Scala and Scalatra: Overcoming the Initial Learning Curve
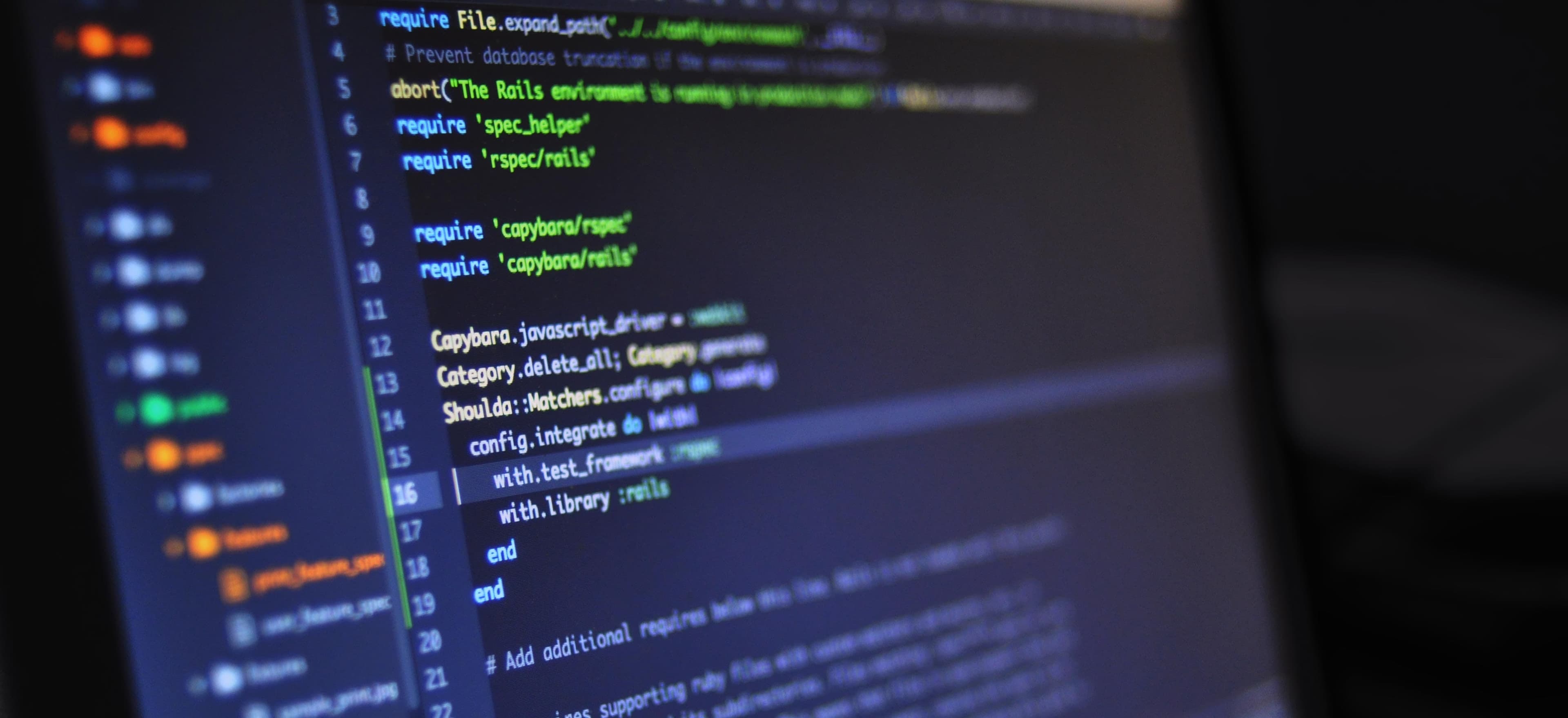
- Published on
Understanding Scala
Scala is a powerful language that combines object-oriented and functional programming in a concise, elegant syntax. It runs on the Java Virtual Machine (JVM) and offers full interoperability with Java. Scala's expressive syntax allows for the creation of complex, scalable, and maintainable applications.
When venturing into Scala development, one may encounter a learning curve, especially if coming from a purely object-oriented background. However, the benefits of mastering Scala are substantial. With its strong type system, support for immutability, and powerful functional programming capabilities, Scala enables developers to write robust and highly performant code.
Getting Started with Scalatra
Scalatra, a web application framework written in Scala, provides a lightweight and flexible environment for building RESTful web services. Despite its simplicity, Scalatra offers great extensibility and a high level of control, making it an attractive choice for developing scalable web applications.
Overcoming the Initial Hurdles
As with any new technology, getting past the initial learning curve is essential to fully benefit from its potential. In the case of Scala and Scalatra, this involves understanding the fundamental concepts of the language and the framework, as well as familiarizing oneself with best practices and common patterns.
Let's dive into some key strategies for overcoming the initial learning curve when working with Scala and Scalatra.
Embracing Functional Programming
One of the major paradigm shifts when transitioning to Scala is embracing functional programming. Despite the initial challenges, understanding and harnessing the power of functions as first-class citizens can lead to more maintainable and concise code.
// Example of a higher-order function in Scala
def operateOnNumber(num: Int, operation: Int => Int): Int = {
operation(num)
}
val result = operateOnNumber(5, x => x * x) // Output: 25
In the above code snippet, the operateOnNumber
function takes an integer and a function as arguments. This exemplifies the elegance of functional programming, where functions can be passed as parameters and returned as values.
By leveraging functional programming concepts such as immutability, higher-order functions, and pattern matching, developers can write code that is not only more robust but also more concise and expressive.
Leveraging Scala's Type System
Scala's rich type system allows for the creation of highly descriptive and strongly-typed code. While this can initially seem overwhelming, it ultimately leads to more reliable and maintainable codebases.
// Example of defining a case class in Scala
case class User(id: Long, name: String, email: String)
val user = User(1, "John Doe", "john@example.com")
In the above example, the User
case class provides a clear and structured way to define user data, with minimal boilerplate code. Leveraging Scala's type system in this manner enhances code readability and reduces the likelihood of runtime errors.
Unleashing the Power of Scalatra
Once a solid understanding of Scala is in place, transitioning to Scalatra becomes more approachable. Scalatra's lightweight nature and emphasis on simplicity make it an ideal choice for building RESTful APIs and web services.
Routing and Controllers in Scalatra
Scalatra's routing DSL allows for defining RESTful routes with ease, providing a clear and concise way to map HTTP requests to controller logic.
// Example of defining routes in Scalatra
get("/users") {
// Logic to retrieve and return a list of users
}
post("/users") {
// Logic to create a new user
}
In the above snippet, the get
and post
functions define routes for handling HTTP GET and POST requests, respectively. This straightforward approach to routing simplifies the process of exposing API endpoints and handling incoming requests.
Embracing Scalatra's Extensibility
Scalatra's extensible nature allows developers to plug in additional functionality as needed, making it a versatile framework for a wide range of web application scenarios.
// Example of adding a custom filter in Scalatra
class CustomFilter extends ScalatraFilter {
before() {
// Logic to be executed before each request
}
after() {
// Logic to be executed after each request
}
}
In the above example, a custom filter is defined to execute logic before and after each request. This exemplifies Scalatra's extensibility, enabling developers to tailor the request-handling pipeline to their specific requirements.
Wrapping Up
Mastering Scala and Scalatra may pose initial challenges, but the benefits of doing so are immense. By embracing functional programming, leveraging Scala's type system, and harnessing the power of Scalatra, developers can create robust, scalable, and maintainable web applications.
Overcoming the initial learning curve is a worthwhile investment, and with the right approach and perseverance, Scala and Scalatra can unlock a world of possibilities for web application development.
In conclusion, by embracing the elegance of Scala and the flexibility of Scalatra, developers can pave the way for building powerful and efficient web applications.
To delve further into Scala and Scalatra, consider exploring the comprehensive documentation provided by the official Scala website (scala-lang.org) and the Scalatra project repository on GitHub (github.com/scalatra/scalatra).
Happy coding!