Solving the Spring 3.1 Profiles Puzzle on Tomcat
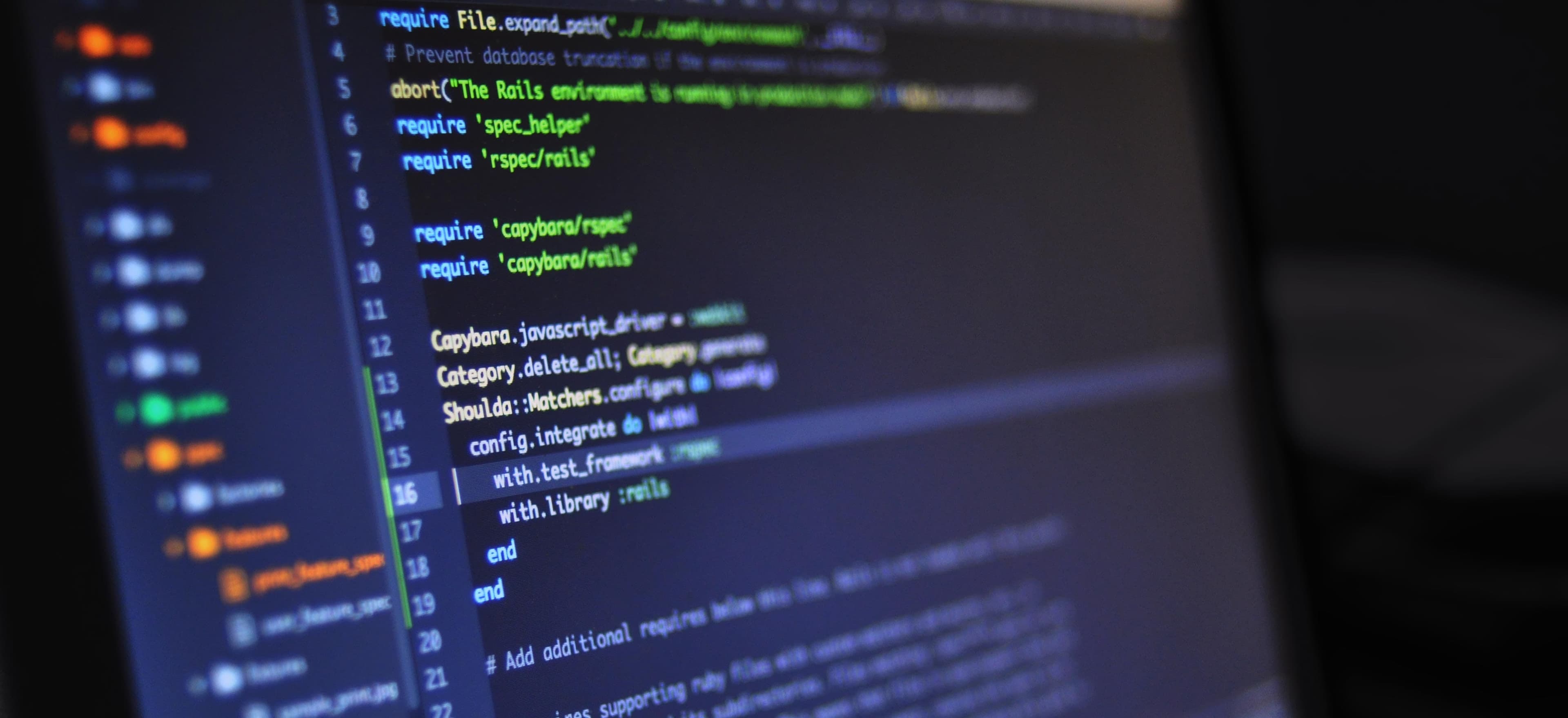
- Published on
A Guide to Configuring Spring 3.1 Profiles on Tomcat
When it comes to managing different environments, such as development, testing, and production, Spring 3.1 profiles offer a viable solution by allowing developers to segregate bean configurations for specific environments. However, deploying these profiles on Tomcat can sometimes feel like solving a puzzle. In this post, we will demystify the process of configuring Spring 3.1 profiles on Tomcat.
Understanding Spring Profiles
Spring Profiles provide a way to segregate parts of your application configuration and make it available only in certain environments. This can be extremely useful when you have different database configurations, service integrations, or logging levels for different environments. It allows you to have different configurations for development, testing, and production without having to maintain separate codebases.
In Spring 3.1, profiles are activated through the use of the @Profile
annotation, XML configuration, or property files. When the application is started, a specific profile can be activated, and only the beans associated with that profile will be registered in the application context.
Configuring Spring Profiles for Tomcat
Step 1: Setting Up Maven Dependencies
To get started, ensure that you have the necessary dependencies in your pom.xml
file for processing Spring profiles. Here's an example of how you can include the required dependencies:
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>3.1.0.RELEASE</version>
</dependency>
Step 2: Creating Profile-specific Bean Configurations
In your Spring configuration, you can define beans that are specific to certain profiles. Let's say you have a DataSource
bean that needs to be different for development and production environments. You can achieve this as follows:
@Configuration
public class DataConfig {
@Bean
@Profile("dev")
public DataSource devDataSource() {
// Configuration for development environment
}
@Bean
@Profile("prod")
public DataSource prodDataSource() {
// Configuration for production environment
}
}
In the above code, the devDataSource()
bean will be registered only when the "dev" profile is active, and similarly for prodDataSource()
.
Step 3: Activating Profiles in Tomcat
To activate a specific profile when deploying on Tomcat, you can utilize a context-param
in the web.xml
file. This can be achieved by including the following configuration:
<context-param>
<param-name>spring.profiles.active</param-name>
<param-value>dev</param-value> <!-- Replace with your desired profile -->
</context-param>
By setting the spring.profiles.active
parameter, you can specify which profile should be active when the application starts on Tomcat.
Step 4: Deploying the Application
After configuring the profiles and activating the desired profile in the web.xml
, you can proceed with deploying your application on Tomcat. Ensure that the necessary property files or annotation-based profile activation is in place.
Best Practices and Tips
-
Consistent Naming: Keep your profile names consistent across different environments to avoid confusion.
-
Externalized Properties: Consider externalizing profile-specific properties to easily manage configurations across different environments without changing the code.
-
Testing Profiles: Always thoroughly test your profiles to ensure that the correct beans are being activated for each environment.
Final Considerations
In conclusion, configuring Spring 3.1 profiles on Tomcat involves a strategic utilization of dependencies, profile-specific bean configurations, and activation of the desired profile. By following best practices and leveraging the flexibility offered by Spring profiles, you can seamlessly manage different environments without introducing complexities into your codebase.
By following these steps, you can effectively tackle the challenge of deploying Spring 3.1 profiles on Tomcat, ensuring that your application behaves consistently across different environments.
Incorporate these practices into your projects and witness the power of Spring profiles in action!
For further information on Spring profiles, refer to the official Spring Documentation.
Happy coding!
Checkout our other articles