Boost Code Quality: The Crucial Role of Unit Testing
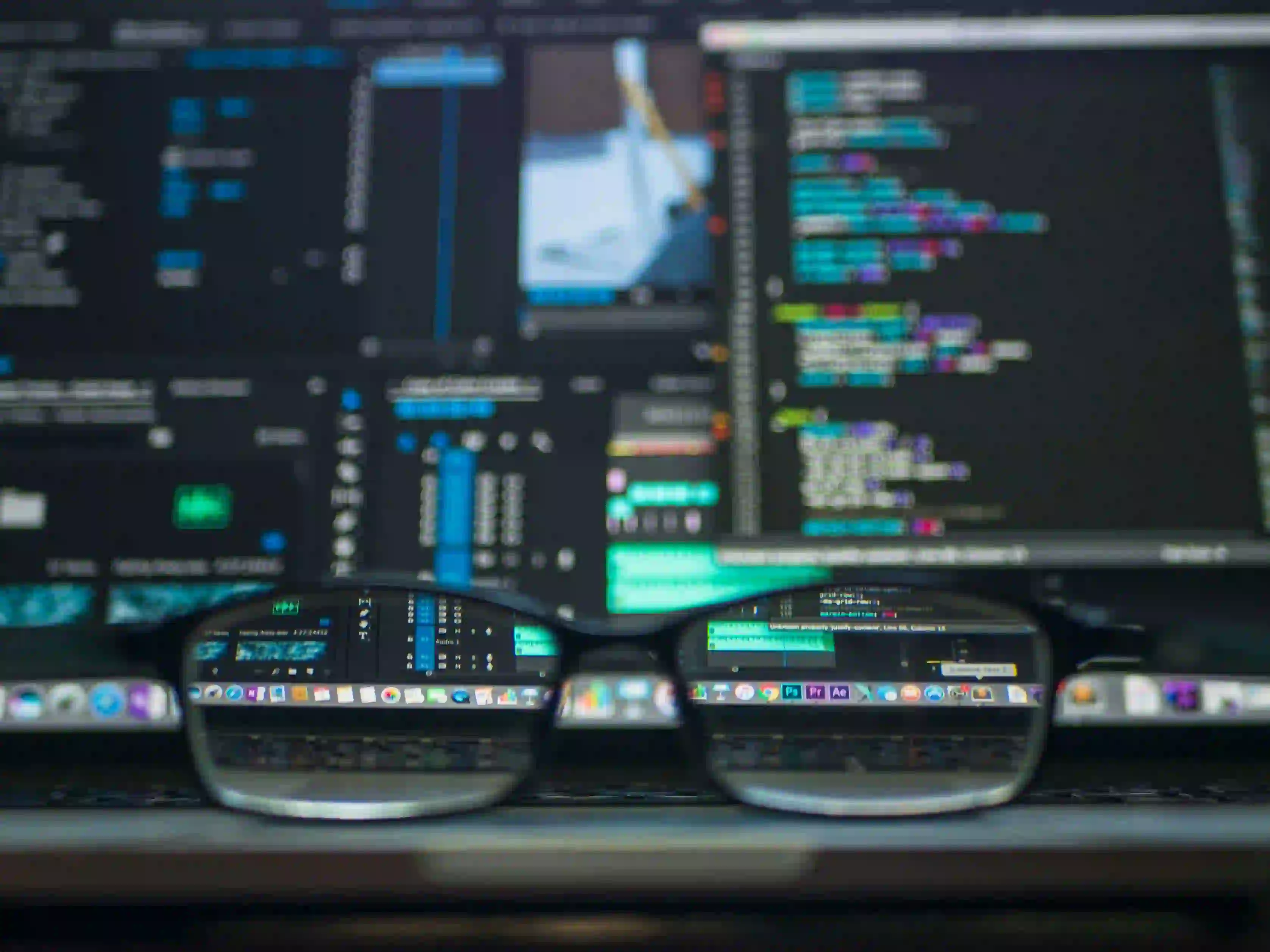
Boost Code Quality: The Crucial Role of Unit Testing
In the fast-paced world of software development, ensuring a high level of code quality is paramount. One powerful tool in achieving this goal is unit testing. When it comes to Java, unit testing helps in verifying the individual units of source code to ensure they perform as expected. This blog post will delve into the significance of unit testing in Java and demonstrate how it can significantly enhance code quality.
Why Unit Testing Matters
Unit testing plays a crucial role in the software development lifecycle. It allows developers to test individual units or components of a Java application under specific conditions. As a result, it provides a granular level of validation, enabling developers to identify and rectify issues early in the development process.
By writing unit tests, you can define how each piece of your code should behave under various scenarios. This not only aids in catching bugs early but also serves as living documentation, showcasing how the code should function.
The JUnit Framework
In the Java ecosystem, JUnit stands out as one of the most popular unit testing frameworks. JUnit provides a simple and elegant way to write and run repeatable tests. Let's take a look at a basic example of a JUnit test:
import static org.junit.jupiter.api.Assertions.assertEquals;
import org.junit.jupiter.api.Test;
public class MyMathTest {
@Test
public void testAddition() {
MyMath math = new MyMath();
int result = math.add(3, 5);
assertEquals(8, result, "3 + 5 should equal 8");
}
}
In this example, the MyMathTest
class contains a test method testAddition
that verifies the add
method from the MyMath
class returns the expected result. The assertEquals
method compares the expected result with the actual result obtained from running the add
method.
It's important to note that the use of assertions, such as assertEquals
, forms the cornerstone of unit tests in JUnit. These assertions play a critical role in verifying expected outcomes, thereby ensuring the correctness of the code.
Embracing Test-Driven Development (TDD)
Test-Driven Development (TDD) is a development approach that revolves around writing tests before implementing the corresponding code. This practice encourages a focus on the desired behavior of the code and promotes a more deliberate and conscious implementation.
By adhering to TDD, developers can gain a clearer understanding of the expected functionality and can subsequently build their code to meet those expectations. This results in code that is inherently testable and inclined towards higher quality and maintainability.
Integrating Unit Testing into Continuous Integration
As software projects grow in complexity, the need for continuous integration and continuous delivery (CI/CD) becomes increasingly critical. Unit testing serves as a cornerstone in this pipeline, allowing for the automatic validation of code changes in a systematic and frequent manner.
By integrating unit tests into a CI/CD pipeline, developers can swiftly identify defects that may arise due to code changes. Additionally, it instills confidence in the codebase by ensuring that new changes do not compromise the existing functionality.
Best Practices for Effective Unit Testing
While writing unit tests, it's imperative to follow best practices to ensure their effectiveness. Some of these best practices include:
- Isolation: Tests should be independent and not reliant on external factors. The use of mocking frameworks, such as Mockito, can aid in isolating the unit under test.
- Clarity and Readability: Write test cases that are clear and easily understandable. This ensures that the purpose and expected behavior of the code are evident to anyone reviewing the tests.
- Maintainability: Just like production code, tests require maintenance. Refactor tests as the codebase evolves to keep them aligned with the changes.
Wrapping Up
Unit testing in Java is not just about verifying code; it's about promoting a culture of quality, reliability, and maintainability. By leveraging tools like JUnit and embracing practices such as TDD, developers can significantly enhance their ability to produce robust, high-quality code.
Incorporating unit testing into the software development process is an investment that pays dividends in the form of reduced defects, improved code maintainability, and enhanced developer confidence. As software continues to evolve, the role of unit testing in ensuring code quality remains more crucial than ever.
In conclusion, striving for high code quality through unit testing is not just a choice; it's a necessity in today's software development landscape.
So, let's continue to write tests, advocate for best practices, and ultimately, elevate the overall quality of our Java code.
Remember, quality begins with each line of code and each test we write.
Happy coding and testing!