Optimizing Hibernate ElementCollection for Improved Performance
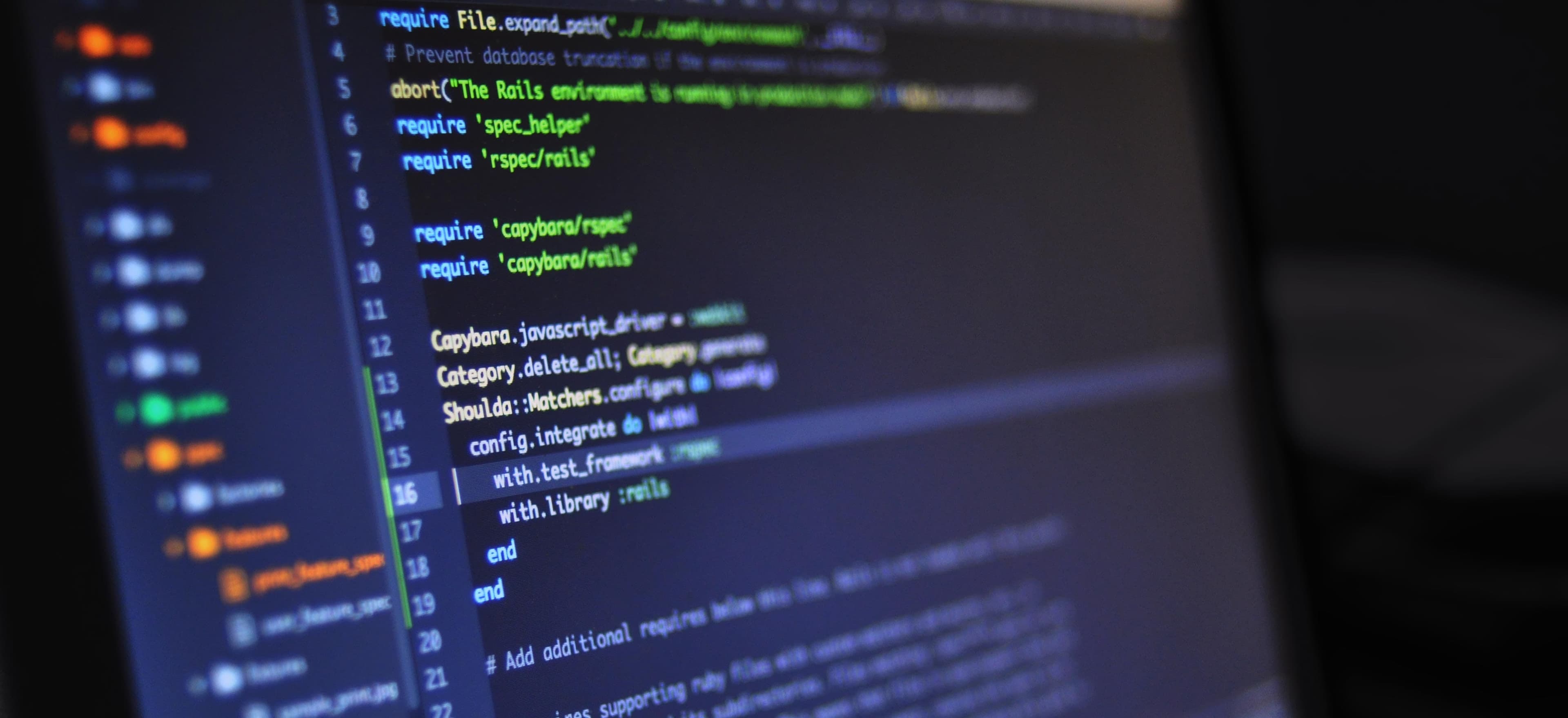
- Published on
Optimizing Hibernate ElementCollection for Improved Performance
When working with Hibernate and managing complex data structures, leveraging ElementCollection can be a powerful tool for storing collections of embedded objects. However, as the size of the collections grows, performance considerations become critical. In this article, we will explore strategies for optimizing ElementCollection in Hibernate to enhance application performance.
Understanding ElementCollection in Hibernate
In Hibernate, ElementCollection is used to map a collection of instances of a basic type or embeddable class. This allows us to persist a collection of simple or embeddable objects as a separate table that is joined to the owning entity.
Let’s consider a scenario where we have an entity Book
with an ElementCollection of Author
objects. This allows us to store multiple authors for a single book without creating a separate entity for authors.
@Entity
public class Book {
@ElementCollection
List<Author> authors;
// other fields and methods
}
Performance Challenges with ElementCollection
As the size of the ElementCollection grows, certain performance challenges can arise:
- Loading Overhead: Fetching entities with large ElementCollections can result in increased loading overhead, impacting application performance.
- Inefficient Updates: Modifying large ElementCollections may result in inefficient updates and impact database performance.
- Memory Consumption: Loading large ElementCollections into memory can consume significant resources, potentially leading to OutOfMemory errors.
To address these challenges, we need to optimize our usage of ElementCollection in Hibernate.
Strategies for Optimizing ElementCollection
1. Limiting Collection Size
If the size of an ElementCollection is expected to grow significantly, consider limiting the size to a reasonable threshold. This can be achieved by defining a custom @PrePersist
or @PreUpdate
method to enforce the size limit and prevent the collection from growing excessively.
2. Lazy Loading
By default, ElementCollection is eagerly fetched, which can lead to performance issues, especially when dealing with large collections. To mitigate this, we can configure the ElementCollection to be lazily loaded, ensuring that the collection is only fetched when accessed.
@ElementCollection(fetch = FetchType.LAZY)
3. Batch Fetching
To minimize the N+1 query issue when accessing ElementCollections, consider utilizing batch fetching. This can be achieved by specifying the @BatchSize
annotation on the collection field, allowing Hibernate to fetch the collection in batches rather than individually.
@BatchSize(size = 10)
4. Indexing
For large ElementCollections, adding indexes can significantly improve retrieval performance. By indexing the columns used for querying or sorting within the ElementCollection table, database lookups can be optimized.
5. Caching
Consider implementing caching mechanisms, such as second-level caching, to reduce the frequency of database access when dealing with ElementCollections. By caching the collection data, subsequent read operations can be served from the cache, improving overall performance.
6. Use of Inverse Attribute
When working with bidirectional associations and ElementCollection, utilizing the @Inverse
attribute can help optimize update operations by specifying the owning side of the association. This can prevent unnecessary updates and improve performance when modifying the collection.
To Wrap Things Up
Optimizing ElementCollection in Hibernate is crucial for managing large collections of embedded objects while ensuring efficient performance. By implementing strategies such as lazy loading, batch fetching, indexing, and caching, we can enhance the handling of ElementCollections and mitigate potential performance bottlenecks.
By carefully considering the specific requirements and use cases of ElementCollection in your Hibernate entities, you can effectively optimize performance and deliver a more responsive and scalable application.
Remember to always benchmark and profile your application to measure the impact of these optimizations and make informed decisions based on actual performance metrics.
Incorporate these strategies into your Hibernate projects to unleash the full potential of ElementCollection while maintaining optimal performance.
For further insights on optimizing Hibernate, you can refer to the official Hibernate documentation.
Start implementing these best practices to ensure your Hibernate-powered applications perform at their peak!
Checkout our other articles