Demystifying Median Simulation with Inverse Distribution Functions
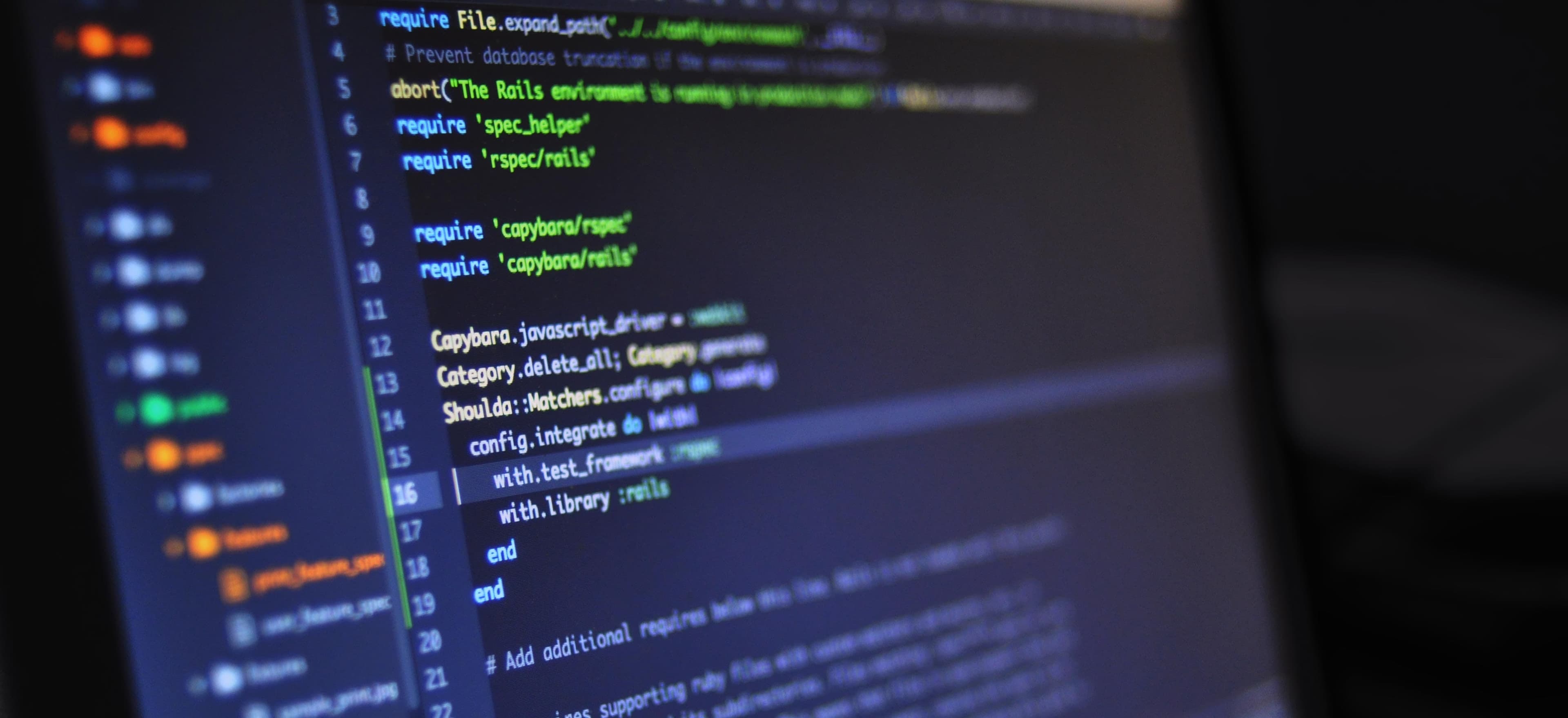
- Published on
Demystifying Median Simulation with Inverse Distribution Functions
If you've ever needed to simulate data with a specific median, you might have found yourself scratching your head. It's not as straightforward as setting the mean or standard deviation in a normal distribution. However, with the help of inverse distribution functions, this task becomes much more manageable.
Understanding the Challenge
Simulating data with a specific median presents a unique challenge because it requires working with the distribution's inverse function. Unlike the mean or variance, the median cannot be directly manipulated on many common distributions.
Enter Inverse Distribution Functions
Inverse distribution functions, also known as quantile functions, provide a solution to this challenge. These functions map a probability to the corresponding value in the distribution. In simpler terms, given a probability, the inverse distribution function returns the value from the distribution that corresponds to that probability.
Implementation in Java
Let's dive into a simple implementation of simulated data with a specific median using inverse distribution functions in Java.
import org.apache.commons.math3.distribution.NormalDistribution;
import org.apache.commons.math3.analysis.UnivariateFunction;
import org.apache.commons.math3.analysis.solvers.BrentSolver;
public class MedianSimulation {
public static void main(String[] args) {
double targetMedian = 5.0;
NormalDistribution distribution = new NormalDistribution(10.0, 2.0);
UnivariateFunction inverseCDF = (double p) -> distribution.inverseCumulativeProbability(p) - targetMedian;
BrentSolver solver = new BrentSolver();
double simulatedValue = solver.solve(100, inverseCDF, 0, 1);
System.out.println("Simulated Value with Median 5.0: " + simulatedValue);
}
}
In the code above, we first define the target median and create a normal distribution with a specified mean and standard deviation using the Apache Commons Math library. We then define the inverse cumulative distribution function (CDF) using a lambda expression.
The inverseCDF function sets up the equation where the difference between the inverse cumulative probability for a given probability and the target median is zero. This is the heart of using inverse distribution functions for median simulation.
We then utilize a solver, in this case, the BrentSolver, to find the solution that satisfies the equation. The solver iteratively refines the solution until the desired level of precision is achieved.
Finally, we print the simulated value that corresponds to the specified median.
Benefits of Using Inverse Distribution Functions
One of the key advantages of using inverse distribution functions for median simulation is the ability to manipulate the median directly, regardless of the distribution's shape. This method provides a more intuitive approach to generating data with a specific median.
My Closing Thoughts on the Matter
In this post, we've delved into the use of inverse distribution functions to demystify simulating data with a specific median. By harnessing the power of inverse distribution functions, we can more effectively tackle this unique simulation challenge.
If you are interested in exploring more about inverse distribution functions and simulation in Java, you might find the official Apache Commons Math documentation and Java documentation useful resources.