Solving Java Module System Mysteries: A Beginner's Guide
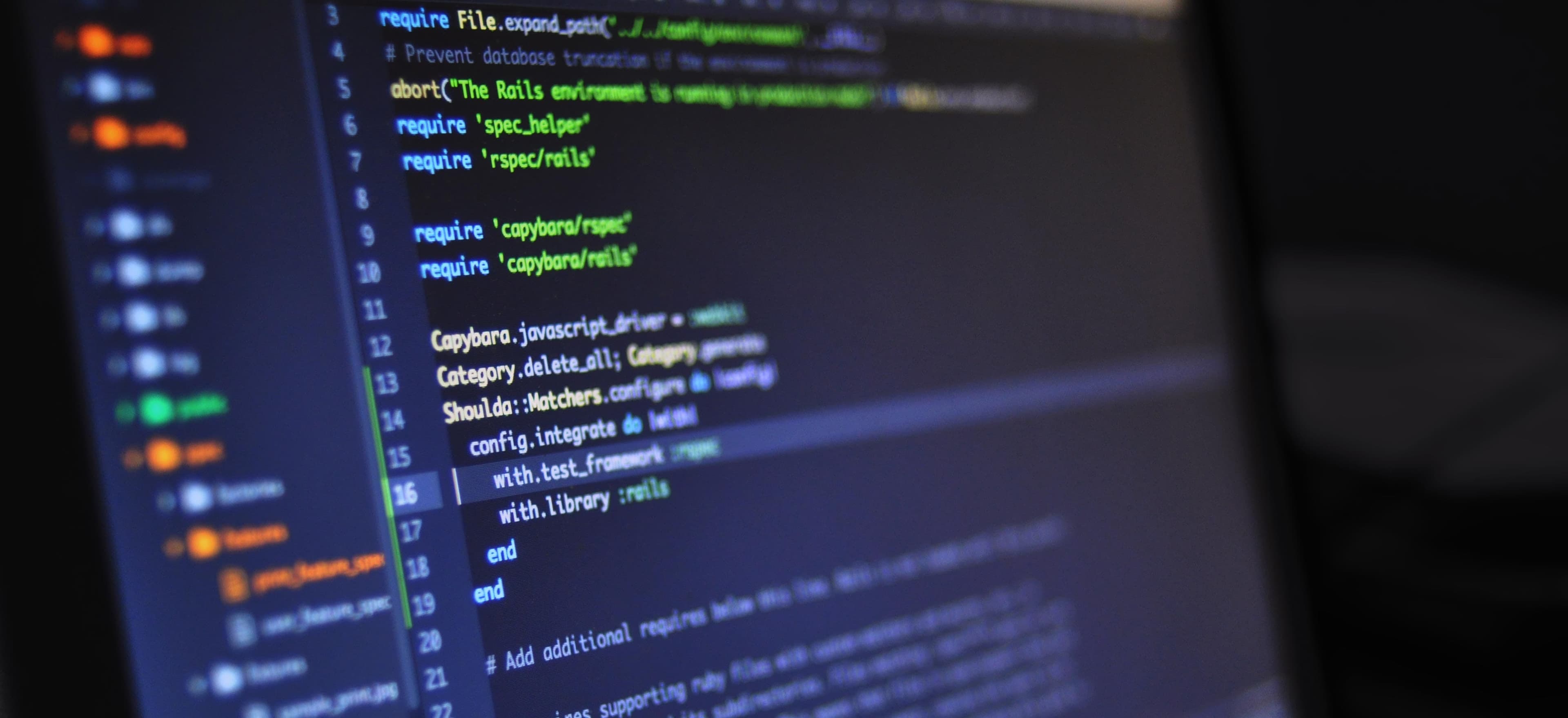
- Published on
Solving Java Module System Mysteries: A Beginner's Guide
Java, a versatile and widely-used programming language, has continued to evolve over the years to meet the demands of modern software development. One of the significant evolutionary leaps was the introduction of the Java Module System with Java 9. In this beginner's guide, we will navigate through the Java Module System, demystifying its key concepts and providing practical guidance for beginners to get started. Whether you are new to Java or experienced in earlier versions, grasping the Java Module System is essential for building reliable, scalable, and maintainable applications.
What is the Java Module System?
The Java Module System, introduced in Java 9, brings modularity to Java, addressing long-standing issues like JAR Hell and enhancing code encapsulation and maintainability. Traditionally, Java applications were organized in JAR (Java Archive) files and relied on classpath for dependencies, often leading to conflicting versions and poorly encapsulated code. The Module System aims to solve these problems by allowing developers to define explicit module dependencies and encapsulate code within modules, resulting in more robust and maintainable applications.
Getting Started with Modules
Creating Your First Module
To create a Java module, we start by setting up the module directory structure and defining a module descriptor. Let's create a simple module named HelloWorldModule
with a HelloWorld
class.
// module-info.java
module HelloWorldModule {
// Define module dependencies here
}
Now, let's create a simple HelloWorld
class within the module:
// HelloWorld.java
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
Compiling and Running Your Module
Once the module and its class are created, we can compile the module using the javac
command and run the compiled module with the java
command.
To compile the module:
javac -d <module_output_directory> <module_source_directory>/module-info.java <module_source_directory>/HelloWorld.java
javac
: The Java compiler command-d <module_output_directory>
: Specifies the output directory for the compiled module<module_source_directory>/module-info.java <module_source_directory>/HelloWorld.java
: Path to the module source files
Once compiled, we can run the module using:
java --module-path <module_output_directory> -m HelloWorldModule/HelloWorld
--module-path <module_output_directory>
: Specifies the directory containing the compiled module-m HelloWorldModule/HelloWorld
: Indicates the module and main class to be executed
With these steps, you have created, compiled, and run your first Java module!
Key Concepts of the Java Module System
Module Path vs. Classpath
In the Java Module System, module dependencies are resolved through the module path instead of the traditional classpath. The module path specifies the location of modules, and the JVM resolves module dependencies based on this path. This provides a more robust and explicit way of managing dependencies compared to the classpath, reducing the likelihood of conflicts and version discrepancies.
Exports and Requires
The module-info.java
file defines module dependencies using the requires
directive and exposes packages to other modules using the exports
directive. This allows for explicit control over module relationships and encapsulation, enhancing the maintainability and reliability of the codebase.
// module-info.java
module HelloWorldModule {
requires someOtherModule;
exports com.example.helloworld;
}
In this example, the HelloWorldModule
requires someOtherModule
and exposes the com.example.helloworld
package to other modules.
Modular JDK
With Java 9, even the JDK itself has transitioned into a modular form. The JDK is now organized into a set of modules, each serving a specific purpose. Some key modules within the JDK include java.base
, java.sql
, java.logging
, and many more. This modularization has improved the maintainability and scalability of the JDK itself, setting the stage for more efficient and manageable Java applications.
Advanced Topics
Services in Modules
Modules also allow the definition of services using the provides
and uses
directives in the module-info.java
file. This enables the creation of extensible and pluggable architectures within Java applications by defining and consuming services provided by other modules.
// module-info.java
module HelloWorldModule {
provides com.example.service.SomeServiceInterface with com.example.service.impl.SomeServiceImpl;
uses com.example.service.SomeServiceInterface;
}
Reflection and Modules
The introduction of the Java Module System also had implications for Java Reflection. With modules, access to reflective operations is restricted by default. This imposes some challenges when working with libraries or frameworks that heavily rely on reflection. However, by expertly configuring the module descriptor and using appropriate command-line options, these challenges can be overcome, allowing for the effective use of reflection in a modular environment.
Lessons Learned
The Java Module System has brought about a paradigm shift in Java development, addressing long-standing issues and providing a more structured, maintainable approach to building applications. As a beginner, getting acquainted with the Java Module System sets a strong foundation for writing robust and scalable Java applications. Experiment with creating and connecting modules to gain a deeper understanding of these concepts. Whether you are building a small application or a large-scale system, the Java Module System empowers you to manage dependencies and encapsulate code effectively.
The evolution of Java continues, and understanding the principles and practices of modularity in Java 9 is the first step towards becoming a proficient Java developer. Remember, the knowledge gained from Java 9's Module System remains relevant and applicable to later versions of Java, making it a valuable skill to master in the ever-changing landscape of software development.
Coding examples and illustrations are vital for learning, and they make understanding complex topics like the Java Module System more manageable. If you are passionate about exploring more about Java modules, refer to the official Oracle documentation on the Java Module System for in-depth understanding.
Call to Action
If you found this guide helpful or have questions, share your experiences and thoughts in the comments section below. Stay tuned for more exciting Java-related content by subscribing to our blog. Let's continue exploring Java together!
In this article, we have delved into the world of Java modules, breaking down complex concepts into beginner-friendly language and providing practical examples. The Java Module System is a crucial advancement in Java's evolution, and we hope this guide has demystified its key aspects, propelling your journey into the exciting world of modular Java development.