Transform Your Code: From Procedural to Object-Oriented Magic
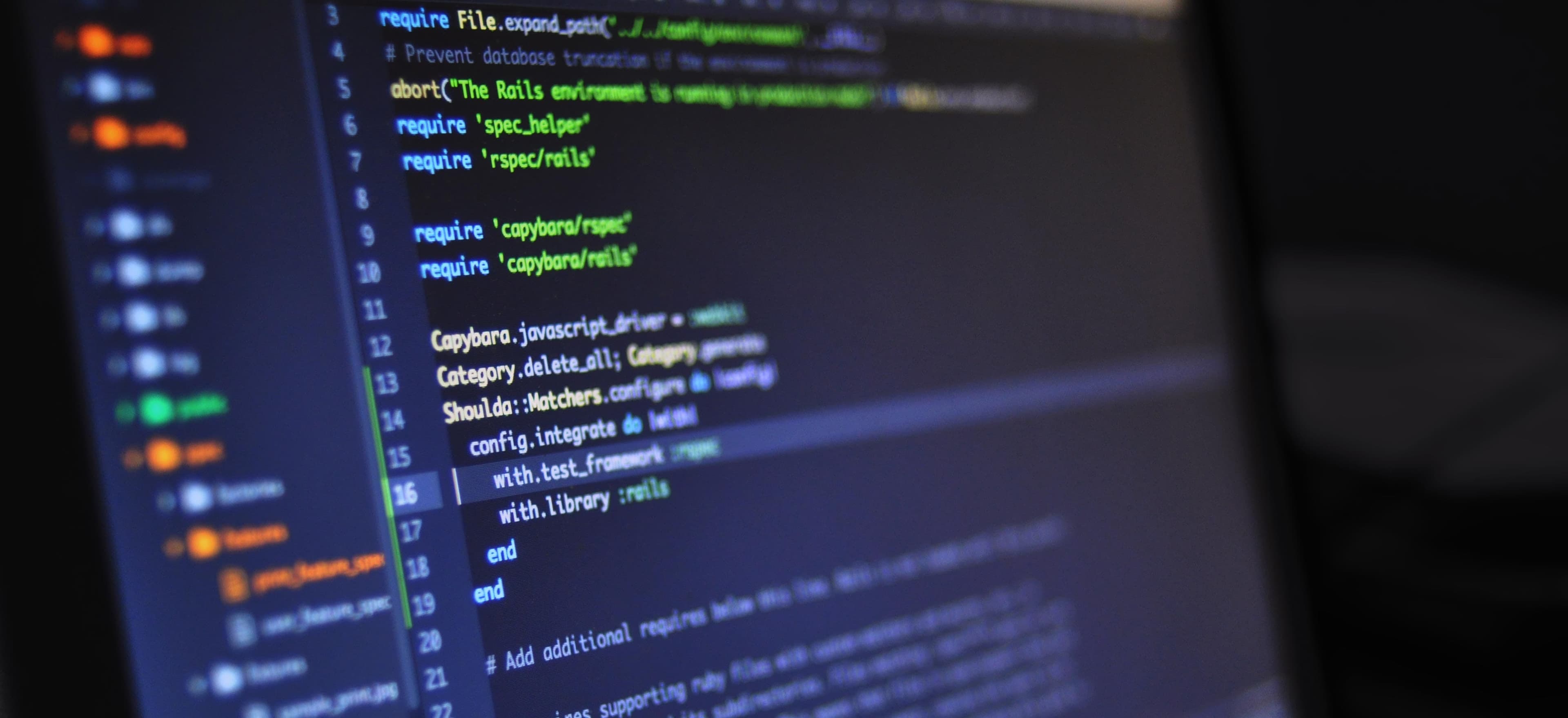
- Published on
Transform Your Code: From Procedural to Object-Oriented Magic
Are you a Java developer looking to level up your coding game? Have you been writing procedural code and have heard about the wonders of object-oriented programming (OOP)? Look no further, as we delve into the world of transforming procedural Java code into elegant, efficient, and maintainable object-oriented code.
Understanding the Procedural vs. Object-Oriented Paradigm
Let's first understand the fundamental differences between procedural and object-oriented programming paradigms. Procedural programming is centered around procedures or functions, where the focus is on the step-by-step execution of code. On the other hand, OOP emphasizes the concept of objects, allowing for the organization of code into reusable and modular units.
The Power of Objects in Java
Java, as an object-oriented language, provides powerful capabilities for creating and manipulating objects. Objects in Java encapsulate data and behavior, promoting concepts such as encapsulation, inheritance, and polymorphism.
Restructuring Procedural Code into Object-Oriented Code
Identifying Entities and Actions
When transforming procedural code into an object-oriented structure, start by identifying the entities and the actions associated with them. For example, if you have procedural code for managing employees, you might identify the entities as 'Employee' and 'Department' and actions such as 'hire', 'fire', and 'promote'.
Creating Classes
In the OOP paradigm, classes are blueprints for creating objects. Each class represents an entity with its attributes (data) and methods (behavior). Let's take the 'Employee' entity as an example and create an Employee
class.
public class Employee {
private String name;
private int employeeId;
private double salary;
// Constructor
public Employee(String name, int employeeId, double salary) {
this.name = name;
this.employeeId = employeeId;
this.salary = salary;
}
// Getters and setters
// ... (omitted for brevity)
// Other methods
// ...
}
Here, the Employee
class encapsulates the attributes of an employee (name, employee ID, and salary) and provides methods for interacting with these attributes.
Encapsulation and Access Modifiers
Encapsulation is a key principle in OOP, and Java provides access modifiers to control the visibility of classes, attributes, and methods. Utilize access modifiers such as public
, private
, and protected
to enforce encapsulation and maintain the integrity of your objects.
Leveraging Inheritance and Polymorphism
Inheritance allows one class to inherit attributes and methods from another, promoting code reusability and hierarchical organization. Polymorphism, another pillar of OOP, enables different classes to be treated as instances of the same class through method overriding and method overloading.
Refactoring Procedural Logic into Methods
In procedural code, logic is often scattered throughout the program. By refactoring this logic into methods within the appropriate classes, you can achieve a more structured and modular design. For instance, if you have procedural code for calculating employee bonus, consider refactoring it into a calculateBonus()
method within the Employee
class.
Utilizing Interfaces and Abstract Classes
Interfaces and abstract classes in Java provide mechanisms for defining contracts and creating hierarchies where common behavior can be shared among classes. When appropriate, introduce interfaces and abstract classes to abstract common behavior and enforce consistent implementation across related classes.
Handling Data Storage and Retrieval
In procedural programming, data storage and retrieval are often handled using disparate functions or modules. In an object-oriented approach, consider creating dedicated classes or interfaces for data access, such as a DataSource
interface for database interaction or a FileReader
class for file handling.
Embracing the Object-Oriented Mindset
Transforming procedural code into object-oriented code goes beyond mere syntax transformation. It requires embracing the object-oriented mindset, understanding the relationships between entities, and encapsulating behavior within objects.
Benefits of Object-Oriented Transformation
Reusability and Modularity
Object-oriented code promotes the reuse of classes and objects, resulting in a more modular and maintainable codebase. With inheritance and polymorphism, common behavior can be shared among classes, reducing redundancy and enhancing code flexibility.
Encapsulation and Abstraction
Encapsulation shields the complexity of an object's internal implementation, exposing only relevant information to the outside. Abstraction allows for the creation of well-defined interfaces, enabling the interaction with objects at a higher level of understanding.
Improved Maintainability and Extensibility
Object-oriented code tends to be more maintainable and extensible, as changes can be localized to specific classes or methods without affecting the entire codebase. This facilitates the addition of new features and the modification of existing behavior with reduced impact.
Enhanced Readability and Understandability
By structuring code around well-defined entities and their interactions, object-oriented code can be more readable and understandable. The use of meaningful class and method names, along with clear relationships between objects, contributes to code that is easier to comprehend.
A Final Look
The transformation from procedural to object-oriented code in Java is a journey that entails recognizing entities, creating classes, leveraging inheritance and polymorphism, and embracing the principles of OOP. Through this transformation, you can unlock the potential of reusability, maintainability, and extensibility, ultimately achieving code that exhibits the elegance and magic of object-oriented design.
So, are you ready to embrace the magic of object-oriented programming in Java? Start your journey today and watch your code transform into a masterpiece of modularity and elegance.
Check out Java's official documentation for further insights into the world of object-oriented programming in Java.
Happy coding!