Boost Your Team's Performance with These Key Metrics!
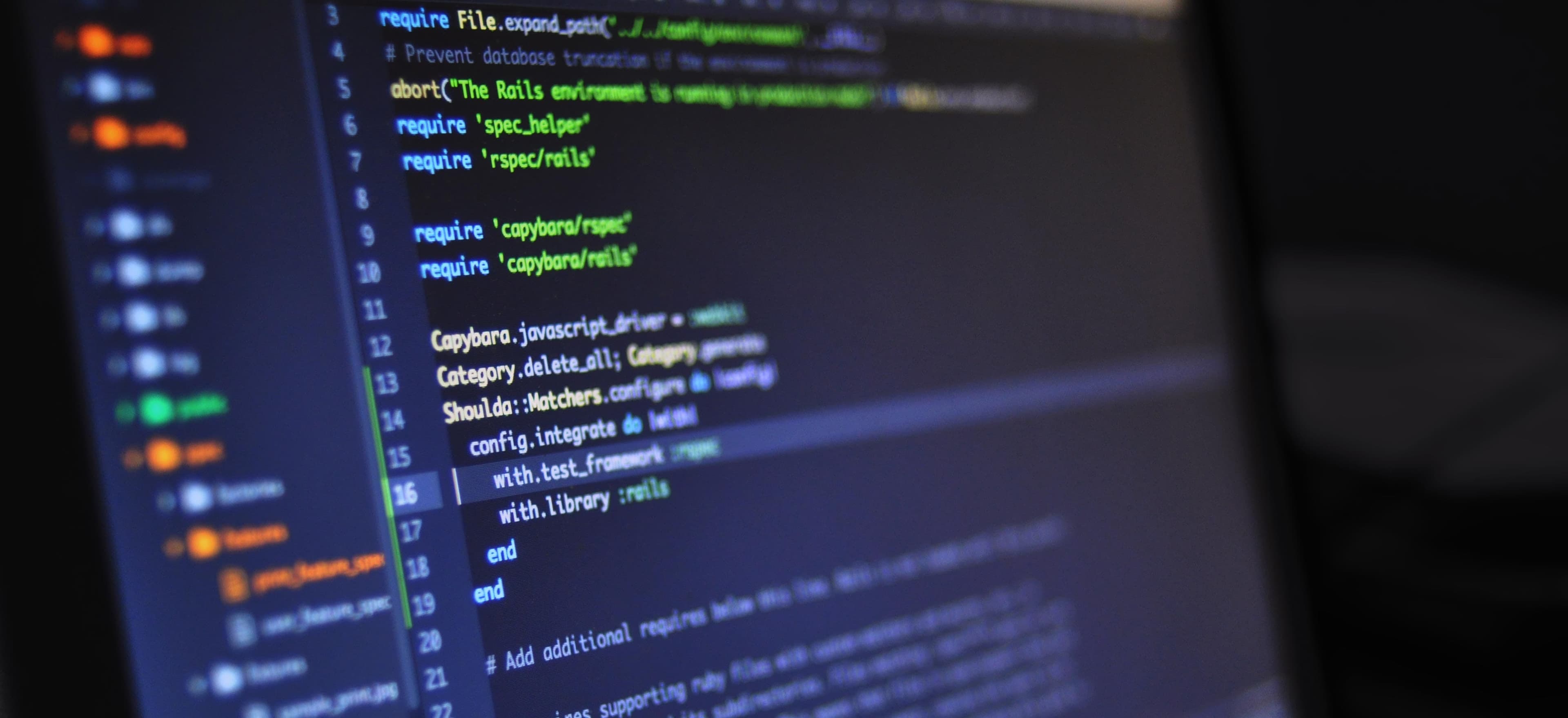
- Published on
Boost Your Team's Performance with these Key Java Metrics!
Monitoring and analyzing the performance of a Java application is crucial for ensuring its efficiency, identifying bottlene-cks, and improving user experience. In this post, we'll delve into key Java performance metrics that can aid in optimizing your application's performance. By focusing on these metrics, you can make informed decisions, identify areas for improvement, and ultimately boost your team's productivity.
Why Monitor Java Application Performance?
Java applications are widely used in enterprise environments for their robustness, scalability, and cross-platform compatibility. However, as the complexity of Java applications increases, so does the need for monitoring and optimizing their performance.
Effective monitoring allows you to:
- Identify performance bottlenecks and resource utilization issues
- Proactively address potential problems before they impact the end users
- Make data-driven decisions when optimizing code and infrastructure
- Improve the overall user experience and satisfaction
Key Performance Metrics for Java Applications
1. Memory Usage and Garbage Collection
Java applications manage memory through the Java Virtual Machine (JVM) which includes a garbage collector responsible for reclaiming memory occupied by objects that are no longer in use. Monitoring memory usage and garbage collection metrics is crucial for identifying memory leaks, inefficient memory allocation, and garbage collection pauses that can impact application performance.
Example code snippet for monitoring memory usage:
// Get the Java runtime
Runtime runtime = Runtime.getRuntime();
// Print the memory usage in MB
System.out.println("Used Memory: " + (runtime.totalMemory() - runtime.freeMemory()) / (1024 * 1024) + " MB");
System.out.println("Free Memory: " + runtime.freeMemory() / (1024 * 1024) + " MB");
System.out.println("Total Memory: " + runtime.totalMemory() / (1024 * 1024) + " MB");
System.out.println("Max Memory: " + runtime.maxMemory() / (1024 * 1024) + " MB");
2. CPU Usage
Monitoring CPU usage provides insights into the computational workload of the Java application. High CPU usage may indicate inefficient algorithms, excessive I/O operations, or resource contention. Tracking CPU usage over time can help in identifying patterns and optimizing resource-intensive tasks.
Example code snippet for monitoring CPU usage:
// Get the Java runtime
RuntimeMXBean runtimeBean = ManagementFactory.getRuntimeMXBean();
long upTime = runtimeBean.getUptime();
// Get the operating system bean
OperatingSystemMXBean osBean = ManagementFactory.getOperatingSystemMXBean();
long processCpuTime = osBean.getProcessCpuTime();
// Calculate CPU usage
double cpuUsage = (processCpuTime / 1000000.0) / upTime;
System.out.println("CPU Usage: " + cpuUsage + " %");
3. Thread Execution and Deadlocks
Threads are fundamental to concurrent programming in Java. Monitoring thread execution, deadlock occurrences, and thread contention can help in identifying concurrency issues that can impact performance and stability.
Example code snippet for detecting deadlocks:
ThreadMXBean threadBean = ManagementFactory.getThreadMXBean();
long[] deadlockedThreadIds = threadBean.findDeadlockedThreads();
if (deadlockedThreadIds != null) {
ThreadInfo[] threadInfos = threadBean.getThreadInfo(deadlockedThreadIds);
for (ThreadInfo info : threadInfos) {
System.out.println("Deadlocked Thread: " + info.getThreadName());
}
}
4. Response Time and Throughput
Measuring response time and throughput provides insights into the performance and scalability of the Java application. Response time reflects the time taken to respond to a request, while throughput represents the rate of processing requests, both of which are critical for user experience and system scalability.
Example code snippet for measuring response time:
long startTime = System.currentTimeMillis();
// Perform the operation or task
long endTime = System.currentTimeMillis();
long responseTime = endTime - startTime;
System.out.println("Response Time: " + responseTime + " ms");
5. Database Query Performance
For Java applications interacting with databases, monitoring database query performance is essential. Metrics such as query execution time, connection pooling, and cache hit ratio can provide insights into database performance and help identify optimizations.
Example code snippet for measuring database query performance:
// Execute the database query
long startTime = System.currentTimeMillis();
// Code to execute the database query
long endTime = System.currentTimeMillis();
long queryExecutionTime = endTime - startTime;
System.out.println("Query Execution Time: " + queryExecutionTime + " ms");
Using Monitoring Tools for Java Applications
While the above examples demonstrate how to programmatically capture performance metrics within the Java application code, there are also specialized monitoring tools and profilers available for in-depth performance analysis.
Tools such as JVisualVM and Java Mission Control provide comprehensive insights into Java application performance, including CPU and memory profiling, thread analysis, and garbage collection behavior.
These tools offer visual representations of performance metrics, real-time monitoring, and the ability to analyze thread dumps and heap dumps for identifying performance issues.
Closing the Chapter
Optimizing the performance of Java applications is a continuous process that requires proactive monitoring, analysis, and optimization. By focusing on key performance metrics such as memory usage, CPU usage, thread execution, response time, and database query performance, teams can gain valuable insights into their application's behavior and identify areas for improvement.
Remember, informed decisions based on accurate performance metrics can lead to significant improvements in application performance, user experience, and ultimately, the overall productivity of your development team. Start monitoring and optimizing your Java applications today to unlock their full potential!
Checkout our other articles