Overcoming Java 8's Limits with Javaslang Data Structures
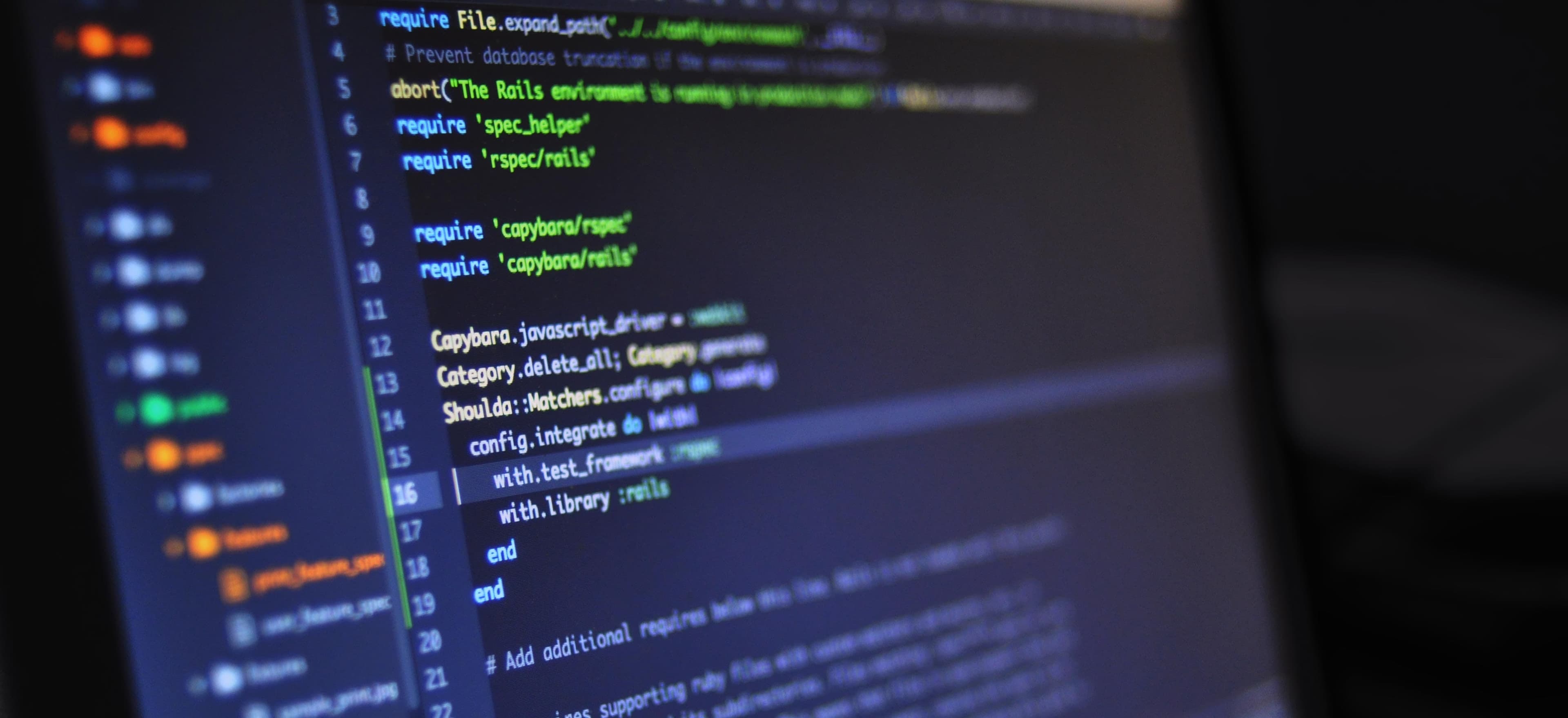
- Published on
Overcoming Java 8's Limits with Javaslang (Vavr) Data Structures
Introduction
Java 8 introduced significant improvements, especially in the area of functional programming, with the addition of lambda expressions, method references, and the Stream API. However, Java 8 has its limitations, particularly regarding data structures and functional programming capabilities. These limitations impact the ability to write concise, efficient, and immutable code. This article will explore how Javaslang, now known as Vavr, offers solutions to overcome these limitations.
What is Javaslang (Vavr) and Why it Matters
Javaslang, now called Vavr, is a functional library for Java that provides immutable, persistent data structures and functional control structures. It aims to complement Java 8 by extending its functional programming capabilities, offering a more expressive and concise way to write code. With Vavr, developers can embrace functional programming concepts such as immutability, pattern matching, and error handling more effectively in Java.
To learn more about Vavr, visit the official website: Vavr.io.
Understanding Java 8's Limitations in More Depth
In Java 8, the standard library provides data structures such as List, Set, and Map, but these structures have several limitations. Some of the key limitations include verbosity, mutability, and the lack of certain functional methods in Java's collection library.
Consider the verbosity when working with Java 8's collections. For example, when using Streams and lambdas, the code can become quite verbose. Moreover, mutable collections in Java 8 can lead to unexpected side effects and reduce the maintainability of the code. Additionally, Java 8's collections lack certain functional methods that are available in libraries like Javaslang/Vavr, making it cumbersome to perform common functional operations.
Introducing Javaslang's Data Structures
Vavr offers a rich set of data structures that are not present in Java 8. These include Tuple, Either, Option, Try, and more. These data structures bring immutability, expressiveness, and functional capabilities to Java, addressing the limitations present in Java 8's standard library.
Let's dive deeper into how Vavr's data structures enhance functional programming in Java.
How Javaslang Enhances Functional Programming in Java
-
Tuple:
- Why you're using the particular data structure: Tuples allow the grouping of a fixed number of items (elements) of different types into a single object, making it useful for representing compound values.
- How it improves upon Java 8's capabilities: Vavr's Tuple is immutable and allows developers to work with heterogeneous lists in a functional way without having to define custom classes.
- Code Snippet Example:
// Creating a Tuple in Java 8 List<Object> pair = Arrays.asList("hello", 10); // Creating a Tuple in Vavr Tuple2<String, Integer> pair = Tuple.of("hello", 10);
-
Option:
- Why you're using the particular data structure: Option represents an optional value, helping to deal with potentially absent values without explicitly checking for null.
- How it improves upon Java 8's capabilities: Vavr's Option allows for safer manipulation of values, reducing the risk of NullPointerExceptions and leading to more expressive and functional code.
- Code Snippet Example:
// Handling null value using Java 8's Optional Optional<String> name = // Some operation that may result in null // Handling null value using Vavr's Option Option<String> name = // Some operation that may result in null
-
Either:
- Why you're using the particular data structure: Either represents a value of two possible types, making it useful for scenarios where a computation could yield either a result or an error.
- How it improves upon Java 8's capabilities: Vavr's Either provides a functional approach to handling success or failure scenarios, allowing developers to handle errors in a more elegant and type-safe manner.
- Code Snippet Example:
// Handling success and failure using Java 8's Optional // Assumes a method 'fetchData' which may either succeed or fail Optional<Data> result = fetchData(); // Handling success and failure using Vavr's Either // Similar scenario using Vavr's Either Either<Error, Data> result = fetchData();
Performance Considerations
Vavr's data structures are designed for immutability and persistence, which can lead to potential performance improvements in certain scenarios. The memory usage and execution speed of Vavr's structures compared to Java 8's structures may vary. This difference can be minimal in some cases, but developers should consider the performance implications based on their specific use cases.
Getting Started with Javaslang in Your Projects
To incorporate Vavr into an existing Java project, add the following Maven/Gradle dependencies:
Maven:
<dependency>
<groupId>io.vavr</groupId>
<artifactId>vavr</artifactId>
<version>0.10.3</version>
</dependency>
Gradle:
implementation 'io.vavr:vavr:0.10.3'
For further guidance, refer to the official documentation for Vavr: Vavr Documentation
Conclusion
In conclusion, Vavr offers an array of data structures and functional programming capabilities that significantly enhance Java 8's functional programming capabilities. By addressing the limitations of Java 8's standard library, Vavr empowers developers to write more expressive, robust, and immutable code. As you explore Vavr, consider experimenting with its data structures and functional constructs to fully appreciate the benefits they bring to Java development. Don't hesitate to engage with the Vavr community and resources to further enhance your understanding and skills in functional programming with Vavr and Java.
By leveraging Vavr, Java developers can transcend the limitations of Java 8 and embrace a more functional and expressive programming style.
Now that you have learned about Vavr's capabilities, what aspects of your Java development would you like to enhance with Vavr's features? Share your thoughts and experiences in the comments below!