Dodging Pitfalls: Integrating MyBatis Caches with Ignite
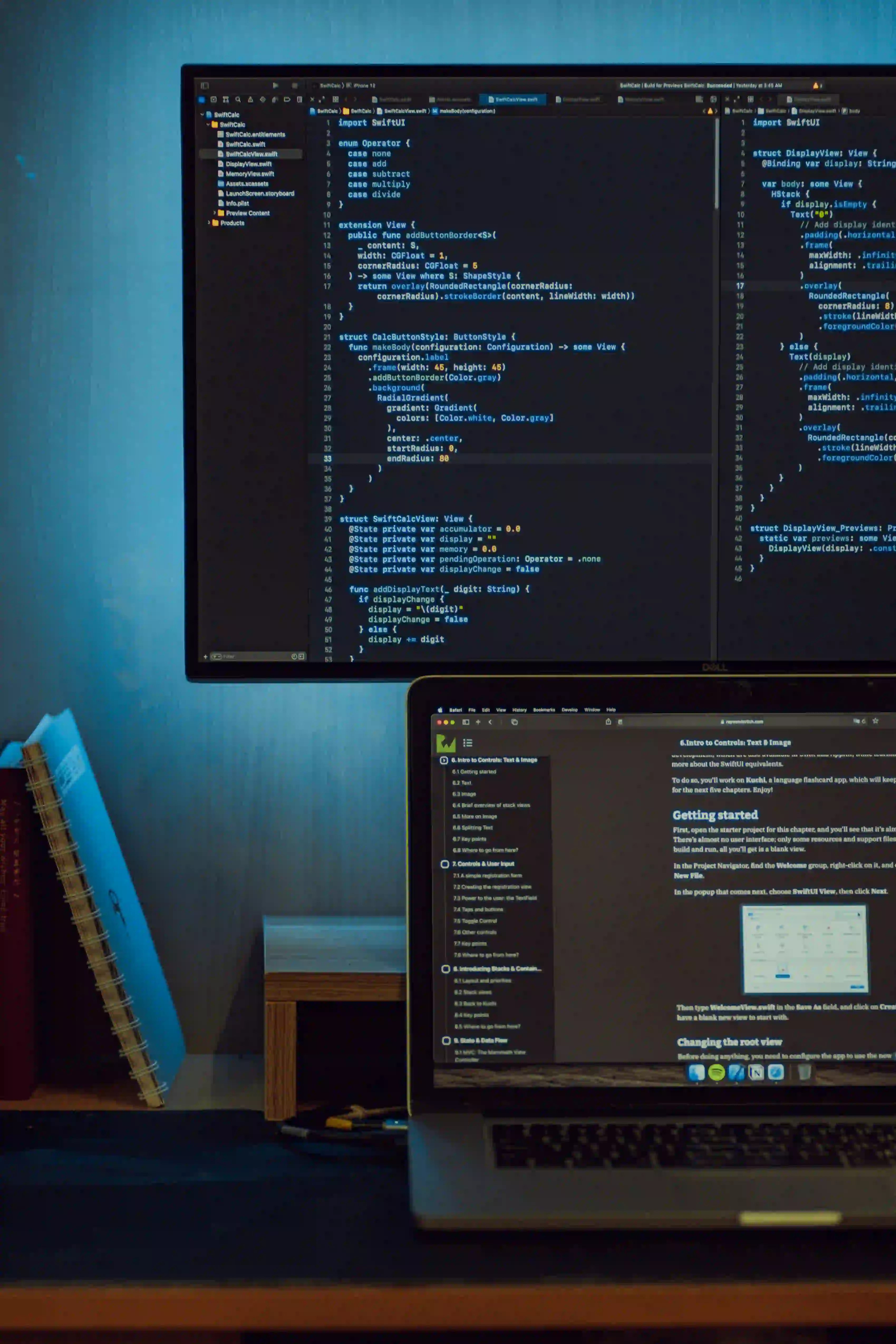
Dodging Pitfalls: Integrating MyBatis Caches with Ignite
Integrating MyBatis caches with Apache Ignite can be akin to walking a tightrope – thrilling, yet fraught with potential pitfalls. In this comprehensive guide, we'll take a deep dive into how to successfully marry MyBatis' powerful ORM capabilities with Ignite's lightning-fast in-memory data grid to supercharge your Java applications. Whether you're an experienced developer or just starting, this piece promises to equip you with the knowledge to seamlessly integrate these two powerhouse technologies while avoiding common mistakes.
Understanding the Duo: MyBatis and Ignite
MyBatis is a first-rate persistence framework that automates the mapping between SQL databases and objects in Java, .NET, and Ruby on Rails. It stands out for its simplicity and control over database interactions, making it a beloved choice among developers who prefer to steer clear of the full abstraction offered by ORM frameworks like Hibernate.
On the other side, Apache Ignite is an in-memory computing platform that significantly accelerates and scales applications by caching data in RAM, drastically reducing the need for frequent disk reads and writes. Its ability to distribute data across multiple nodes while ensuring consistency and reliability makes it an invaluable tool in today's data-intensive applications.
Integrating MyBatis with Ignite combines the straightforward and fine-grained database interaction provided by MyBatis with the sheer speed and scalability of Ignite’s in-memory data grid. But, as with any integration, there are pitfalls to beware.
Step-by-Step Integration Guide
Before diving in, ensure you have both MyBatis and Ignite added to your project dependencies. For Maven projects, you can do this by adding the respective dependencies in your pom.xml
file. For the latest version, check Maven Repository for MyBatis and Apache Ignite.
Step 1: Configure MyBatis to Use Ignite as a Cache Provider
MyBatis supports a custom cache implementation by configuring it in the mapper XML files. To integrate Ignite, you will need to create an Ignite cache configuration that MyBatis can leverage.
<cache type="com.example.MyBatisIgniteCacheAdapter">
<!-- Ignite specific properties can be added here -->
</cache>
In the MyBatisIgniteCacheAdapter
class, implement the MyBatis Cache
interface, initializing Ignite inside it. This adapter acts as a bridge between MyBatis and Ignite, enabling data to be stored and retrieved from the Ignite cache.
Implement the Cache Adapter
Here's a simplified version of what the MyBatisIgniteCacheAdapter
might look like:
public class MyBatisIgniteCacheAdapter implements Cache {
private final IgniteCache<Object, Object> cache;
public MyBatisIgniteCacheAdapter(String id) {
// Connect to Ignite node
Ignite ignite = Ignition.ignite();
// Get or create cache
cache = ignite.getOrCreateCache(id);
}
@Override
public void putObject(Object key, Object value) {
cache.put(key, value);
}
@Override
public Object getObject(Object key) {
return cache.get(key);
}
// Implement other methods defined in the Cache interface
}
This adapter facilitates the essential cache operations between MyBatis and Ignite, ensuring that data fetching strategies can now benefit from the distributed in-memory data grid.
Step 2: Fine-Tuning for Performance and Consistency
While this integration setup will get you started, understanding and adjusting the finer aspects of Ignite's cache configurations is key to unlocking optimal performance and consistency for your application.
For instance, considering the cache mode (PARTITIONED, REPLICATED, or LOCAL) based on your data access patterns can significantly impact performance and scalability. Similarly, diving deeper into Ignite's advanced features, like SQL queries, transactions, and compute capabilities, can provide even more ways to optimize your application.
Common Pitfalls to Avoid
While integrating MyBatis caches with Apache Ignite is powerful, it's not without its challenges. Here are some common pitfalls to watch out for:
- Overlooking Cache Invalidation: Proper cache invalidation is crucial. Outdated or stale data can lead to inconsistencies. Regularly review and test your cache invalidation logic to ensure data integrity.
- Misconfiguration: Both MyBatis and Ignite offer a slew of configurations. Incorrect configurations can lead to suboptimal performance or even application failures. Invest time in understanding the configuration options available.
- Ignoring Data Serialization: For Ignite to store objects in the cache, they must be serializable. Neglecting this can lead to runtime errors. Ensure your objects are efficiently serializable to take full advantage of Ignite’s fast data access.
The Closing Argument
Integrating MyBatis caches with Apache Ignite can transform your Java applications, making them faster and more scalable. By following the step-by-step guide provided and being mindful of common pitfalls, developers can leverage the combined powers of MyBatis' precision in database handling and Ignite's in-memory data grid performance.
However, the integration doesn't stop here. The landscape of data management and caching strategies is vast and continually evolving. Always be on the lookout for new features and best practices from both MyBatis and Apache Ignite communities. This proactive approach will ensure that your application remains at the cutting edge of performance and reliability.
Happy caching!