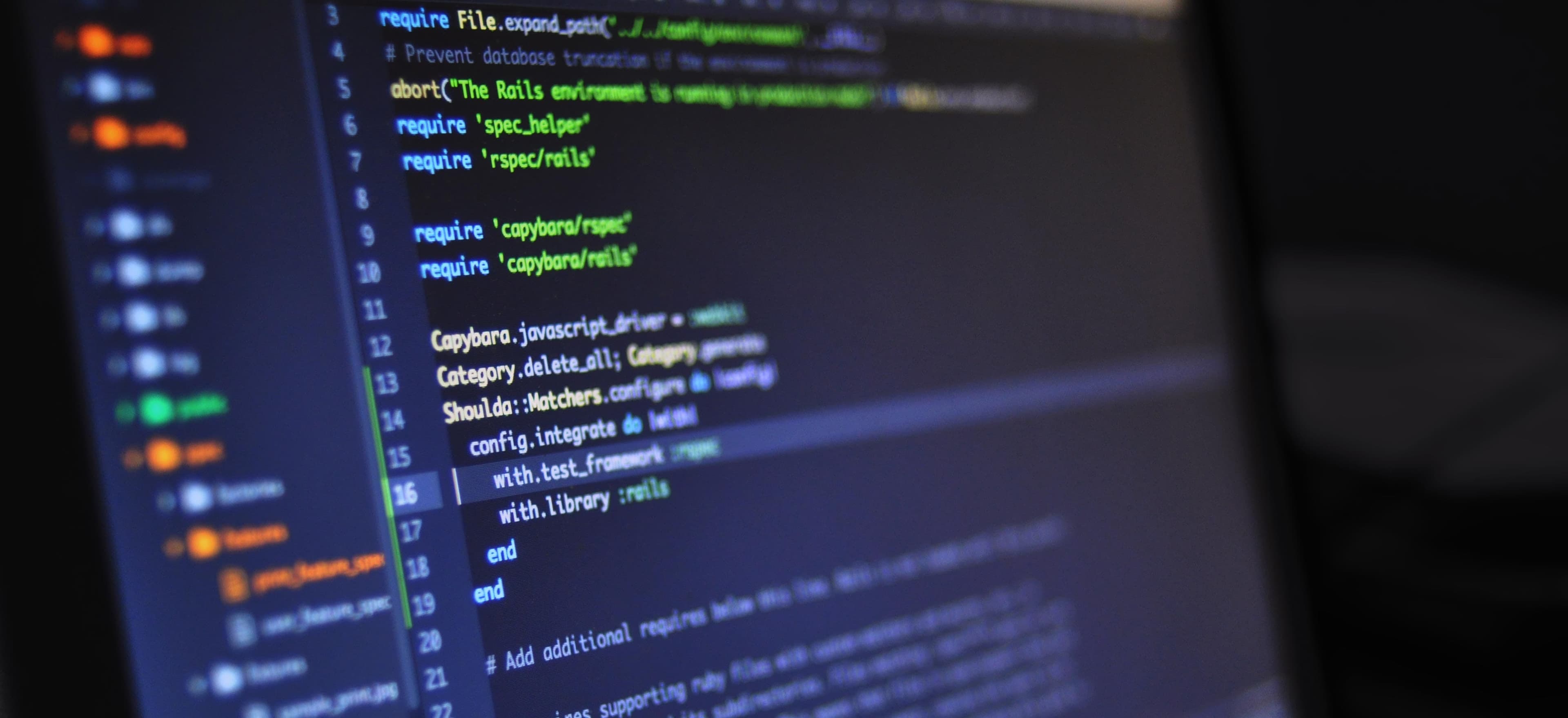
- Published on
Master SSL: Custom TrustManagers for Each URL Connection
In the world of Java, SSL (Secure Sockets Layer) plays a crucial role in securing network communication. When working with SSL in Java, you might encounter scenarios where you need to customize the trust management for each URL connection. This can be achieved by creating custom TrustManagers. In this article, we will explore how to master SSL by creating custom TrustManagers for each URL connection in Java.
Understanding TrustManagers
In Java, TrustManager is responsible for determining whether the remote server's certificate should be trusted. If you want to have fine-grained control over the trust management process for different URL connections, creating custom TrustManagers is the way to go.
The Scenario
Let's consider a scenario where you have multiple URL connections in your application, and you want to use different trust management policies for each connection. One connection might require strict certificate validation, while another might need to trust a self-signed certificate. To achieve this, we'll create custom TrustManagers tailored to the specific requirements of each URL connection.
Creating Custom TrustManagers
To create a custom TrustManager, you'll need to implement the X509TrustManager
interface, which is used for managing X.509 certificates. The X509TrustManager
interface provides three essential methods:
void checkClientTrusted(X509Certificate[] chain, String authType)
void checkServerTrusted(X509Certificate[] chain, String authType)
X509Certificate[] getAcceptedIssuers()
These methods allow you to define how certificates are trusted for a specific URL connection.
Example of a Custom TrustManager
Let's create a custom TrustManager that bypasses certificate validation for a specific URL connection. This can be useful when dealing with self-signed certificates or when you need to explicitly trust a particular certificate.
import java.security.cert.X509Certificate;
import javax.net.ssl.X509TrustManager;
import java.security.cert.CertificateException;
public class BypassCertValidationTrustManager implements X509TrustManager {
@Override
public void checkClientTrusted(X509Certificate[] chain, String authType) throws CertificateException {
// Implement client certificate validation logic if needed
}
@Override
public void checkServerTrusted(X509Certificate[] chain, String authType) throws CertificateException {
// Bypass certificate validation
}
@Override
public X509Certificate[] getAcceptedIssuers() {
return new X509Certificate[0];
}
}
In this example, the BypassCertValidationTrustManager
bypasses certificate validation by allowing all server certificates to be trusted, regardless of their authenticity. This is a simplified demonstration, but in a real-world scenario, you should carefully consider the implications of bypassing certificate validation.
Applying Custom TrustManagers to URL Connections
Once you've created custom TrustManagers, the next step is to apply them to the corresponding URL connections. This involves setting the SSL socket factory for each connection to use the custom TrustManager.
Applying Custom TrustManager to a URL Connection
import java.net.URL;
import javax.net.ssl.HttpsURLConnection;
import java.io.InputStream;
import java.security.KeyStore;
import javax.net.ssl.SSLContext;
public class CustomTrustManagerExample {
public static void main(String[] args) throws Exception {
// Create custom TrustManager
BypassCertValidationTrustManager trustManager = new BypassCertValidationTrustManager();
// Create SSL context
SSLContext sslContext = SSLContext.getInstance("TLS");
sslContext.init(null, new TrustManager[] {trustManager}, null);
// Set custom SSL context for the URL connection
URL url = new URL("https://example.com");
HttpsURLConnection connection = (HttpsURLConnection) url.openConnection();
connection.setSSLSocketFactory(sslContext.getSocketFactory());
// Perform connection and read response
InputStream input = connection.getInputStream();
// Read response...
}
}
In this example, we create an instance of BypassCertValidationTrustManager
and use it to initialize an SSL context. We then set the SSL socket factory of the HttpsURLConnection
to use our custom SSL context. This ensures that the specific URL connection will use the custom TrustManager with the defined trust management policy.
Closing the Chapter
In this article, we've explored the concept of creating custom TrustManagers for each URL connection in Java. By understanding TrustManagers and implementing custom TrustManagers tailored to specific requirements, you can have fine-grained control over the trust management process for SSL connections in your Java applications. This approach allows you to enforce different trust policies for different URL connections, enhancing the security and flexibility of your network communication.
Mastering SSL and custom TrustManagers empowers you to handle diverse SSL scenarios with confidence, ensuring secure and reliable communication in your Java applications.
Now that you've learned how to create custom TrustManagers for URL connections in Java, you can explore more advanced topics such as mutual SSL authentication, dynamic trust management based on runtime conditions, and integrating with certificate authorities for certificate chain validation.
For further reading, you can delve into the official Java Documentation to deepen your understanding of TrustManagers and SSL in Java.
With the knowledge gained from this article, you are well-equipped to tackle SSL challenges and elevate the security posture of your Java applications.