Master Spring WS: Solving Contract-First Approach Hurdles
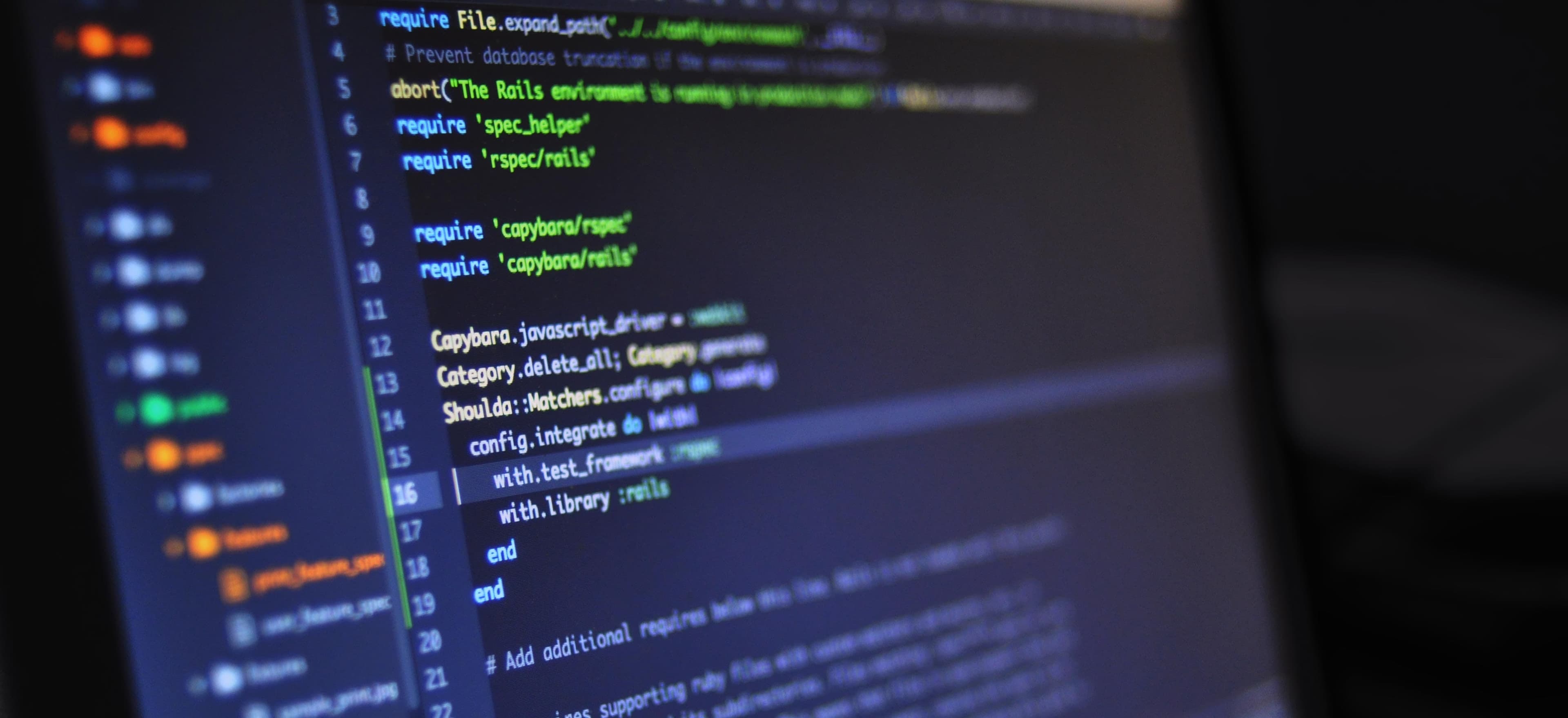
- Published on
Master Spring WS: Solving Contract-First Approach Hurdles
When it comes to building web services, choosing the right framework is crucial. Java developers often opt for Spring Web Services (Spring WS) due to its robust features, ease of use, and seamless integration with other Spring modules. In this blog post, we’ll delve into the world of Spring WS, specifically focusing on how it addresses the challenges of the contract-first approach in web service development.
Understanding the Contract-First Approach
In the context of web services, the contract-first approach emphasizes defining the service contract (WSDL - Web Services Description Language) before writing any implementation code. This ensures that the web service interface is independent of the underlying implementation, promoting loose coupling and interoperability.
Challenges of Contract-First Approach
While the contract-first approach offers numerous benefits, it also presents certain challenges, such as:
-
Manual Synchronization: Keeping the WSDL and the Java code in sync manually can be error-prone and labor-intensive.
-
Complex Configuration: Configuring and integrating the WSDL with the Java code can be complex and time-consuming.
-
Lack of Tooling Support: Traditional Java tools may not provide adequate support for generating code from an existing WSDL.
Enter Spring Web Services
Spring WS provides a comprehensive solution for addressing the challenges associated with the contract-first approach. Let’s explore how Spring WS alleviates these hurdles.
Simplified Configuration
Spring WS simplifies the configuration of web services and WSDL by providing dedicated XML namespaces and tags. This allows developers to define the web service endpoints, message senders, and message receivers concisely. Here's an example of how Spring WS simplifies the configuration:
<sws:annotation-driven />
<bean class="org.springframework.ws.server.endpoint.mapping.PayloadRootQNameEndpointMapping">
<property name="interceptors">
<bean class="org.springframework.ws.soap.server.endpoint.interceptor.PayloadValidatingInterceptor">
<property name="xsdSchema" ref="schema" />
<property name="validateRequest" value="true" />
<property name="validateResponse" value="true" />
</bean>
</property>
</bean>
In this snippet, the sws:annotation-driven
element enables Spring WS annotation-driven support, while the PayloadRootQNameEndpointMapping
and PayloadValidatingInterceptor
bean configurations simplify endpoint mapping and payload validation.
Contract-First Code Generation
Spring WS integrates seamlessly with industry-standard tools like Apache CXF or JAX-WS, allowing developers to generate Java code from an existing WSDL. This eliminates the need for manual synchronization and ensures that the Java code remains in sync with the WSDL. Here's an example of how Spring WS facilitates contract-first code generation:
@Bean
public Jaxb2Marshaller marshaller() {
Jaxb2Marshaller marshaller = new Jaxb2Marshaller();
marshaller.setContextPath("com.example.wsdl");
return marshaller;
}
In this example, the Jaxb2Marshaller
bean is configured to marshal and unmarshal XML to Java objects using JAXB, based on the specified context path, which corresponds to the package where the generated classes reside.
Interoperability and Message Transformation
Spring WS empowers developers to handle message transformations and interoperability concerns seamlessly. It provides powerful abstractions for working with XML payloads, allowing for XML validation, transformation, and manipulation. Here's an example of how Spring WS simplifies message transformation:
@Endpoint
public class OrderEndpoint {
private static final String NAMESPACE_URI = "http://www.example.com/orders";
@PayloadRoot(namespace = NAMESPACE_URI, localPart = "orderRequest")
@ResponsePayload
public JAXBElement<OrderResponse> processOrder(@RequestPayload JAXBElement<OrderRequest> request) {
// Process the order and generate a response
}
}
In this code snippet, the OrderEndpoint
class defines an endpoint for processing orders. By annotating the processOrder
method with @PayloadRoot
and @ResponsePayload
, Spring WS automates the message transformation process, handling XML payloads transparently.
Final Thoughts
In the realm of web service development, the contract-first approach remains a compelling paradigm for promoting service interoperability and loose coupling. Spring WS adeptly tackles the challenges associated with the contract-first approach, offering streamlined configuration, contract-first code generation, and seamless interoperability. By leveraging the power of Spring WS, Java developers can master the intricacies of web service development while adhering to best practices and industry standards.
Mastering Spring WS entails not only understanding its features and capabilities but also grasping the underlying principles of contract-first web service development. With Spring WS as a stalwart ally, developers can navigate the complexities of web service development with confidence and precision.
In conclusion, Spring WS stands as a potent ally for Java developers seeking to surmount the hurdles of the contract-first approach in web service development. By harnessing the capabilities of Spring WS, developers can propel their web service projects to new heights, armed with robustness, interoperability, and adherence to best practices. Embrace Spring WS, master the contract-first approach, and revolutionize your web service development journey.
So, are you ready to unleash the full potential of Spring WS and conquer the challenges of the contract-first approach? Your journey awaits, filled with innovation, seamless integration, and unparalleled mastery of web service development. Sharpen your skills, embrace the power of Spring WS, and embark on a transformative odyssey in the realm of web service development.