Navigating FlexyPool & Dropwizard Metrics: Renaming Woes
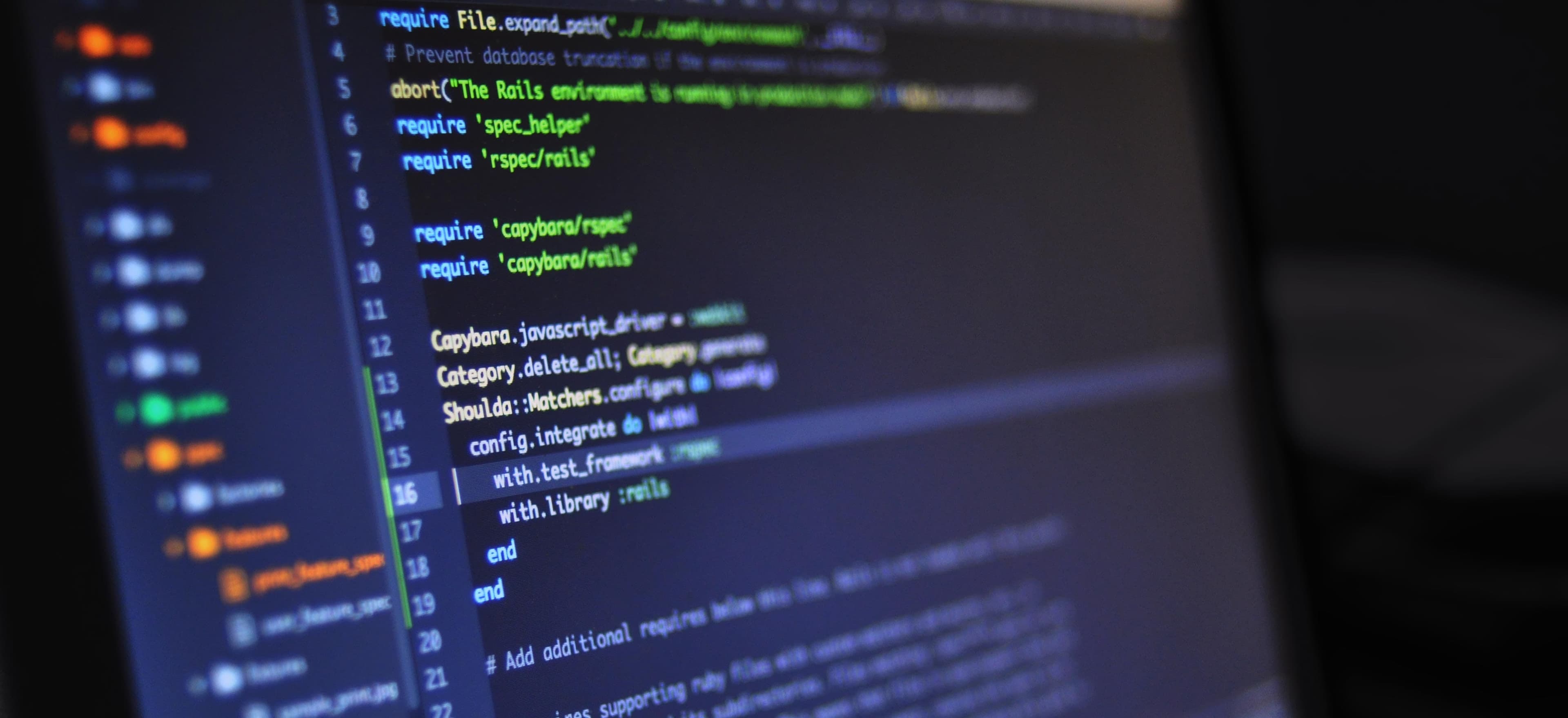
- Published on
Navigating FlexyPool & Dropwizard Metrics: Renaming Woes
In the world of Java development, utilizing libraries for optimizing database connection pooling is a common practice. One such popular library is FlexyPool, which offers great flexibility for monitoring and managing database connection pools. Additionally, Dropwizard Metrics is widely used for measuring various aspects of a Java application. Both of these libraries are powerful tools individually, but when used together, some unexpected challenges may arise.
This blog post will discuss one such challenge encountered when using FlexyPool and Dropwizard Metrics together: the renaming conflict that occurs due to both libraries using the same metric names. We'll explore the root cause of the issue, the impact it can have on monitoring and troubleshooting, and finally, the solution to effectively resolve the conflict.
Understanding the Issue
When integrating FlexyPool and Dropwizard Metrics into a Java application, you may notice that FlexyPool uses Dropwizard Metrics under the hood to publish metrics. This can lead to a clash when both libraries attempt to register metrics with the same names, causing confusion and ambiguity in the gathered data.
For instance, FlexyPool and Dropwizard Metrics both use a metric named Connections
to track the number of active database connections. When these metrics get registered with the same name in the MetricRegistry, it becomes challenging to differentiate between the two and extract relevant insights from the collected data.
The Impact
The impact of this naming conflict is significant, especially when analyzing and monitoring the performance of the database connection pool. Without clear and distinguishable metrics, it becomes arduous to pinpoint the source of issues such as connection leaks, spikes in connection usage, or other performance anomalies.
Moreover, when leveraging monitoring tools or dashboards that rely on these metrics, the lack of proper differentiation can lead to misleading visualizations and inaccurate interpretations of the actual state of the connection pool.
To address this issue effectively, let’s dive into a practical solution that involves renaming the conflicting metrics to provide clarity and precision in monitoring and troubleshooting.
The Solution: Renaming Metrics
To resolve the naming conflict between FlexyPool and Dropwizard Metrics, we can rename the metrics published by FlexyPool to ensure uniqueness and avoid overlapping with the metrics from Dropwizard Metrics.
One approach to achieve this is by leveraging the MetricRegistryListener
provided by FlexyPool. This listener allows us to intercept and modify the metrics before they are registered in the MetricRegistry, enabling us to rename them as desired.
Let’s take a look at how we can implement this solution:
Step 1: Implement MetricRegistryListener
First, we need to create a custom MetricRegistryListener
that intercepts the metrics being registered by FlexyPool and renames them. Below is an example of how this can be achieved:
import com.vladmihalcea.flexypool.metric.MetricRegistry;
import com.vladmihalcea.flexypool.metric.MetricRegistryListener;
public class CustomMetricRegistryListener implements MetricRegistryListener {
@Override
public void onGaugeAdded(String metricName, com.codahale.metrics.Gauge<?> gauge) {
// Check if the metricName matches the one to be renamed
if ("Connections".equals(metricName)) {
MetricRegistry.INSTANCE.remove(metricName);
MetricRegistry.INSTANCE.register("FlexyPoolConnections", gauge);
}
}
// Implement other overridden methods as needed
}
In this example, we are checking if the metric name is Connections
and then renaming it to FlexyPoolConnections
before registering it in the MetricRegistry.
Step 2: Register MetricRegistryListener
Next, we need to register our custom MetricRegistryListener
with FlexyPool to ensure that our renaming logic is applied. This can be done during the initialization of FlexyPool, as shown below:
import com.vladmihalcea.flexypool.FlexyPoolDataSource;
import javax.sql.DataSource;
DataSource actualDataSource = // Obtain the actual DataSource
CustomMetricRegistryListener customListener = new CustomMetricRegistryListener();
FlexyPoolDataSource flexyPoolDataSource = new FlexyPoolDataSource(actualDataSource, customListener);
By associating the custom MetricRegistryListener
with FlexyPool, we ensure that our renaming logic is triggered whenever metrics are being registered, allowing us to rename the conflicting metrics appropriately.
Final Thoughts
By addressing the naming conflict between FlexyPool and Dropwizard Metrics through metric renaming, we have successfully mitigated the ambiguity and confusion in monitoring database connection pool metrics.
This solution enhances the clarity and accuracy of the metrics, enabling efficient troubleshooting, performance analysis, and visualization of the database connection pool state. With distinct and well-labeled metrics, developers and operations teams can make more informed decisions and effectively manage the database connection pool.
In conclusion, while integrating powerful libraries like FlexyPool and Dropwizard Metrics can bring substantial benefits, understanding and managing potential conflicts is crucial to harness their full potential without encountering unexpected hurdles.
With a strategic approach to resolving such conflicts, we can harness the collective capabilities of these libraries, elevating the robustness and observability of Java applications.
This wraps up our exploration of the renaming woes when navigating FlexyPool and Dropwizard Metrics. We have delved into the impact of the conflict, the solution through metric renaming, and the resulting advantages in monitoring and troubleshooting.
Stay tuned for more insights into Java development best practices and effective utilization of libraries!
Checkout our other articles