Mastering @Context in JAX-RS: Solutions to Common Pitfalls
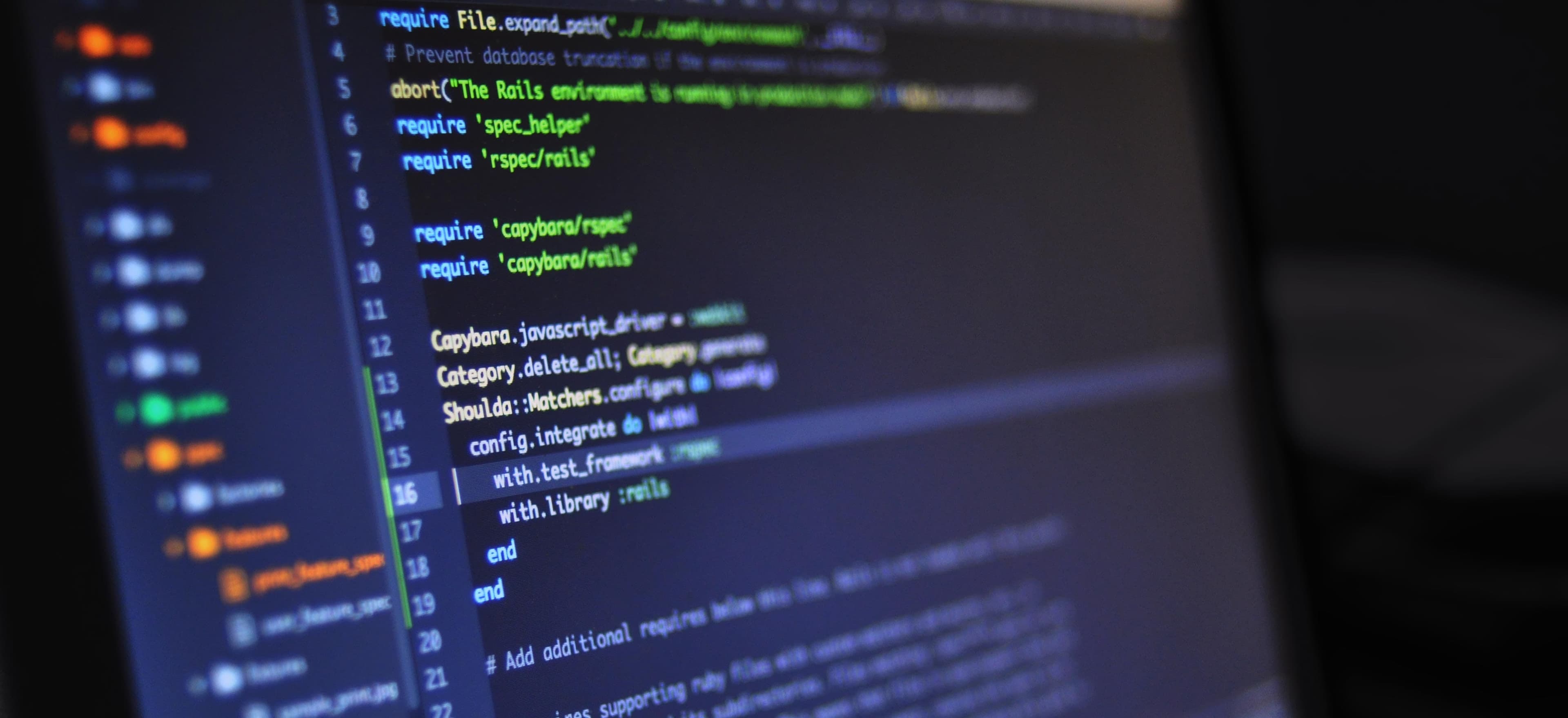
- Published on
Mastering @Context in JAX-RS: Solutions to Common Pitfalls
Introduction
In the world of Java web service development, JAX-RS (Java API for RESTful Web Services) plays a pivotal role in creating efficient and robust REST services. It provides a developer-friendly approach to building RESTful applications in Java. One of the key tools in the JAX-RS toolkit is the @Context
annotation, which allows developers to access various components such as HTTPHeaders, UriInfo, and SecurityContext in a type-safe manner. This article aims to guide readers through common pitfalls associated with using @Context
and how to navigate them effectively.
What is @Context in JAX-RS?
JAX-RS is a Java programming language API that provides support in creating web services according to the Representational State Transfer (REST) architectural style. The @Context
annotation plays a vital role in JAX-RS by allowing the injection of various contextual objects into a class.
@Path("/example")
public class ExampleEndpoint {
@Context
private UriInfo uriInfo;
// Demonstration of using UriInfo to access query parameters
}
In the code snippet above, the @Context
annotation is used to inject UriInfo
into the ExampleEndpoint
class. This grants access to information about the URI and the query parameters, leading to a cleaner and more intuitive way to handle this data in the context of a RESTful service.
Common Pitfall #1: Misunderstanding the Scope of Injected Objects
One common pitfall associated with the usage of @Context
is the misunderstanding of the scope of injected objects. Developers might expect the lifecycle of context-injected objects to be tied to the class they're injected into. However, this is not always the case. For example, an injected instance like HttpServletRequest
may not retain state across different requests. This misunderstanding can lead to unexpected behavior and hard-to-debug issues.
Solution to Pitfall #1
To address this pitfall, it is important to have a correct understanding of the lifecycle of injected objects. Context-injected objects are typically resolved per request, and new instances are provided for each request. It's vital to follow best practices in managing state if needed and avoid relying on the persistence of injected objects across multiple requests.
Common Pitfall #2: Incorrect Usage in Singleton Scopes
Another challenge with @Context
arises when it is used in classes annotated with @Singleton
, which defines a single instance per application. In this scenario, the behavior of @Context
may not align with expectations due to the lifecycle of the singleton scope.
Solution to Pitfall #2
To mitigate this issue, a recommended solution involves using the Provider<T>
pattern for lazy injection. By injecting a Provider<UriInfo>
instead of UriInfo
directly, the correct instance of the context can be obtained for each request within the singleton scope.
@Singleton
public class SingletonResource {
@Context
private Provider<UriInfo> uriInfoProvider;
// Use uriInfoProvider.get() to access UriInfo
}
Utilizing the Provider<T>
pattern ensures that the UriInfo
instance obtained is specific to the current request, allowing for proper management of contextual information within a singleton-scoped bean.
Common Pitfall #3: Overlooking SecurityContext
A crucial aspect often overlooked is the incorporation of SecurityContext
through the usage of @Context
for fine-grained authorization in RESTful services. Neglecting to leverage SecurityContext
can lead to inadequate handling of security-related aspects.
Solution to Pitfall #3
The incorporation of SecurityContext
through @Context
provides an effective means of authorizing API calls within a RESTful service. By injecting SecurityContext
, developers can perform role-based and user-based authorization effectively.
@Path("/secure")
public class SecureEndpoint {
@Context
SecurityContext securityContext;
// Method showing how to use SecurityContext for authorization
}
Leveraging SecurityContext
through the @Context
annotation allows for seamless integration of fine-grained authorization strategies, ensuring secure and robust REST services.
Best Practices and Tips
In summary, here are some best practices when using @Context
in JAX-RS:
- Understand the scope and lifecycle of injected objects, and manage state accordingly.
- Leverage the
Provider<T>
pattern for singleton-scoped beans to ensure proper context resolution. - Incorporate
SecurityContext
through@Context
for robust authorization mechanisms.
Conclusion
Mastering @Context
in JAX-RS is essential for creating efficient, secure, and maintainable Java-based RESTful services. By understanding and addressing common pitfalls associated with @Context
, developers can elevate their skills and build high-quality web services.
Call to Action
We invite readers to share their experiences, questions, or additional tips regarding the use of @Context
in JAX-RS. Additionally, you can explore the official JAX-RS specification for in-depth information on RESTful web services in Java.
Further Reading
- Best Practices for JAX-RS Development
- RESTful Web Services: Core Principles and Best Practices
- Java EE 8: Introduction to JAX-RS
Checkout our other articles