Mastering Asserts in Java: Dive into Hamcrest Matchers
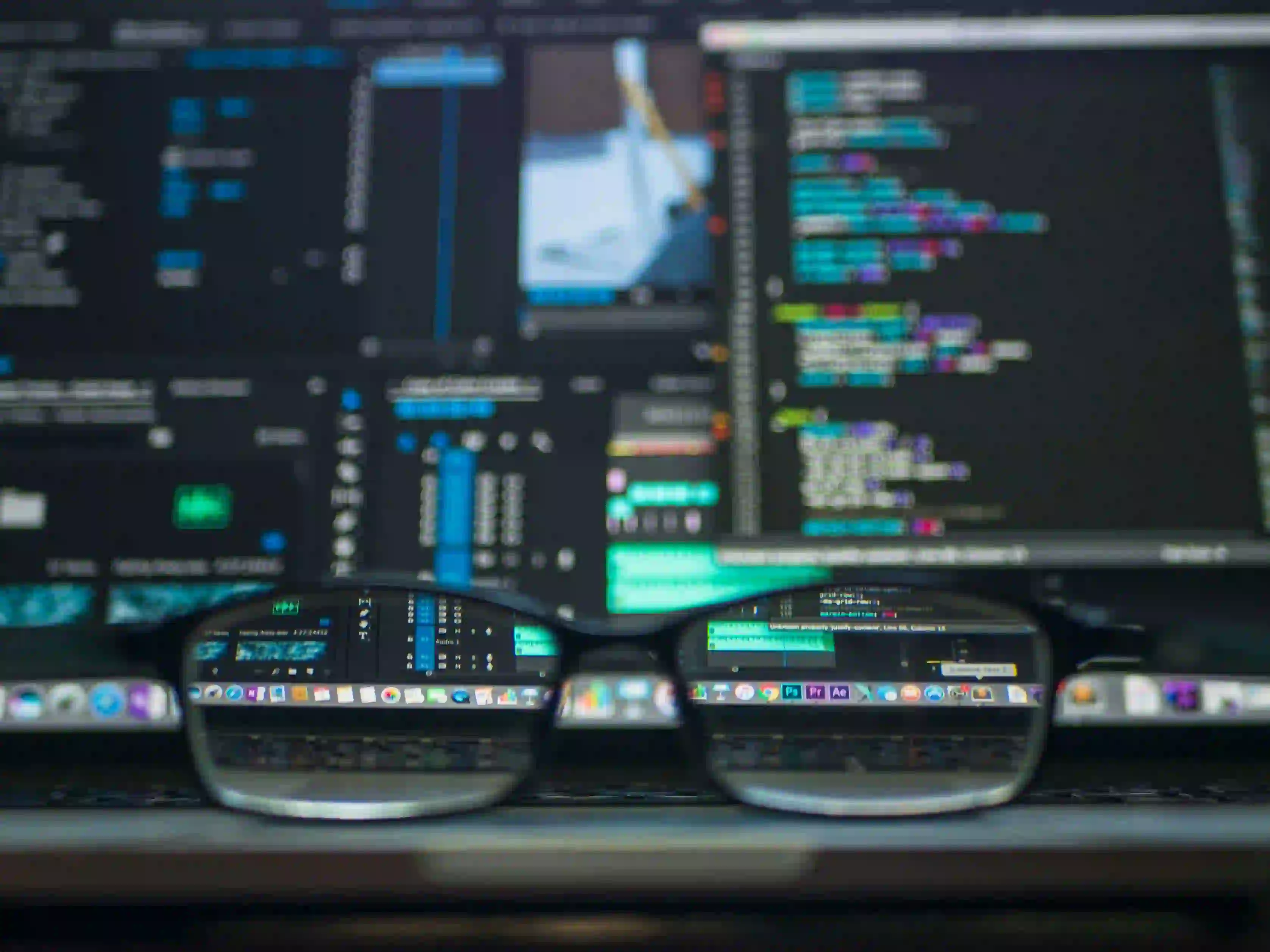
Mastering Asserts in Java: Dive into Hamcrest Matchers
When writing unit tests in Java, using meaningful and expressive assertions is crucial for writing maintainable and readable tests. This is where Hamcrest comes into play. Hamcrest is a framework that provides a rich set of matchers, allowing you to write assertions in a more fluent and expressive way compared to the standard assertions provided by JUnit.
In this article, we will explore the power of Hamcrest matchers and how they can improve the quality and readability of your tests.
What are Hamcrest Matchers?
Hamcrest matchers provide a way to write assertions that are more readable and expressive. They are designed to be composable, allowing you to create complex assertions by combining simple matchers using logical operations.
For example, consider a simple assertion to check if a list contains a specific item:
// Standard JUnit assertion
assertTrue(list.contains(item));
// Using Hamcrest
assertThat(list, hasItem(item));
The Hamcrest version reads more like a sentence, making it easier to understand the intent of the assertion.
Getting Started with Hamcrest
To start using Hamcrest in your Java project, you'll need to include the Hamcrest library as a dependency. If you're using Maven, you can add the following dependency to your pom.xml
:
<dependency>
<groupId>org.hamcrest</groupId>
<artifactId>hamcrest-library</artifactId>
<version>2.2</version>
<scope>test</scope>
</dependency>
With the dependency added, you can start using Hamcrest matchers in your tests.
Using Hamcrest Matchers
Hamcrest provides a wide range of matchers for various types of assertions. Here are some commonly used matchers and their respective use cases:
Equality Matchers
equalTo
– Matches if the object is equal to another using theequals
method.is
– An alias forequalTo
.not
– Matches if the value is not equal to a given value.
// Example using equalTo
assertThat(10, equalTo(10));
// Example using is (alias for equalTo)
assertThat("Hello", is("Hello"));
// Example using not
assertThat(5, not(equalTo(10)));
Collection Matchers
hasItem
– Matches if the given collection contains the specified item.hasItems
– Matches if all specified items are present in the given collection.hasSize
– Matches if the collection has a specific size.
// Example using hasItem
List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
assertThat(names, hasItem("Bob"));
// Example using hasItems
assertThat(names, hasItems("Alice", "Charlie"));
// Example using hasSize
assertThat(names, hasSize(3));
String Matchers
containsString
– Matches if the string contains a specified substring.startsWith
– Matches if the string starts with a specified prefix.endsWith
– Matches if the string ends with a specified suffix.
// Example using containsString
String message = "Hello, world!";
assertThat(message, containsString("world"));
// Example using startsWith
assertThat(message, startsWith("Hello"));
// Example using endsWith
assertThat(message, endsWith("!"));
These are just a few examples of the many matchers provided by Hamcrest. Using these matchers not only makes your tests more expressive but also provides more detailed failure messages when an assertion fails.
Creating Custom Matchers
While Hamcrest provides a rich set of built-in matchers, there may be cases where you need to create custom matchers to express specific domain logic in your tests. Fortunately, Hamcrest allows you to create custom matchers easily.
Here's an example of a custom matcher that checks if a string is a palindrome:
import org.hamcrest.Description;
import org.hamcrest.TypeSafeMatcher;
public class IsPalindrome extends TypeSafeMatcher<String> {
@Override
public void describeTo(Description description) {
description.appendText("a palindrome");
}
@Override
protected boolean matchesSafely(String str) {
return str.equals(new StringBuilder(str).reverse().toString());
}
public static IsPalindrome palindrome() {
return new IsPalindrome();
}
}
You can then use the custom matcher in your tests:
String palindrome = "deified";
assertThat(palindrome, is(palindrome()));
Creating custom matchers allows you to encapsulate complex logic and make your tests more expressive and readable.
Combining Matchers
One of the powerful features of Hamcrest is the ability to combine matchers to create complex assertions. This allows you to express sophisticated conditions in a clear and readable manner.
For example, consider the following assertion that checks if a string is both a palindrome and has a length of 5:
assertThat("deified", allOf(is(palindrome()), hasLength(7)));
In this example, allOf
is a logical matcher that ensures all of its component matchers pass for it to succeed.
Key Takeaways
In this article, we've only scratched the surface of what Hamcrest can do to improve the readability and expressiveness of your assertions in Java. By using Hamcrest matchers, you can write tests that are not only robust but also easy to understand and maintain.
To dive deeper into Hamcrest, you can explore the complete list of matchers and their usage in the official Hamcrest documentation: Hamcrest Official Documentation
By mastering Hamcrest matchers, you can elevate the quality of your Java unit tests and ultimately contribute to the reliability and maintainability of your codebase.