Decoding Protocol Buffers: The Beginners Must-Know Guide
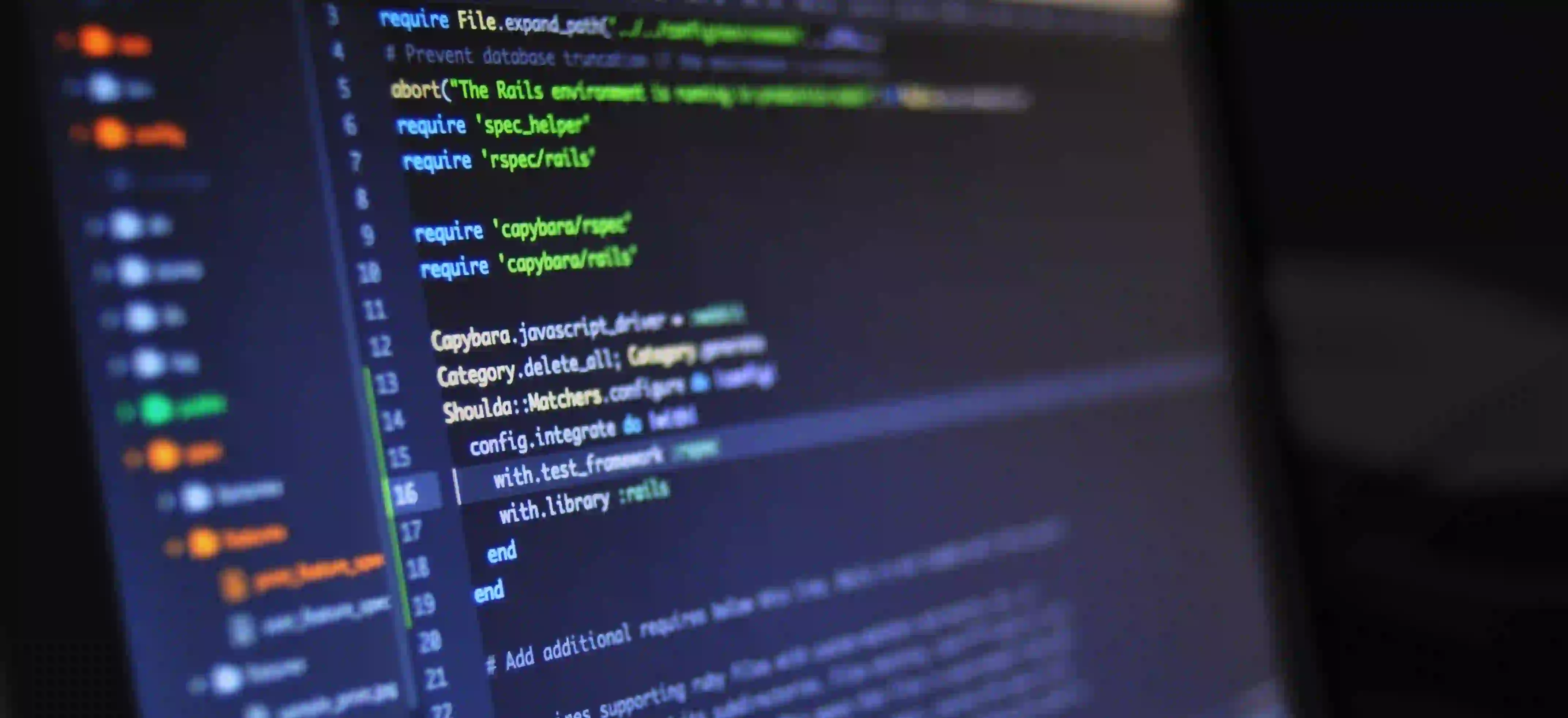
Decoding Protocol Buffers: The Beginners Must-Know Guide
If you are a beginner Java developer looking to decode Protocol Buffers, you are in the right place. Protocol Buffers, also known as protobuf, is a language-agnostic binary data serialization format developed by Google. It is widely used for serializing structured data to be used for communication protocols, data storage, and more. This guide will walk you through the basics of Protocol Buffers and how to decode them in Java.
What are Protocol Buffers?
Protocol Buffers are a language-neutral, platform-neutral, extensible way of serializing structured data for use in communications protocols, data storage, and more. They are designed to be more efficient than JSON or XML, making them ideal for use in high-performance or bandwidth-sensitive scenarios.
Protocol Buffers define a simple language to define the structure of your data, along with a set of code generators that generate data access classes from your definitions in a variety of programming languages. These generated classes provide simple and efficient APIs for reading and writing data encoded with Protocol Buffers.
Setting Up the Environment
Before decoding Protocol Buffers in Java, you need to have the required libraries in place. The most commonly used library for Protocol Buffers in Java is protobuf-java
. You can include it in your project using Maven or Gradle.
For Maven, add the following dependency:
<dependency>
<groupId>com.google.protobuf</groupId>
<artifactId>protobuf-java</artifactId>
<version>3.18.1</version>
</dependency>
For Gradle, include the dependency:
implementation 'com.google.protobuf:protobuf-java:3.18.1'
After setting up the environment, you are ready to decode Protocol Buffers in Java.
Decoding Protocol Buffers in Java
To decode Protocol Buffers in Java, you need the .proto
file which defines the message types and their fields. Let's take a simple .proto
file as an example:
syntax = "proto3";
message Person {
string name = 1;
int32 id = 2;
string email = 3;
}
In this example, we have a Person
message with three fields: name
, id
, and email
.
Generating Java Classes from .proto File
Once you have the .proto
file, you need to generate Java classes from it. You can use the Protocol Buffers compiler protoc
for this purpose. Additionally, you can use the protoc
plugin for Java, which is available as a Maven or Gradle plugin.
Here's an example of using the protoc
plugin for Maven:
<build>
<plugins>
<plugin>
<groupId>org.xolstice.maven.plugins</groupId>
<artifactId>protobuf-maven-plugin</artifactId>
<version>0.6.1</version>
<configuration>
<protocArtifact>com.google.protobuf:protoc:3.12.0:exe:${os.detected.classifier}</protocArtifact>
<pluginId>grpc-java</pluginId>
<pluginArtifact>io.grpc:protoc-gen-grpc-java:1.22.0:exe:${os.detected.classifier}</pluginArtifact>
</configuration>
<executions>
<execution>
<goals>
<goal>compile</goal>
<goal>compile-custom</goal>
</goals>
</execution>
</executions>
</plugin>
</plugins>
</build>
After generating the Java classes, you can start decoding Protocol Buffers in Java.
Decoding Protocol Buffers Example
Now, let's see an example of decoding Protocol Buffers in Java. Suppose you have a byte array byteData
containing serialized Protocol Buffers data of type Person
. Here's how you can decode it using the generated Java classes:
import com.example.PersonProto.Person;
public class ProtobufDecoder {
public static void main(String[] args) throws Exception {
byte[] byteData = { /* Populate byte array with serialized Protocol Buffers data */ };
// Decode the Protocol Buffers data
Person person = Person.parseFrom(byteData);
// Access the decoded fields
String name = person.getName();
int id = person.getId();
String email = person.getEmail();
System.out.println("Name: " + name);
System.out.println("ID: " + id);
System.out.println("Email: " + email);
}
}
In this example, Person.parseFrom(byteData)
is used to decode the Protocol Buffers data into an instance of the Person
class. Then, you can access the fields of the decoded Person
object using the generated getter methods.
In Conclusion, Here is What Matters
In this guide, you've learned the basics of Protocol Buffers and how to decode them in Java. You've set up the environment, generated Java classes from a .proto
file, and seen an example of decoding Protocol Buffers in Java. Protocol Buffers offer a fast and efficient way to serialize structured data, and with this knowledge, you are well-equipped to work with Protocol Buffers in your Java projects.
By understanding the role of Protocol Buffers in serialization and deserialization processes, you can develop a deeper understanding of efficient data management and communication in Java application development. Happy coding!
Remember to continuously check the Protocol Buffers documentation and Java Protocol Buffers API reference for more in-depth insights and hands-on practices.