Mastering OSGi: Turning Java Errors Into Learning Opportunities
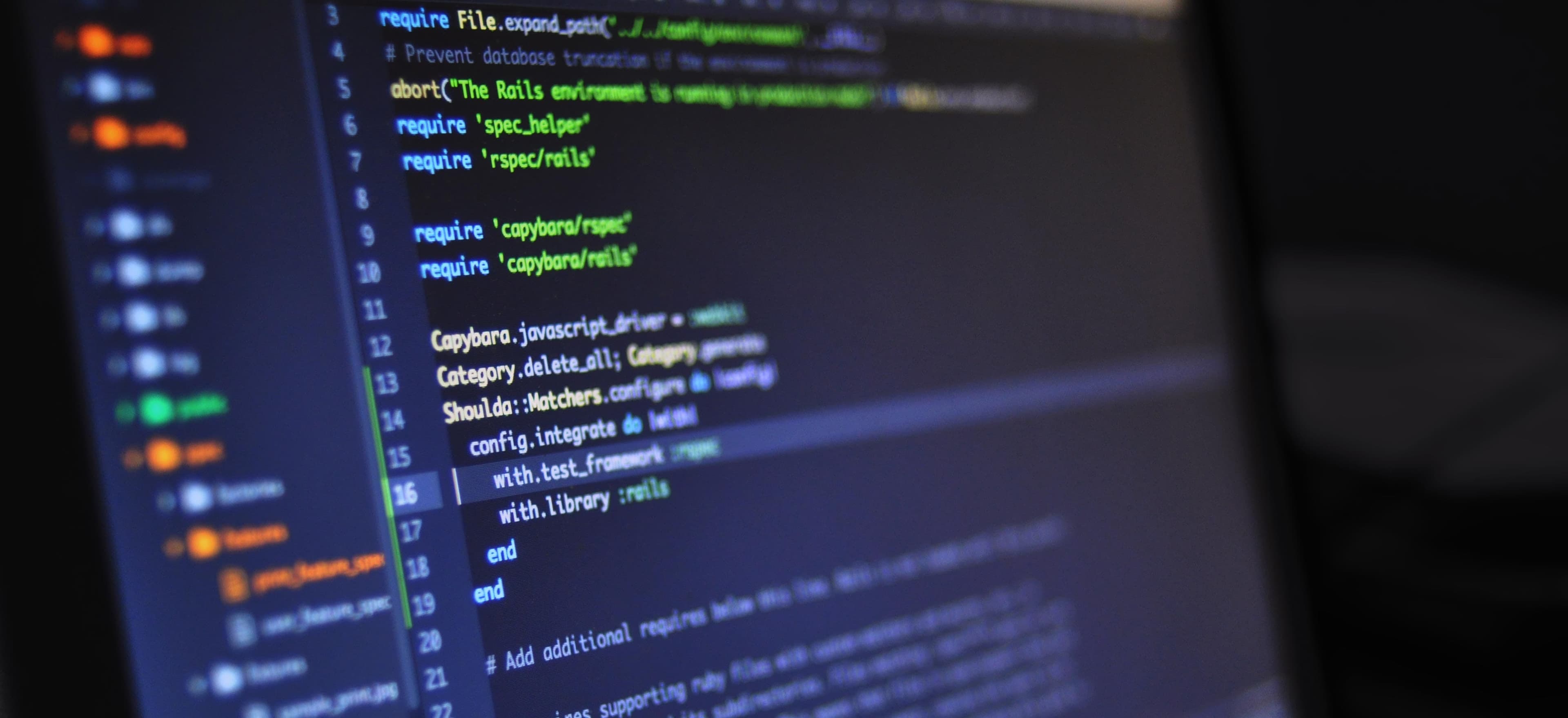
- Published on
Mastering OSGi: Turning Java Errors Into Learning Opportunities
The journey of a Java developer is full of challenges, and encountering errors is an integral part of this journey. One such challenge that Java developers often face is the complexity of managing and modularizing large-scale applications. This is where OSGi (Open Services Gateway initiative) comes into play. OSGi provides a dynamic module system for Java that enables developers to modularize their applications and effectively manage dependencies.
In this blog post, we will explore the world of OSGi, understand its significance, and delve into the process of turning Java errors into learning opportunities through the effective use of OSGi.
Understanding OSGi
What is OSGi?
OSGi is a dynamic module system for Java that allows developers to create modular applications. It provides a framework for building and deploying applications as a set of loosely-coupled, reusable, and dynamic modules known as bundles. These bundles can be installed, started, stopped, updated, and uninstalled dynamically without requiring a restart of the entire application.
Why OSGi?
- Modularity: OSGi promotes a modular architecture, allowing developers to divide their applications into smaller, manageable components.
- Dependency Management: OSGi provides a robust mechanism for handling dependencies between modules, ensuring that the application remains cohesive and resilient to changes.
- Dynamic Updates: OSGi enables dynamic updates and hot-swapping of modules, allowing for seamless upgrades and maintenance of running applications.
Dealing with Java Errors Using OSGi
Scenario: Classpath Hell
One common issue Java developers encounter is the dreaded "classpath hell" – managing dependencies, resolving conflicts, and avoiding version clashes. OSGi addresses this challenge by enforcing strong encapsulation and versioning of dependencies within bundles, thus eliminating classpath-related errors.
Code Example: OSGi Bundle Manifest
Let's take a look at a sample OSGi bundle manifest to understand how OSGi helps in resolving classpath-related issues:
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-SymbolicName: com.example.mybundle
Bundle-Version: 1.0.0
Import-Package: org.example.dependency;version="[1.0.0,2.0.0)"
In the above manifest, the Import-Package
header specifies the required dependency org.example.dependency
along with a version range. This ensures that the bundle will only resolve and use the specified version of the dependency, thereby avoiding classpath conflicts.
Scenario: Runtime Errors and Service Discovery
Another common challenge in Java development is dealing with runtime errors and ensuring dynamic service discovery within applications. OSGi's service-oriented architecture facilitates the registration, discovery, and consumption of services at runtime, thus mitigating the risk of runtime errors and enabling robust dynamic behavior.
Code Example: OSGi Service Registration
Let's consider a scenario where we need to register a service using OSGi:
public interface DataService {
// Service methods
}
@Component
public class DataServiceImpl implements DataService {
// Implementation of service methods
}
In the above code snippet, the DataServiceImpl
class is annotated as a component, indicating that it provides the DataService
service. OSGi's service registry will automatically register this service, making it available for dynamic discovery and consumption by other bundles at runtime.
Scenario: Versioning and Upgrades
Maintaining version consistency and ensuring smooth upgrades across various components of a Java application can be quite challenging. OSGi addresses this issue by offering a versioning mechanism that allows for coexistence of different versions of the same module within the same application, enabling graceful transitions and upgrades.
Code Example: OSGi Bundle Versioning
Consider a scenario where we need to define versioning for an OSGi bundle:
Manifest-Version: 1.0
Bundle-ManifestVersion: 2
Bundle-SymbolicName: com.example.mybundle
Bundle-Version: 1.0.0
In the manifest above, the Bundle-Version
header specifies the version of the OSGi bundle. OSGi's versioning mechanism ensures that different versions of the same bundle can coexist and operate independently within the OSGi framework, thereby facilitating smooth upgrades and transitions.
The Bottom Line
In the fast-paced world of Java development, embracing OSGi can transform daunting Java errors into valuable learning opportunities. By leveraging OSGi's modularity, dependency management, dynamic updates, and service-oriented architecture, developers can overcome common Java challenges and build robust, scalable applications.
So, the next time you encounter a Java error, consider it an opportunity to delve deeper into OSGi, master its capabilities, and elevate your Java development skills to new heights.
Mastering OSGi is not just about resolving errors; it's about evolving as a Java developer and harnessing the power of modularity and dynamicity to create resilient and adaptable applications.
Let OSGi be your gateway to turning Java errors into learning opportunities, and let your journey as a Java developer be enriched with the invaluable lessons it offers.
Start your OSGi journey today and discover the boundless possibilities it brings to the world of Java development.
To further enhance your OSGi skills and delve deeper into its intricacies, check out the official OSGi Alliance website for comprehensive resources and documentation.
Happy OSGi coding!