Master KivaKit Docker Builds: Overcoming Common Hurdles
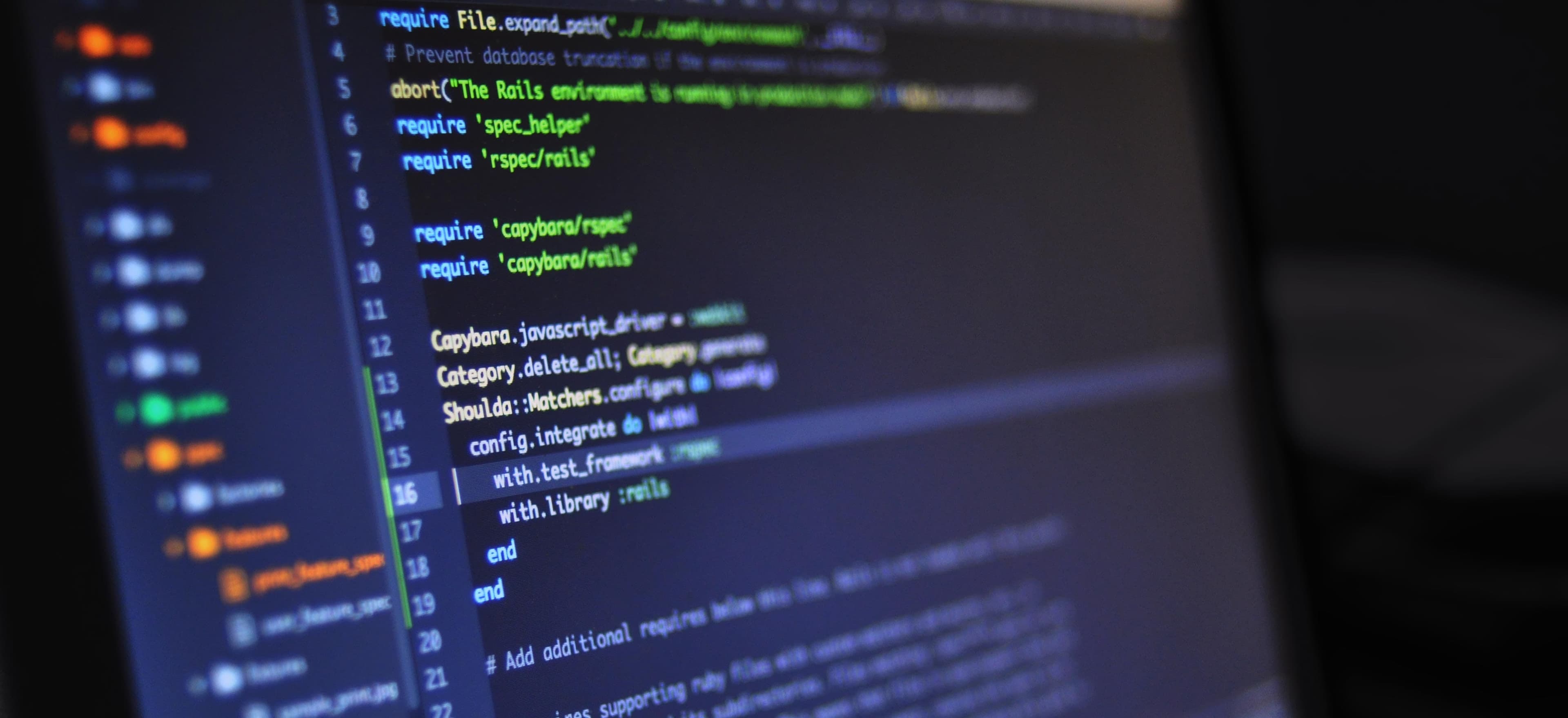
- Published on
Master KivaKit Docker Builds: Overcoming Common Hurdles
Docker has emerged as a leading technology for containerization, revolutionizing the way we develop, ship, and run applications. In the Java ecosystem, Docker plays a pivotal role in streamlining the build and deployment processes. In this blog post, we will delve into the best practices and strategies to master Docker builds in Java, addressing common hurdles along the way.
Understanding the Importance of Docker in Java Development
Docker provides an effective solution for packaging, distributing, and running applications in a consistent environment. For Java developers, Docker offers the ability to encapsulate Java applications and their dependencies into lightweight, portable containers. This not only ensures consistency across different environments but also simplifies the deployment workflow.
Now let's consider some common hurdles in Java Docker builds and how to overcome them.
1. Dealing with Multi-Stage Builds for Java Applications
One common challenge in Java Docker builds is managing multi-stage builds effectively. This is particularly important for Java applications, where the build process involves compilation, dependency resolution, and packaging.
Consider the following example of a multi-stage Dockerfile for a Java application:
# Stage 1: Build the application
FROM maven:3.6.3-jdk-11 AS builder
WORKDIR /app
COPY pom.xml .
COPY src ./src
RUN mvn package
# Stage 2: Create the final image
FROM adoptopenjdk:11-jre-hotspot
WORKDIR /app
COPY --from=builder /app/target/myapp.jar .
CMD ["java", "-jar", "myapp.jar"]
In this example, the first stage builds the application using Maven, while the second stage creates the final Docker image using the compiled artifacts. Utilizing multi-stage builds not only keeps the final image lean but also ensures security by discarding unnecessary build-time tools and dependencies.
2. Managing Java Dependencies and Caching
Dependency management is crucial in Java application development. When building Docker images, caching dependencies effectively can significantly improve build times. Utilizing Docker layer caching along with dependency management tools such as Maven or Gradle can greatly optimize build processes.
# Set up the base image
FROM maven:3.6.3-jdk-11 AS builder
WORKDIR /app
# Cache dependency downloads
COPY pom.xml .
RUN mvn dependency:go-offline
# Build the application
COPY src ./src
RUN mvn package
In this example, by copying the pom.xml
and running mvn dependency:go-offline
first, we can leverage Docker's layer caching mechanism to cache dependency downloads. This ensures that dependencies are only re-downloaded when the pom.xml
file changes, thus optimizing the build process.
3. Optimizing Docker Image Size for Java Applications
Java applications often suffer from large Docker image sizes due to the inclusion of unnecessary dependencies and artifacts. To optimize the image size, it's essential to carefully curate the contents of the image and minimize the layers. Utilizing tools like Jib or Buildpacks can streamline this process by automatically packaging the Java application without the need for writing Dockerfiles.
For instance, Jib allows you to build optimized Docker and OCI images without a Docker daemon, thereby reducing image size and build complexity. It achieves this by leveraging layers and choosing an appropriate base image for the Java application.
4. Handling Environment-specific Configurations
Java applications often require environment-specific configurations, such as database URLs, credentials, and external service endpoints. When containerizing Java applications, it's vital to externalize such configurations to enable flexibility and portability across diverse environments.
One approach to tackling this challenge is through the use of environment variables. By injecting environment-specific configurations during container runtime, the same Docker image can be deployed across various environments without modification.
# Use environment variables for configuration
FROM adoptopenjdk:11-jre-hotspot
WORKDIR /app
COPY target/myapp.jar .
CMD ["java", "-Ddatabase.url=$DATABASE_URL", "-jar", "myapp.jar"]
In this example, the database URL is injected as an environment variable at runtime, allowing the same Docker image to be deployed across different environments with varying database configurations.
To Wrap Things Up
Mastering Docker builds for Java applications is essential for efficient and consistent software delivery. By understanding the nuances of Java Docker builds and implementing best practices such as multi-stage builds, dependency caching, image size optimization, and environment configuration handling, developers can streamline the build and deployment processes while ensuring consistency and portability across diverse environments.
With the ever-evolving landscape of containerization and Java development, continuous learning and adaptation to new tools and best practices are imperative for Java developers to stay ahead in the game.
In conclusion, Docker plays a vital role in the Java ecosystem, and mastering Docker builds is crucial for streamlined and efficient software delivery.
Are you ready to conquer the world of Java Docker builds? Let's dive in!