Mastering Sorted Pagination in Cassandra: Tips & Tricks
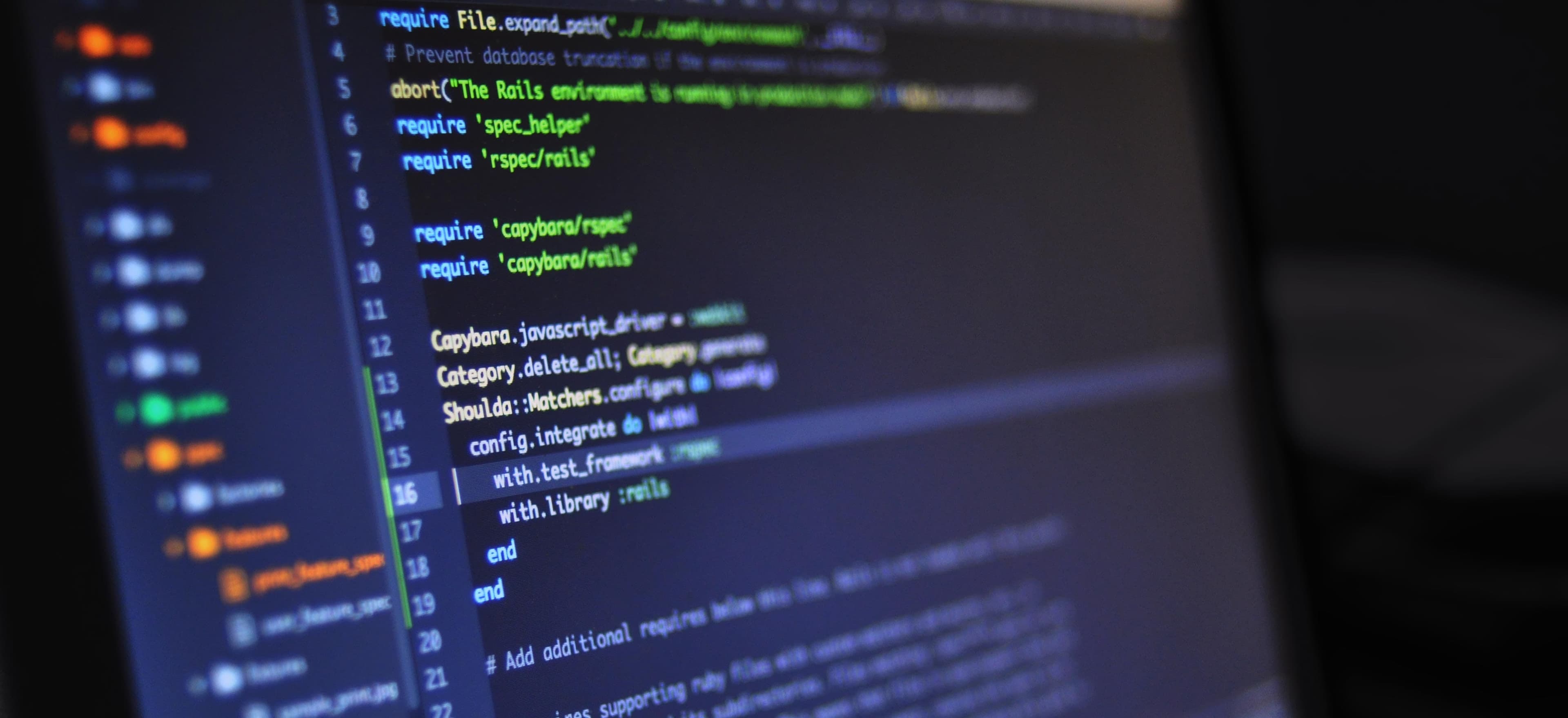
- Published on
Mastering Sorted Pagination in Cassandra: Tips & Tricks
When dealing with large datasets in Cassandra, implementing sorted pagination can be a challenge. In this post, we'll explore how to achieve efficient sorted pagination in Cassandra using the DataStax Java Driver. We'll cover the necessary code snippets and techniques to master sorted pagination effectively.
Understanding Sorted Pagination
Sorted pagination involves retrieving a subset of data from a large dataset while maintaining a specific order. In the context of Cassandra, this typically entails querying a table and retrieving results in a sorted manner based on a certain column or columns.
The Importance of Choosing the Right Clustering Column
In Cassandra, the clustering columns determine the sort order of the data within a partition. When implementing sorted pagination, selecting the appropriate clustering column or columns is crucial. It's essential to choose columns that align with the desired sorting criteria.
// Example of table creation with clustering columns
CREATE TABLE example_table (
partition_key text,
clustering_column1 int,
clustering_column2 text,
data text,
PRIMARY KEY (partition_key, clustering_column1, clustering_column2)
) WITH CLUSTERING ORDER BY (clustering_column1 ASC, clustering_column2 ASC);
In the above example, the clustering_column1
and clustering_column2
are designated as clustering columns, and the WITH CLUSTERING ORDER BY
specifies the desired sort order.
Using the DataStax Java Driver for Sorted Pagination
The DataStax Java Driver provides a robust set of tools for interacting with Cassandra. When it comes to sorted pagination, the driver's capabilities shine through.
Executing a Paginated Query
// Creating a SimpleStatement with pagination
SimpleStatement statement = SimpleStatement.builder("SELECT * FROM example_table WHERE partition_key = ?")
.addPositionalValue("value")
.setPageSize(10)
.build();
// Executing the paginated query
ResultSet rs = session.execute(statement);
The setPageSize
method allows us to specify the number of rows to be retrieved per page, facilitating paginated queries.
Retrieving and Handling Paginated Results
// Processing paginated results
ResultSet rs = session.execute(statement);
for (Row row : rs) {
// Process each row
}
When iterating over the ResultSet
, it's important to handle and process the retrieved rows systematically.
Efficient Sorted Pagination Techniques
Achieving efficient sorted pagination in Cassandra involves more than simple querying and retrieving. Here are some techniques to optimize sorted pagination performance.
Efficient Use of Clustering Columns
By carefully structuring the clustering columns based on the access patterns and sorting requirements, the efficiency of sorted pagination can be significantly improved. It's essential to understand the data access patterns and design the table schema accordingly.
Leveraging Secondary Indexes
In some scenarios, utilizing secondary indexes in conjunction with clustering columns can enhance sorted pagination. Secondary indexes can facilitate querying based on non-primary key columns, broadening the scope of sorted pagination possibilities.
// Creating a table with a secondary index
CREATE TABLE example_table (
partition_key text,
clustering_column1 int,
clustering_column2 text,
secondary_index_column text,
data text,
PRIMARY KEY (partition_key, clustering_column1, clustering_column2)
);
CREATE INDEX ON example_table (secondary_index_column);
In the above example, a secondary index is created on the secondary_index_column
, allowing queries based on this column in addition to the clustering columns.
Utilizing Apache Cassandra 3.0+ Features
Recent versions of Apache Cassandra, particularly 3.0 and above, have introduced features that can directly enhance sorted pagination. The introduction of materialized views and other improvements offer new avenues for optimizing sorted pagination queries.
Final Considerations
Mastering sorted pagination in Cassandra with the DataStax Java Driver requires a deep understanding of clustering columns, effective use of the driver's features, and optimization techniques tailored for Cassandra's data model. By carefully structuring table schemas, leveraging the driver's capabilities, and embracing Cassandra's evolving features, efficient and performant sorted pagination can be achieved.
Sorted pagination is a critical aspect of working with large datasets in Cassandra, and with the right approach and tools, it can be effectively tamed to meet the requirements of diverse use cases.
In conclusion, achieving efficient sorted pagination in Cassandra using the DataStax Java Driver is a multifaceted endeavor, demanding a blend of theoretical understanding and practical implementation.
To dive deeper into Cassandra, Java, and database optimization, check out the DataStax Academy for comprehensive learning resources.
Implementing sorted pagination in Cassandra goes beyond the basics, demanding a holistic approach that encompasses schema design, query execution, and performance optimization strategies. With the right techniques and tools at hand, mastering sorted pagination in Cassandra becomes an achievable goal.