Supercharge Your Enums: Dual-Method Extension Magic!
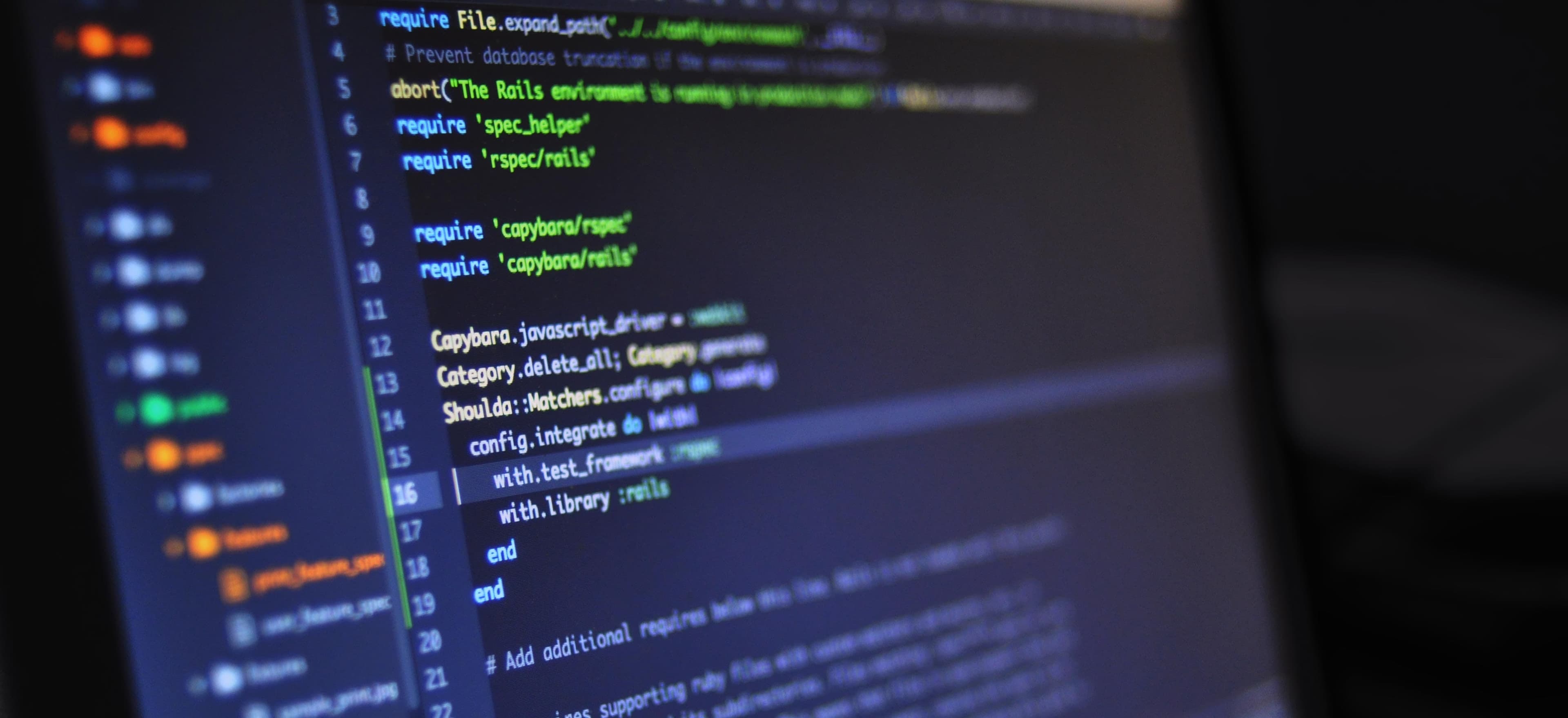
- Published on
Supercharge Your Enums: Dual-Method Extension Magic!
Enums are a powerful and versatile feature in Java. They allow us to define a set of constants in a more type-safe and organized manner. However, their capabilities can be further enhanced by using dual-method extension, a technique that can unlock the full potential of enums.
In this article, we'll explore how to supercharge your enums in Java by leveraging dual-method extension. We'll start by understanding the basics of enums and then gradually dive into dual-method extension, providing practical examples to illustrate its benefits.
Understanding Enums in Java
In Java, an enum type is a special data type that enables a variable to be a set of predefined constants. Enums are declared using the enum
keyword, and each constant value is separated by commas. Here's a basic example of an enum representing days of the week:
public enum Day {
MONDAY, TUESDAY, WEDNESDAY, THURSDAY, FRIDAY, SATURDAY, SUNDAY
}
Enums can also have fields, constructors, and methods like a regular class. They can be used in switch statements and can have associated behavior.
Leveraging Dual-Method Extension
Dual-method extension is a technique that extends the behavior of enums by adding methods that can be invoked on individual enum constants. This approach allows us to associate behavior with each enum constant, making the code more modular and easier to maintain.
Let's dive deeper into dual-method extension and how it can be implemented to supercharge your enums.
Implementing Dual-Method Extension
To implement dual-method extension, we'll define an interface with default methods and have the enum implement this interface. This enables each enum constant to provide its own implementation for the interface methods.
Here's an example of an interface Operation
with a default method calculate
:
public interface Operation {
default int calculate(int x, int y) {
return 0;
}
}
We can then define an enum BasicOperation
that implements the Operation
interface and provides a different implementation for the calculate
method for each constant:
public enum BasicOperation implements Operation {
ADD {
@Override
public int calculate(int x, int y) {
return x + y;
}
},
SUBTRACT {
@Override
public int calculate(int x, int y) {
return x - y;
}
},
MULTIPLY {
@Override
public int calculate(int x, int y) {
return x * y;
}
},
DIVIDE {
@Override
public int calculate(int x, int y) {
if (y != 0) {
return x / y;
} else {
throw new IllegalArgumentException("Cannot divide by zero");
}
}
}
}
In this example, each enum constant (ADD, SUBTRACT, MULTIPLY, DIVIDE) provides its own implementation for the calculate
method, allowing us to perform different operations based on the enum constant.
Benefits of Dual-Method Extension
Dual-method extension offers several benefits when applied to enums:
Modular and Extensible
By using dual-method extension, each enum constant encapsulates its behavior, making the code modular and easier to extend. If new behavior needs to be added, it can be done individually for each enum constant without affecting the others.
Readable and Maintainable
Dual-method extension promotes cleaner and more readable code by associating behavior directly with enum constants. This makes it easier for developers to understand and maintain the codebase.
Type Safety
Enums already provide type safety by restricting the set of valid constants. Dual-method extension further enhances type safety by ensuring that each constant provides the necessary behavior, reducing the chances of unexpected runtime errors.
Practical Use Cases
Dual-method extension can be applied to a wide range of use cases. Here are some practical examples where dual-method extension can be leveraged to enhance the functionality of enums:
Currency Conversion
An enum representing different currencies could implement a dual-method extension to perform currency conversion based on the selected currency.
public enum Currency {
USD {
@Override
public double convertToUSD(double amount) {
return amount;
}
},
EUR {
@Override
public double convertToUSD(double amount) {
return amount * 1.17;
}
},
GBP {
@Override
public double convertToUSD(double amount) {
return amount * 1.34;
}
};
public abstract double convertToUSD(double amount);
}
HTTP Response Handling
An enum representing different HTTP status codes could implement a dual-method extension to handle specific actions for each status code.
public enum HttpStatus {
OK {
@Override
public void handleResponse() {
// Handle OK response
}
},
NOT_FOUND {
@Override
public void handleResponse() {
// Handle NOT_FOUND response
}
},
SERVER_ERROR {
@Override
public void handleResponse() {
// Handle SERVER_ERROR response
}
};
public abstract void handleResponse();
}
The Closing Argument
Dual-method extension is a powerful technique that can significantly enhance the functionality of enums in Java. By associating behavior with individual enum constants, dual-method extension promotes modularity, readability, and type safety, making the code more maintainable and extensible.
Incorporating dual-method extension in your enum designs can lead to more robust and flexible code, especially when dealing with a set of related constants with distinct behavior.
Give it a try in your next project and experience the magic of supercharged enums with dual-method extension! Your code will thank you.
For more information on enums and dual-method extension, refer to the official Java documentation and various tutorials.