Fixing instanceof Pitfalls in Java equals() Method
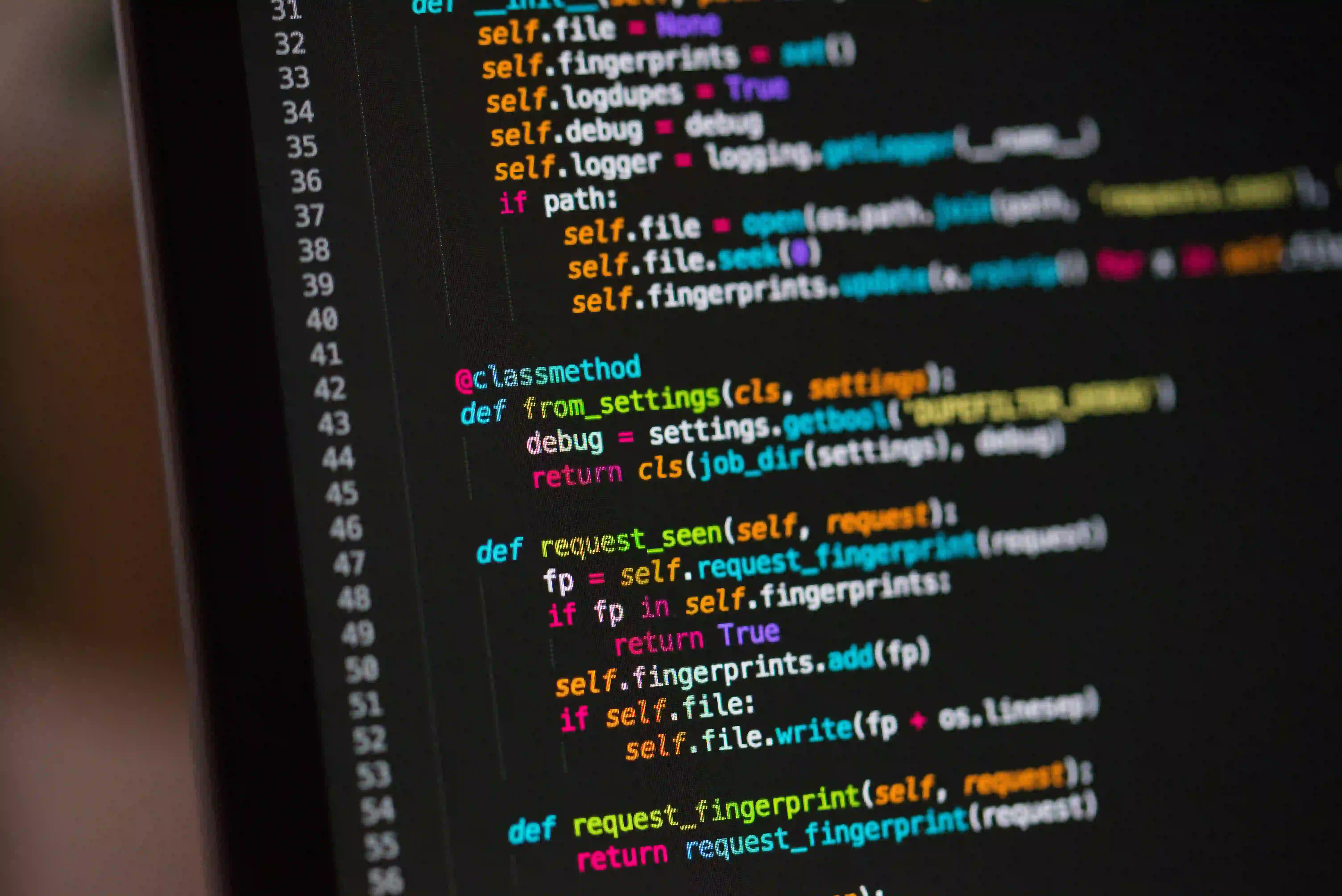
Fixing instanceof Pitfalls in Java equals() Method
Java's equals()
method is used to compare the equality of objects. When overriding this method, it's crucial to handle instances of different classes properly to avoid bugs and unexpected behavior. One common pitfall is the improper use of instanceof
inside the equals()
method, which can lead to incorrect results and violates the transitivity requirement of the equals()
method.
In this blog post, we'll discuss the pitfalls of using instanceof
in the equals()
method, explore the reasons behind its inefficacy, and provide alternative solutions to ensure the correctness and robustness of your equals()
method implementation.
The instanceof Pitfall
Let's say we have a Circle
class and a Rectangle
class, both of which subclass a common Shape
class. Now, consider the following faulty implementation of the equals()
method in the Circle
class:
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
Circle circle = (Circle) obj;
return this.radius == circle.radius;
}
The problem with this implementation is the use of getClass()
and instanceof
to perform type checking. This can lead to unexpected behavior when comparing objects of different subclasses of the Shape
class, violating the symmetric and transitive properties of the equals()
method.
The Pitfalls Explained
The instanceof
operator checks whether an object is an instance of a particular class, including its subclasses. When used in the equals()
method, it can cause issues because it doesn't uphold the Liskov substitution principle. This principle states that objects of a superclass should be replaceable with objects of its subclasses without affecting the correctness of the program.
The use of instanceof
in equals()
violates the principle and can lead to incorrect comparisons, especially when dealing with inheritance hierarchies, potentially causing unexpected behavior and bugs.
Alternative Approaches
To overcome the pitfalls associated with using instanceof
in the equals()
method, consider the following alternative approaches:
Class Checking
Instead of using instanceof
, check for the class equality using the getClass()
method. This approach ensures that the comparison is restricted to objects of the exact class, adhering to the principles of object-oriented programming.
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj == null || getClass() != obj.getClass()) {
return false;
}
Circle circle = (Circle) obj;
return this.radius == circle.radius;
}
By checking for class equality using getClass()
, we avoid the pitfalls associated with instanceof
and ensure that the comparison is limited to objects of the same class.
getClass() vs. instanceof
It's important to understand the distinction between getClass()
and instanceof
. While getClass()
returns the runtime class of an object, instanceof
checks if an object is an instance of a particular class or its subclasses. By using getClass()
, we focus on exact class comparison, promoting a more robust and predictable equals()
method.
Closing the Chapter
In conclusion, the improper use of instanceof
in the equals()
method can lead to bugs and violate the principles of object-oriented programming. By understanding the pitfalls associated with instanceof
and adopting alternative approaches such as class checking using getClass()
, you can ensure the correctness and reliability of your equals()
method implementation.
It's essential to always consider the implications of type checking and class hierarchies when implementing the equals()
method to guarantee consistent and expected behavior.
By addressing the pitfalls of using instanceof
in the equals()
method, you can enhance the consistency and reliability of your Java applications, leading to improved code quality and reduced potential for bugs and unexpected behavior.
For further reading on best practices for implementing the equals()
method in Java, check out Effective Java, Third Edition by Joshua Bloch, which provides comprehensive insights into writing effective and robust Java code.
Take the time to review and refine your equals()
method implementations to ensure the integrity and stability of your Java applications. By addressing these pitfalls, you can elevate the quality and maintainability of your codebase, promoting a more efficient and reliable software development process.