Overcoming Chunk Size Challenges in Spring Batch
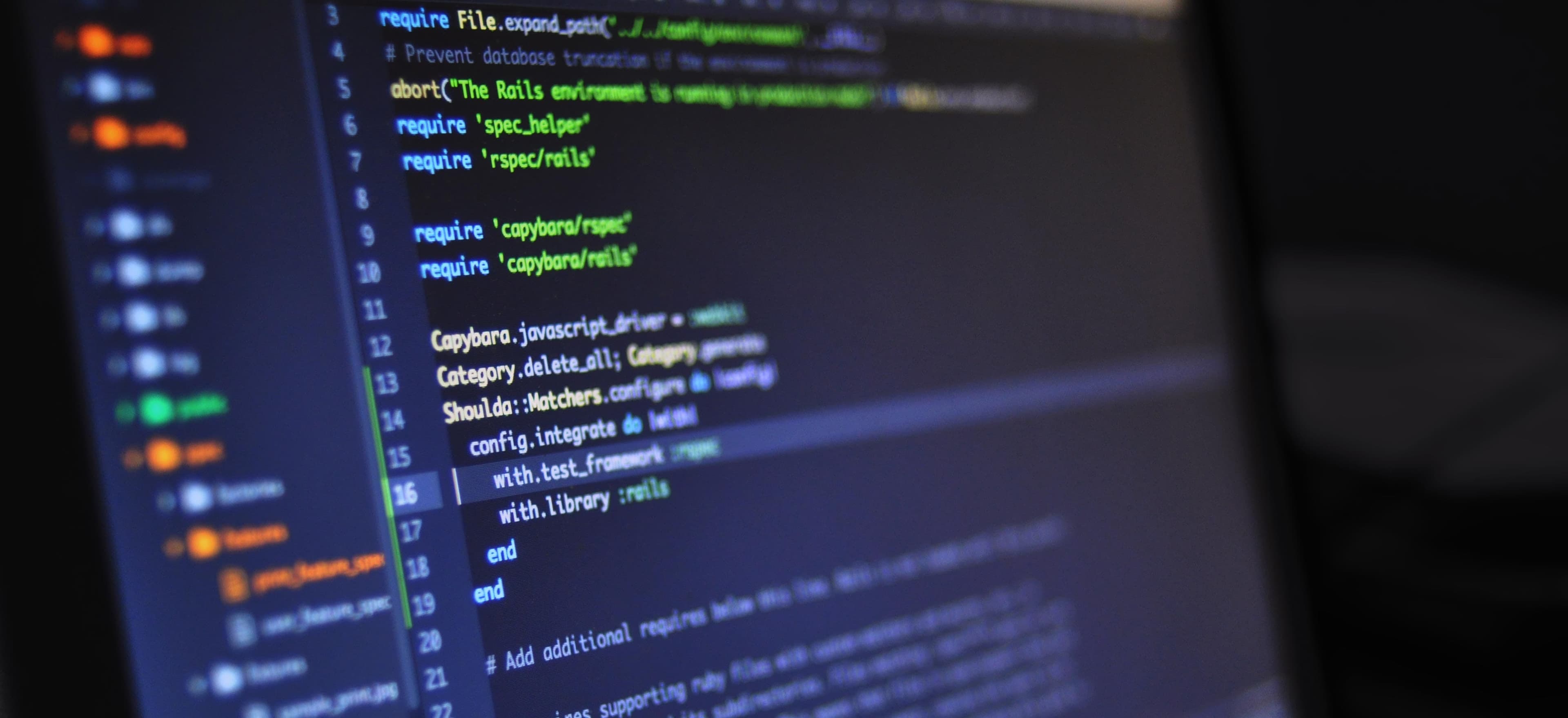
- Published on
Overcoming Chunk Size Challenges in Spring Batch
When working with large datasets in Spring Batch, a common challenge that developers face is deciding on the optimal chunk size for processing data efficiently. The chunk size determines the number of items that are read, processed, and written in each transaction. Finding the right balance is crucial for achieving optimal performance and resource utilization. In this article, we will delve into the significance of chunk size in Spring Batch, challenges associated with it, and strategies to overcome these challenges.
Understanding Chunk Processing in Spring Batch
In Spring Batch, chunk processing is a key concept that governs the efficiency of batch jobs. A chunk-oriented step, as the name suggests, processes data in chunks. The reading, processing, and writing of data occur within a transaction boundary defined by the chunk size. When the chunk size is exceeded, a commit point is reached, and the current transaction is committed before the next chunk is processed.
Significance of Chunk Size
The chunk size plays a pivotal role in the performance and efficiency of batch processing. A larger chunk size can enhance throughput by minimizing the overhead of transaction management, but it can also lead to increased memory consumption and longer transaction times. On the other hand, a smaller chunk size reduces memory consumption and transaction times but can introduce additional overhead due to frequent commits.
Challenges with Chunk Size
Memory Consumption
One of the primary challenges associated with determining the chunk size is managing memory consumption. A larger chunk size means more items are held in memory during processing, potentially leading to OutOfMemory errors, especially when dealing with large datasets. On the contrary, a smaller chunk size may alleviate memory pressure but could impact the overall efficiency of the batch job.
Transaction Overhead
Another challenge is the transaction overhead. With a larger chunk size, the overhead of managing the transaction for a large number of items in a chunk could impact the performance. Conversely, a smaller chunk size results in more frequent commits, potentially affecting the throughput of the batch job.
Strategies to Overcome Chunk Size Challenges
Dynamic Chunk Sizing
To address the challenges posed by static chunk sizes, Spring Batch provides the flexibility to dynamically determine chunk sizes. This allows the application to adapt the chunk size based on runtime conditions, such as memory availability and processing time. The dynamic chunk sizing approach involves periodically recalculating the chunk size to optimize resource utilization.
Throttling and Paging
Throttling the reading and writing of data can be an effective strategy to manage memory consumption. By implementing paging techniques, data can be read, processed, and written in smaller, manageable units, thus reducing the memory footprint. Additionally, the use of buffering and pagination can facilitate controlled access to the dataset, mitigating memory issues associated with large chunk sizes.
Experimental Analysis
Conducting performance experiments with varying chunk sizes can provide valuable insights into the optimal configuration for a specific batch job and dataset. By measuring the throughput, memory usage, and transaction times for different chunk sizes, developers can determine the most efficient chunk size that balances memory consumption and processing overhead.
Implementing Dynamic Chunk Size in Spring Batch
@EnableBatchProcessing
@Configuration
public class BatchConfiguration {
@Autowired
private JobBuilderFactory jobBuilderFactory;
@Autowired
private StepBuilderFactory stepBuilderFactory;
@Bean
public Job sampleJob() {
return jobBuilderFactory.get("sampleJob")
.start(sampleStep())
.build();
}
@Bean
public Step sampleStep() {
return stepBuilderFactory.get("sampleStep")
.<Input, Output>chunk(chunkSize()) // Dynamic chunk size
.reader(reader())
.processor(processor())
.writer(writer())
.build();
}
@Value("#{jobParameters[chunkSize]}")
private Integer chunkSize;
private ItemReader<Input> reader() {
// Define and return the reader
}
private ItemProcessor<Input, Output> processor() {
// Define and return the processor
}
private ItemWriter<Output> writer() {
// Define and return the writer
}
private int chunkSize() {
// Calculate and return the dynamic chunk size based on runtime conditions
}
}
In the above code snippet, the chunkSize()
method dynamically calculates the chunk size based on runtime conditions, enabling adaptive chunk sizing within the batch job.
My Closing Thoughts on the Matter
In the realm of Spring Batch processing, the chunk size is a critical factor that significantly influences the performance and resource utilization of batch jobs. By understanding the challenges associated with chunk size determination and implementing strategies such as dynamic chunk sizing, throttling, and experimental analysis, developers can effectively overcome chunk size challenges and optimize the processing of large datasets in Spring Batch applications.
References:
- Spring Batch Chunk Processing
- Throttling and Pagination in Spring Batch
- Dynamic Chunk Sizing in Spring Batch
In this blog post, we've explored the significance of chunk size in Spring Batch, the challenges it presents, and effective strategies to address these challenges. We've also provided a practical example of implementing dynamic chunk sizing in a Spring Batch job configuration. By adopting these insights and techniques, developers can empower their batch jobs to efficiently process large volumes of data, achieving optimal performance and resource utilization.
Checkout our other articles