Stop Maven Woes: Exclude All Transitive Dependencies Now!
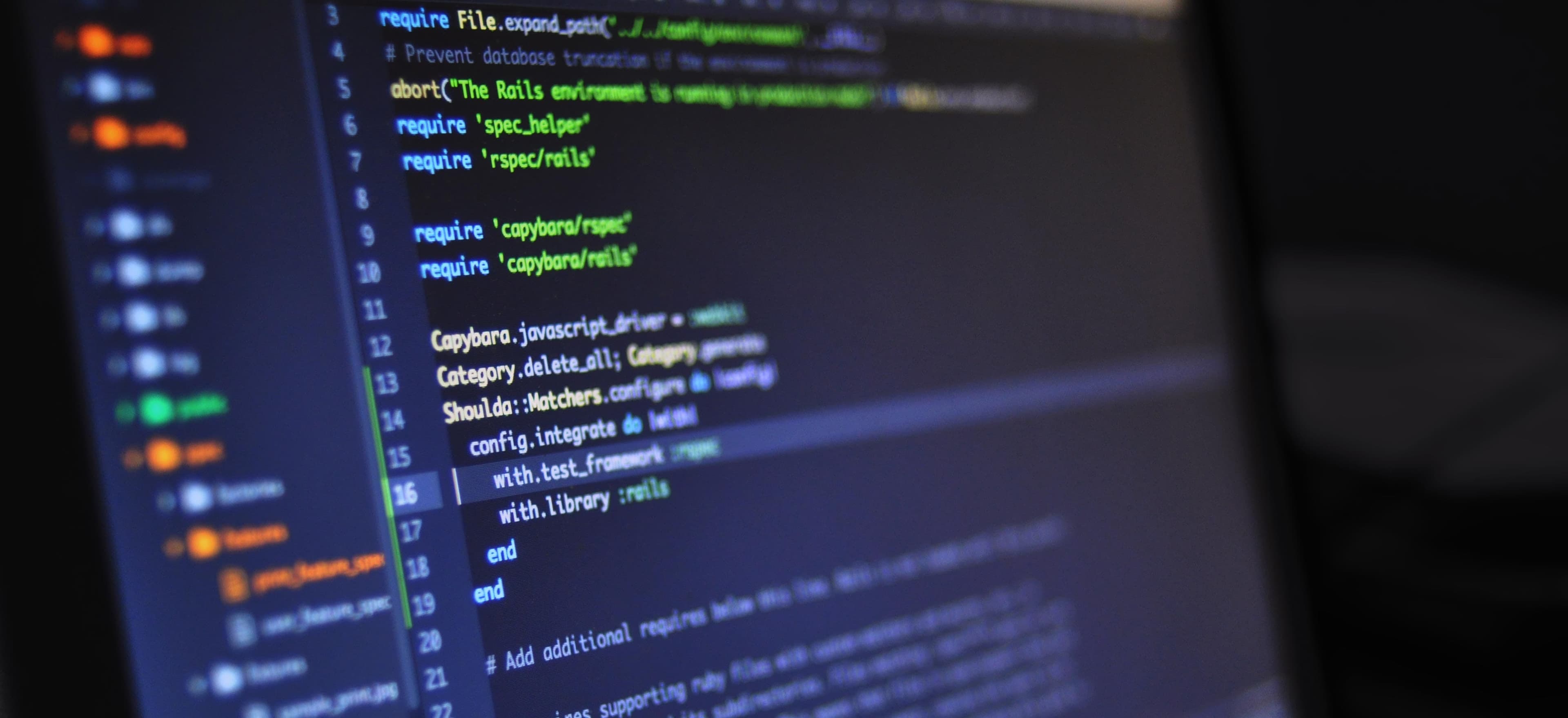
- Published on
Stop Maven Woes: Exclude All Transitive Dependencies Now!
Maven is a powerful build management tool used primarily for Java projects. However, one common issue that many developers encounter when using Maven is dealing with transitive dependencies.
Transitive dependencies are the dependencies of your project's dependencies. Sometimes, these transitive dependencies can cause conflicts or issues within your project, leading to unexpected behavior or errors. Fortunately, Maven provides a way to exclude transitive dependencies so you can have more control over the dependencies used in your project.
In this article, we will explore how to exclude all transitive dependencies in Maven, allowing you to effectively manage your project's dependencies and alleviate any related headaches.
Understanding Transitive Dependencies
Imagine you have a project that depends on a library called my-library
, which has its own dependencies, such as dependency-a
and dependency-b
. If dependency-a
also depends on dependency-b
, then dependency-b
becomes a transitive dependency of your project.
While transitive dependencies are helpful in resolving and managing complex dependencies automatically, they can also introduce conflicts or unwanted versions of libraries into your project.
Excluding Transitive Dependencies in Maven
Excluding transitive dependencies in Maven involves using the <exclusions>
tag within the <dependency>
tag. This tag allows you to exclude specific transitive dependencies, providing greater control over the dependencies used in your project.
Let's consider an example where we want to exclude all transitive dependencies for a specific dependency in our project. For this purpose, we will exclude all transitive dependencies for the my-library
in the following steps.
Step 1: Locate the Dependency in Your POM.xml
First, you need to locate the dependency for which you want to exclude all transitive dependencies in your project's pom.xml
file. Find the <dependency>
tag for the my-library
in the <dependencies>
section.
Step 2: Add Exclusion Configuration
Once you have located the <dependency>
tag for my-library
, you can add the <exclusions>
tag within it to exclude all transitive dependencies. The <exclusions>
tag includes one or more <exclusion>
tags, each specifying the groupId
and artifactId
of a transitive dependency to exclude.
Here is an example of how to exclude all transitive dependencies for the my-library
dependency:
<dependency>
<groupId>com.example</groupId>
<artifactId>my-library</artifactId>
<version>1.0.0</version>
<exclusions>
<exclusion>
<groupId>*</groupId>
<artifactId>*</artifactId>
</exclusion>
</exclusions>
</dependency>
In this example, the <exclusion>
tag with <groupId>*</groupId>
and <artifactId>*</artifactId>
ensures that all transitive dependencies of my-library
are excluded.
Step 3: Update and Validate
After adding the exclusion configuration, save your pom.xml
file. Then, run a Maven build to ensure that the transitive dependencies are excluded as expected. You can use the mvn clean install
command to perform a clean build and validate the exclusion of transitive dependencies.
By following these steps, you can effectively exclude all transitive dependencies for a specific dependency in your Maven project.
Why Exclude All Transitive Dependencies?
You might wonder why you would want to exclude all transitive dependencies in the first place. Let's explore some scenarios where excluding transitive dependencies can be beneficial.
Preventing Conflict Resolution Issues
In some cases, a transitive dependency might conflict with another existing dependency in your project, leading to resolution issues. By excluding all transitive dependencies, you can avoid these conflicts and ensure that only the explicitly declared dependencies are used.
Managing Dependency Bloat
Transitive dependencies can sometimes introduce unnecessary libraries into your project, leading to dependency bloat. This bloat can increase the size of your project and potentially introduce unnecessary complexities. By excluding all transitive dependencies, you can keep your project lean and focused on essential dependencies.
Maintaining Control Over Dependencies
Excluding all transitive dependencies gives you more granular control over the libraries used in your project. You can explicitly declare only the necessary dependencies without worrying about unexpected transitive dependencies interfering with your project.
Considerations and Best Practices
While excluding all transitive dependencies can be useful, it's essential to consider the following best practices and considerations:
Understand the Implications
Excluding all transitive dependencies should be approached with caution. Ensure that you fully understand the implications of excluding specific transitive dependencies, as doing so might lead to unexpected runtime errors or missing functionality.
Use with Discretion
Excluding all transitive dependencies should be used judiciously. Consider the necessity of excluding all transitive dependencies for a specific dependency, and analyze the potential impact on your project before making the exclusion.
Document Exclusions
If you choose to exclude all transitive dependencies for a particular dependency, document the reasons for doing so in your project's documentation. This documentation helps maintain clarity and informs other developers about the intentional exclusions.
Consider Dependency Management Tools
In some cases, using dependency management tools such as the Maven Dependency Plugin can provide a more systematic approach to managing dependencies, including transitive dependencies. Evaluate the use of such tools before opting to exclude all transitive dependencies.
Bringing It All Together
Excluding all transitive dependencies in Maven can be a powerful tool for managing and controlling the dependencies in your project. By following the outlined steps, understanding the use cases, and considering best practices, you can effectively exclude all transitive dependencies where necessary, ensuring a more predictable and streamlined dependency resolution process.
Remember, while excluding transitive dependencies can resolve specific issues, it should be approached thoughtfully and with a clear understanding of its implications. With a well-managed approach, you can streamline your Maven project's dependencies and ensure a more controlled and efficient build process.