Mastering PL/pgSQL: Solving Common Function Pitfalls
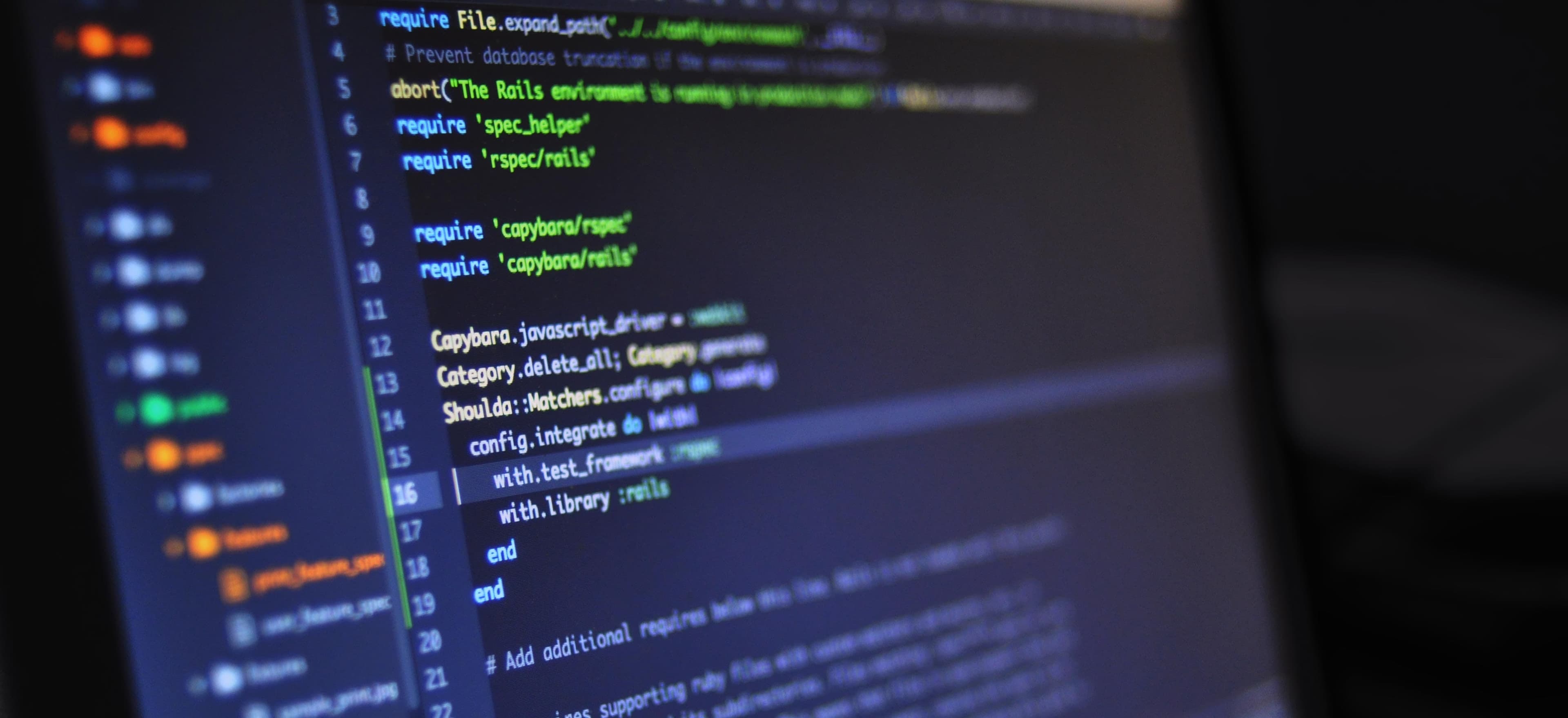
- Published on
Mastering PL/pgSQL: Dodging Common Function Pitfalls
When diving into the world of PostgreSQL and particularly its procedural language, PL/pgSQL, programmers often find themselves entangled in a web of common mistakes and pitfalls. This programming environment, while powerful and flexible, harbours its fair share of complexities that can trip up even the most experienced developers. But fear not! With the right guidance and understanding, you can navigate through these challenges and harness the full potential of PL/pgSQL in your database operations.
In this comprehensive guide, we'll shine a spotlight on the most prevalent pitfalls in PL/pgSQL function development and, crucially, how to sidestep or overcome them. By the end of this piece, you'll not only have deepened your understanding of this potent programming tool but also equipped yourself with practical strategies to avoid common mistakes, enhancing both the efficiency and robustness of your database applications.
Understanding the Basics: PL/pgSQL
Before we delve into the nitty-gritty, let’s briefly touch base on what PL/pgSQL is. PL/pgSQL stands for Procedural Language/PostgreSQL, a procedural programming language designed specifically for the PostgreSQL database system. It allows for the creation of powerful functions, procedures, and triggers to manipulate and process data directly within your database. The charm of PL/pgSQL lies in its ability to perform complex operations and logic directly on the data, minimizing the need for application-side data processing.
Common Pitfall #1: Using the Wrong Data Types
One of the most insidious mistakes in PL/pgSQL function development is the misuse of data types. Failing to properly match data types between PL/pgSQL variables and PostgreSQL columns can lead to unexpected behavior, degraded performance, or outright errors.
Example and Solution:
Consider this snippet of a function that attempts to calculate the total sales:
CREATE OR REPLACE FUNCTION calculate_total_sales()
RETURNS numeric AS $$
DECLARE
total_sales numeric;
BEGIN
SELECT SUM(amount) INTO total_sales FROM sales;
RETURN total_sales;
END;
$$ LANGUAGE plpgsql;
In this example, we’re ensuring that the total_sales
variable is declared with a numeric
data type, matching the data type of the amount
column in the sales
table. This cohesiveness ensures data type compatibility and optimal function performance.
Common Pitfall #2: Improper Error Handling
Error handling is another crucial area where many PL/pgSQL developers stumble. Overlooking error management can lead to functions that fail silently, making debugging a nightmare.
Example and Solution:
To implement robust error handling, use the EXCEPTION
block in PL/pgSQL:
CREATE OR REPLACE FUNCTION process_order(order_id bigint)
RETURNS void AS $$
BEGIN
-- Attempt to process the order
-- Code for processing the order here
EXCEPTION WHEN OTHERS THEN
-- Log the error or take corrective action
RAISE NOTICE 'An error occurred: %', SQLERRM;
-- Optionally, re-throw the error
RAISE;
END;
$$ LANGUAGE plpgsql;
By including an EXCEPTION
block, this function is now better equipped to handle unexpected errors gracefully, alerting the developer or end-user to the issue without crashing.
Common Pitfall #3: Ignoring Performance Best Practices
Performance in PL/pgSQL can easily degrade if best practices are not followed. One such practice is to avoid excessive use of PL/pgSQL for operations better handled by SQL.
Example and Solution:
For instance, consider a scenario where we need to update a large dataset:
-- Less performant approach:
DO $$
DECLARE
rec record;
BEGIN
FOR rec IN SELECT * FROM large_table LOOP
-- Imagine complex processing here
UPDATE large_table SET processed = true WHERE id = rec.id;
END LOOP;
END;
$$ LANGUAGE plpgsql;
A more performant approach would leverage a bulk operation:
-- More performant approach:
UPDATE large_table SET processed = true;
Whenever possible, leveraging SQL's set-based operations can dramatically improve the performance of your PL/pgSQL functions.
Enhancing Your PL/pgSQL Toolbox
While avoiding the aforementioned pitfalls will significantly improve the robustness and performance of your PL/pgSQL functions, the journey doesn’t end here. PL/pgSQL offers a plethora of commands, functions, and patterns that can further refine your database programming prowess. For example, familiarize yourself with cursors for advanced data retrieval, listen/notify commands for inter-process communication, and the use of dynamic SQL for flexible query execution.
Resources and Further Reading
To dive deeper into PL/pgSQL and PostgreSQL, here are two invaluable resources:
- The official PostgreSQL documentation on PL/pgSQL: PL/pgSQL - SQL Procedural Language
- A comprehensive guide to PostgreSQL performance tips: PostgreSQL Performance Tuning
By taking advantage of these resources and adhering to best practices, you can master PL/pgSQL and unlock the full potential of your PostgreSQL databases.
In Conclusion, Here is What Matters
PL/pgSQL is a powerful tool in the hands of a skilled PostgreSQL developer. By understanding and avoiding common pitfalls like improper data type usage, neglecting error handling, and overlooking performance best practices, developers can create efficient, robust, and maintainable PL/pgSQL functions. Remember, the key to mastering PL/pgSQL is a blend of understanding its core principles, continuous learning, and practical application of best practices. Equip yourself with the knowledge shared in this guide, and you’re well on your way to becoming a PL/pgSQL expert.
Checkout our other articles