Tackling Complexity: Mastering State Design Pattern in Java
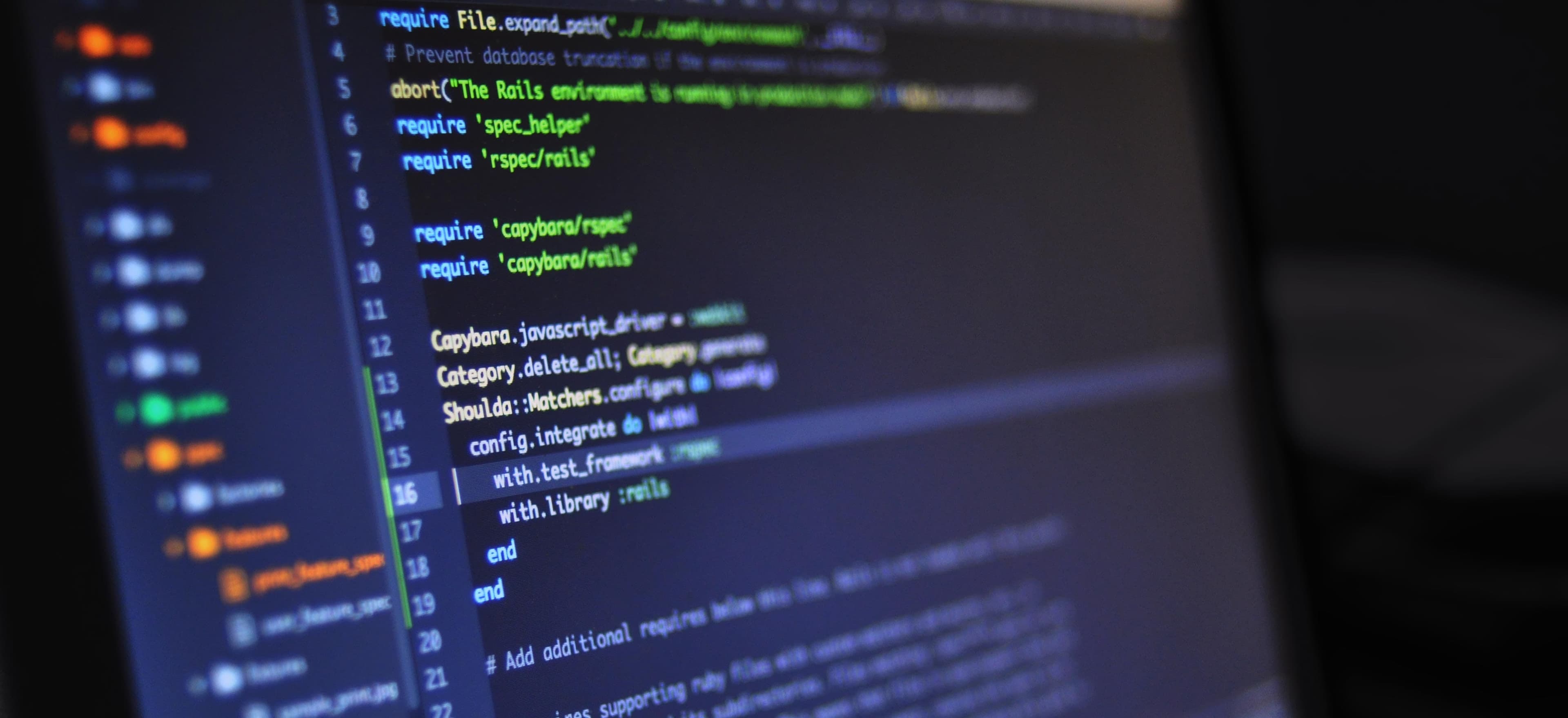
- Published on
Tackling Complexity: Mastering State Design Pattern in Java
In the world of software development, managing complexity is one of the core challenges. As applications grow in size and functionality, the complexity of managing state transitions becomes increasingly daunting. This is where the State design pattern comes to the rescue. By encapsulating varying behavior in different state objects and delegating state-specific tasks to these objects, the State pattern simplifies the code and makes it more maintainable.
Understanding the State Design Pattern
At its core, the State design pattern allows an object to alter its behavior when its internal state changes. This pattern is particularly useful when an object's behavior is dependent on its state, and the number of states is large or dynamic. By representing each state as an object, the State pattern enables a cleaner and more structured approach to managing state-specific behaviors.
The Problem: Unruly States
Consider a vending machine as an example. The behavior of a vending machine varies depending on its state – whether it is out of stock, currently dispensing a product, awaiting payment, or fully stocked. Without the State pattern, managing the transitions between these states and their associated behaviors can lead to complex conditional statements intertwined with the machine's core functionality.
The Solution: Applying the State Pattern
By applying the State pattern, each state of the vending machine – such as "OutOfStockState," "DispensingState," "AwaitingPaymentState," and "FullyStockedState" – becomes a separate class that encapsulates state-specific behavior. The context class, in this case, the vending machine, delegates the behavior to the current state object. When the state changes, the context's behavior changes accordingly without complex conditional logic.
Implementation in Java
Let's delve into a concrete implementation of the State pattern in Java. Suppose we have a vending machine that dispenses products based on its current state. We'll start by defining the State interface and concrete state classes, followed by the context class representing the vending machine.
// State interface
public interface VendingMachineState {
void insertCoin();
void selectProduct(String product);
void dispenseProduct();
}
// Concrete state classes
public class WorkingState implements VendingMachineState {
// Implement state-specific behavior
}
public class OutOfStockState implements VendingMachineState {
// Implement state-specific behavior
}
// Context class
public class VendingMachine {
private VendingMachineState currentState;
// Other vending machine attributes and methods
public void setCurrentState(VendingMachineState state) {
this.currentState = state;
}
// Delegate state-specific behavior to the current state
public void insertCoin() {
currentState.insertCoin();
}
public void selectProduct(String product) {
currentState.selectProduct(product);
}
public void dispenseProduct() {
currentState.dispenseProduct();
}
}
In this example, the VendingMachine
class maintains a reference to the current state object and delegates state-specific behaviors to it. When the state changes, the context's behavior is automatically updated.
Why Use the State Design Pattern
The State pattern offers several key benefits:
-
Simplifying Maintenance: As new states and behaviors need to be added, the State pattern makes it easier to introduce new state classes and modify existing ones without impacting the context class.
-
Improving Readability: By separating state-specific behavior into individual state classes, the code becomes more readable and easier to understand. Complex conditional statements are replaced with cohesive, encapsulated state classes.
-
Promoting Extensibility: The State pattern enables easier extension by allowing new states and behaviors to be added without modifying existing code, thereby promoting the Open/Closed Principle.
Real-World Application: Traffic Light Management
A classic example that illustrates the State pattern's real-world application is the management of traffic lights. The behavior of a traffic light at an intersection is heavily dependent on its current state, such as "GreenState," "YellowState," and "RedState." Implementing the State pattern in this scenario allows for clean, maintainable code where each state encapsulates its specific behavior.
The Bottom Line
In the realm of Java development, mastering the State design pattern is essential for efficiently managing complex state transitions. By encapsulating state-specific behavior into individual state classes, the State pattern leads to cleaner, more maintainable code and promotes extensibility. Understanding and applying this pattern enables developers to engineer elegant solutions for intricate state-dependent problems, ultimately leading to more robust and scalable applications.
Checkout our other articles