Mastering Matchers: Solving Common Testing Challenges
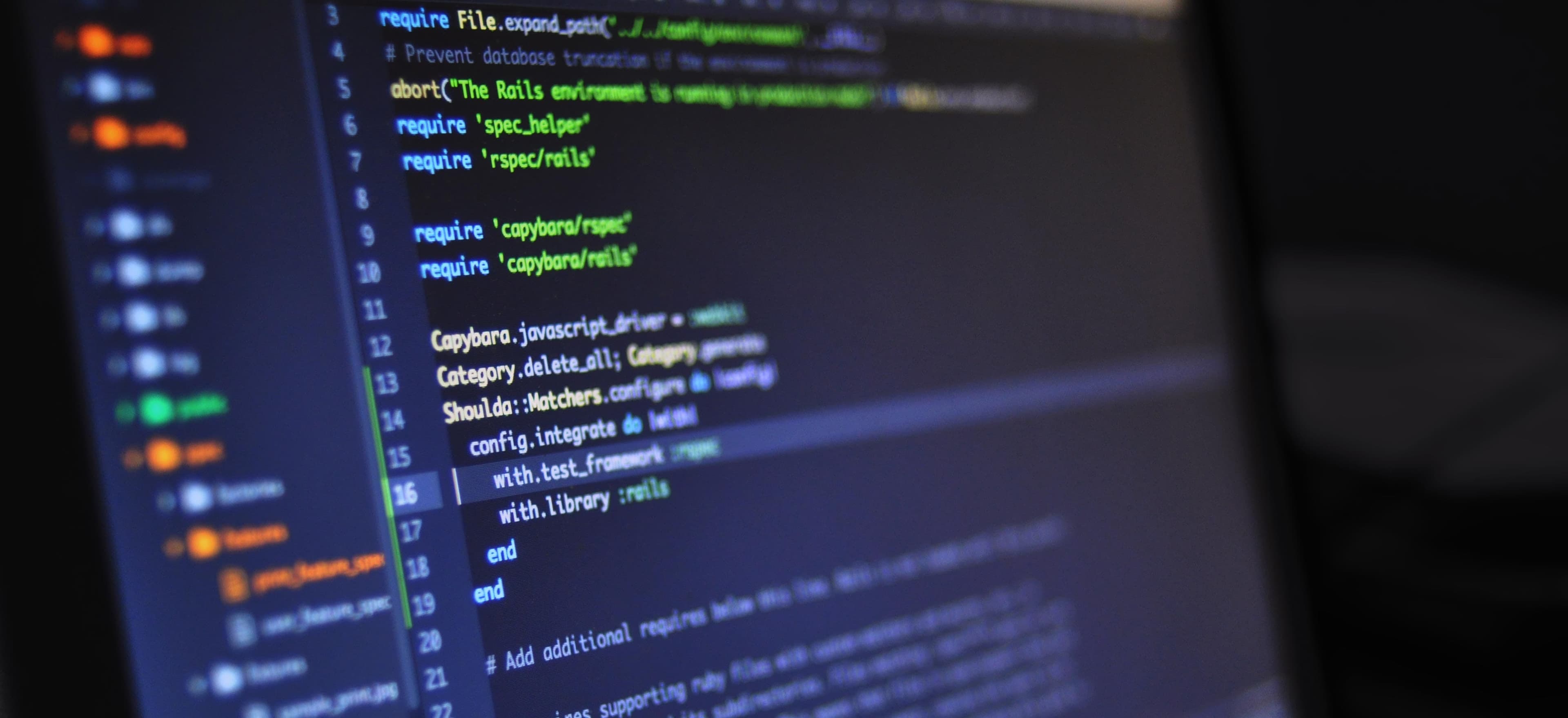
- Published on
Mastering Matchers: Solving Common Testing Challenges
When it comes to writing effective unit tests in Java, using the right tools and techniques can make a significant difference in the quality and efficiency of your testing process. One essential tool in a Java developer's testing arsenal is the concept of matchers. Matchers are powerful tools that allow developers to write expressive and flexible assertions in their unit tests.
In this blog post, we will explore the concept of matchers in Java and how they can be used to solve common testing challenges. We will delve into the benefits of using matchers, discuss various types of matchers, and provide practical examples of how matchers can be leveraged to write effective unit tests.
The Power of Matchers
Matchers are an integral part of popular testing frameworks in Java, such as JUnit and Mockito. They provide a way to define flexible and readable assertions in tests, making the intent of the tests clear and the failure messages informative. Matchers allow developers to write assertions that go beyond simple equality checks, enabling them to express complex conditions and verify various aspects of the code under test.
Types of Matchers
In Java testing frameworks, matchers come in different flavors, each catering to specific use cases and testing scenarios. Let's explore some common types of matchers and their benefits:
Hamcrest Matchers
Hamcrest is a popular matching library that provides a rich set of matchers for creating expressive and readable assertions. Using Hamcrest matchers, developers can define custom assertion logic and compose complex matchers using a fluent and flexible API. For example, the equalTo
, containsString
, and instanceOf
are some of the commonly used Hamcrest matchers that allow for intuitive and descriptive assertions in tests.
Argument Matchers in Mockito
When writing unit tests for code that depends on external dependencies, such as collaborating objects or services, Mockito's argument matchers come in handy. Mockito provides a range of argument matchers, such as eq
, any
, and argThat
, which enable developers to define flexible expectations on method arguments. This flexibility allows for robust and precise verification of method invocations and interactions with external dependencies.
Custom Matchers
In addition to using built-in matchers, developers can also create custom matchers tailored to their specific testing needs. Custom matchers are particularly useful when writing domain-specific tests or when the existing matchers do not fully capture the intent of the assertions. By creating custom matchers, developers can encapsulate reusable assertion logic and promote reusability and maintainability across their test suites.
Practical Examples
Let's illustrate the power of matchers through practical examples using JUnit and Mockito.
Using Hamcrest Matche'rs in JUnit
Consider a scenario where we want to test a simple Calculator
class that performs arithmetic operations. We can leverage Hamcrest matchers in our assertions to write expressive and meaningful tests:
import org.junit.Test;
import static org.hamcrest.MatcherAssert.assertThat;
import static org.hamcrest.Matchers.*;
public class CalculatorTest {
@Test
public void testAddition() {
Calculator calculator = new Calculator();
int result = calculator.add(2, 3);
assertThat(result, equalTo(5));
}
@Test
public void testDivision() {
Calculator calculator = new Calculator();
double result = calculator.divide(10, 2);
assertThat(result, closeTo(5, 0.001));
}
}
In the above example, we use Hamcrest matchers such as equalTo
and closeTo
to clearly specify the expected outcomes of the operations being tested.
Leveraging Argument Matchers in Mockito
For testing scenarios that involve mocking and verifying interactions with external dependencies, Mockito's argument matchers prove to be invaluable. Consider the following test case for a PaymentService
class that depends on a PaymentGateway
:
import org.junit.Test;
import static org.mockito.Mockito.*;
public class PaymentServiceTest {
@Test
public void testProcessPayment() {
PaymentGateway paymentGateway = mock(PaymentGateway.class);
PaymentService paymentService = new PaymentService(paymentGateway);
// Define expectations using argument matchers
when(paymentGateway.processPayment(anyString(), anyDouble())).thenReturn(true);
// Execute the method under test
boolean success = paymentService.processPayment("1234567890", 100.00);
// Verify interactions using argument matchers
verify(paymentGateway).processPayment(eq("1234567890"), doubleThat(closeTo(100.00, 0.001)));
assertTrue(success);
}
}
In this example, we use argument matchers such as anyString
, anyDouble
, eq
, and doubleThat
to define flexible expectations and verify method invocations with precise arguments.
Final Considerations
In conclusion, matchers play a crucial role in writing effective unit tests in Java. By leveraging the power of matchers, developers can create expressive, maintainable, and robust tests that accurately capture the intent of the assertions. Whether it's using Hamcrest matchers for descriptive assertions or Mockito's argument matchers for verifying interactions with external dependencies, mastering matchers is key to solving common testing challenges and elevating the quality of your test suite.
In your own testing endeavors, remember to explore the rich capabilities of matchers provided by Java testing frameworks, and don't hesitate to create custom matchers when the need arises. With the right use of matchers, you can conquer common testing challenges and unlock the full potential of your unit testing efforts.
For further reading on matchers and testing in Java, consider exploring the official documentation for Hamcrest and Mockito, as well as diving into advanced topics such as custom matcher creation and best practices for leveraging matchers effectively. Happy testing!